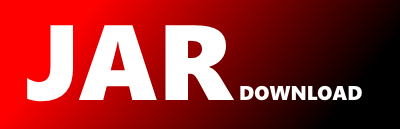
jaxx.runtime.swing.navigation.NavigationMultiTreeHandler Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.swing.navigation;
import jaxx.runtime.JAXXContext;
import jaxx.runtime.JAXXObject;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jdesktop.swingx.JXTreeTable;
import javax.swing.event.TreeSelectionEvent;
import javax.swing.tree.TreePath;
import javax.swing.tree.TreeSelectionModel;
import java.awt.Component;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
/**
* The handler of a navigation tree.
*
* This is also the selection model to use, since we must check before moving
* from nodes we can not just listen selection model changed, we must control
* it.
*
* @author sletellier
* @since 2.0.1
*/
public abstract class NavigationMultiTreeHandler extends NavigationTreeHandler {
/**
* Logger
*/
static private final Log log = LogFactory.getLog(NavigationMultiTreeHandler.class);
public NavigationMultiTreeHandler(String contextPrefix, JAXXObject context, Strategy strategy) {
super(contextPrefix, context, strategy);
}
@Override
public void valueChanged(TreeSelectionEvent event) {
TreePath[] paths = event.getPaths();
// TODO : verifier que la selection n'est pas la même
List nodes = new ArrayList();
if (paths == null){
selectNodeUI(new ArrayList());
return;
}
for(TreePath path : paths){
NavigationTreeNode node = (NavigationTreeNode) path.getLastPathComponent();
nodes.add(node);
if (log.isDebugEnabled()){
log.debug("Adding path : " + path);
log.debug("As node : " + node.getFullPath());
}
}
selectNodeUI(nodes);
}
/**
* Ouvre l'ui associée aux noeuds sélectionnés dans l'arbre de navigation.
*
* @param newUI l'ui associé au noeud sélectionné à ouvrir
* @param nodes les node de l'ui a ouvrir
* @throws Exception if any
*/
protected abstract void openUI(Component newUI, List nodes) throws Exception;
/**
* Instancie une nouvelle ui associé à des noeuds de l'arbre de navigation
*
* @param nodes les noeuds associés à l'ui à créer
* @return la nouvelle ui associée au noeud
* @throws Exception if any
*/
protected abstract Component createUI(List nodes) throws Exception;
/**
* @param nodes les noeuds associés à l'ui à retrouver
* @return l'ui associés au nouveau noeud sélectionné
*/
protected abstract Component getUI(List nodes);
protected void selectNodeUI(List nodes) {
log.info("select nodes " + nodes);
try {
List paths = new ArrayList();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy