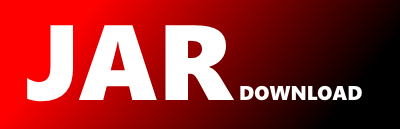
jaxx.runtime.swing.navigation.NavigationTreeContextHelper Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.swing.navigation;
import jaxx.runtime.JAXXContext;
import jaxx.runtime.JAXXUtil;
import jaxx.runtime.context.JAXXContextEntryDef;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jdesktop.swingx.JXTreeTable;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JTree;
/**
* To help getting and setting navigation tree objects from a {@link JAXXContext}.
*
* There is seven types of data which can be hold in a context :
*
* - tree : the tree
* - tree-tablr : the jx tree table
* - tree model : the navigation tree model
* - tree handler : the navigation tree handler
* - selected path : the navigation path of the selected node
* - selected node : the selected node
* - selected bean : the selected bean
*
*
* To make possible the use of more than one navigation tree system in a same
* context, we MUST distinguish the context entries definition. For this
* purpose, entries definition are normalized and prefixed by a unique {@link #prefix}.
*
* Here is the keys mapping :
*
* - tree : {@code prefix + "-tree"}
* - tree-table : {@code prefix + "-tree-table"}
* - tree model : {@code prefix + "-tree-model"}
* - tree handler : {@code prefix + "-tree-handler"}
* - selected path : {@code prefix + "-selected-path"}
* - selected node : {@code prefix + "-selected-node"}
* - selected bean : {@code prefix + "-selected-bean"}
*
*
* @author chemit
* @since 1.7.2
*/
public class NavigationTreeContextHelper {
/**
* Logger
*/
static private final Log log = LogFactory.getLog(NavigationTreeContextHelper.class);
protected final String prefix;
protected JAXXContextEntryDef> selectedPathsContextEntry;
protected JAXXContextEntryDef> selectedBeansContextEntry;
protected JAXXContextEntryDef> selectedNodesContextEntry;
protected JAXXContextEntryDef modelContextEntry;
protected JAXXContextEntryDef handlerContextEntry;
protected JAXXContextEntryDef treeContextEntry;
protected JAXXContextEntryDef treeTableContextEntry;
public NavigationTreeContextHelper(String prefix) {
this.prefix = prefix;
treeContextEntry = JAXXUtil.newContextEntryDef(prefix + "-tree", JTree.class);
treeTableContextEntry = JAXXUtil.newContextEntryDef(prefix + "-tree-table", JXTreeTable.class);
modelContextEntry = JAXXUtil.newContextEntryDef(prefix + "-model", NavigationModel.class);
handlerContextEntry = JAXXUtil.newContextEntryDef(prefix + "-handler", NavigationTreeHandler.class);
selectedBeansContextEntry = JAXXUtil.newListContextEntryDef(prefix + "-selected-beans");
selectedNodesContextEntry = JAXXUtil.newListContextEntryDef(prefix + "-selected-nodes");
selectedPathsContextEntry = JAXXUtil.newListContextEntryDef(prefix + "-selected-paths");
}
public String getPrefix() {
return prefix;
}
public JTree getTree(JAXXContext context) {
JTree r = getTreeContextEntry().getContextValue(context);
return r;
}
public JXTreeTable getTreeTable(JAXXContext context) {
JXTreeTable r = getTreeTableContextEntry().getContextValue(context);
return r;
}
public NavigationModel getModel(JAXXContext context) {
NavigationModel r = getModelContextEntry().getContextValue(context);
return r;
}
/**
* @param context where to find model
* @deprecated since 2.0 please use {@link #getModel(JAXXContext)}, will
* be remove soon
* @return the specific tree model
*/
@Deprecated
public NavigationTreeModel getTreeModel(JAXXContext context) {
NavigationModel r = getModel(context);
if (r instanceof NavigationTreeModel) {
return (NavigationTreeModel) r;
}
return null;
}
public NavigationTreeHandler getTreeHandler(JAXXContext context) {
NavigationTreeHandler r =
getTreeHandlerContextEntry().getContextValue(context);
return r;
}
public String getSelectedPath(JAXXContext context) {
String result = getSelectedValue(getSelectedPathContextEntry(),context);
return result;
}
public List getSelectedPaths(JAXXContext context) {
return getSelectedPathContextEntry().getContextValue(context);
}
public NavigationTreeNode getSelectedNode(JAXXContext context) {
NavigationTreeNode result = getSelectedValue(getSelectedNodeContextEntry(),context);
return result;
}
public List getSelectedNodes(JAXXContext context) {
return getSelectedNodeContextEntry().getContextValue(context);
}
public Object getSelectedBean(JAXXContext context) {
Object result = getSelectedValue(getSelectedBeanContextEntry(),context);
return result;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy