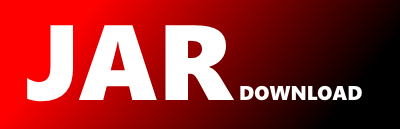
jaxx.runtime.swing.editor.EnumEditor Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%* */
package jaxx.runtime.swing.editor;
import javax.swing.JComboBox;
import java.util.EnumSet;
import java.util.Iterator;
import org.nuiton.util.ReflectUtil;
/**
* Une éditeur d'enum.
*
* @param le type d'enumeration a editer.
*
* @author chemit
*
* @since 1.6.0
*/
public class EnumEditor> extends JComboBox {
private static final long serialVersionUID = 2L;
/**
* Type of enumeration
*/
protected Class type;
/**
* Creates a {@link EnumEditor} for the given enumeration {@code type}, with
* all values of enumeration.
*
* @param type type of enumeration
* @param generci type of enumeration
* @return the instanciated editor
*/
public static > EnumEditor newEditor(Class type) {
return new EnumEditor(type);
}
/**
* Creates a {@link EnumEditor} for the given enumeration {@code type}, with
* all values of enumeration which {@code ordinal} is strictly lower than
* the given {@code maxOrdinal}.
*
* @param type type of enumeration
* @param maxOrdinal the upper (strict) bound of ordinal values allowed
* @param generic type of enumeration
* @return the instanciated editor
*/
public static > EnumEditor newEditor(Class type,
int maxOrdinal) {
return new EnumEditor(type, maxOrdinal);
}
/**
* Creates a {@link EnumEditor} for the given enumeration {@code type}, with
* all given {@code universe} values of enumeration.
*
* @param universe enumerations to put in editor
* @param generci type of enumeration
* @return the instanciated editor
*/
public static > EnumEditor newEditor(E... universe) {
return new EnumEditor(universe);
}
public EnumEditor(Class type) {
super(buildModel(type));
}
public EnumEditor(Class type, int maxOrdinal) {
super(buildModel(type, maxOrdinal));
}
public EnumEditor(E... universe) {
super(universe);
}
@Override
public E getSelectedItem() {
return (E) super.getSelectedItem();
}
protected static > Object[] buildModel(Class type) {
Class enumClass = ReflectUtil.getEnumClass(type);
EnumSet result = EnumSet.allOf(enumClass);
return result.toArray(new Object[result.size()]);
}
protected static > Object[] buildModel(Class type,
int maxOrdinal) {
Class enumClass = ReflectUtil.getEnumClass(type);
EnumSet result = EnumSet.allOf(enumClass);
Iterator itr = result.iterator();
while (itr.hasNext()) {
E e = itr.next();
if (e.ordinal() > maxOrdinal) {
itr.remove();
}
}
return result.toArray(new Object[result.size()]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy