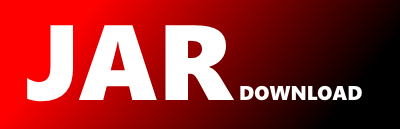
jaxx.runtime.swing.navigation.NavigationTreeHandlerWithCardLayout Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.swing.navigation;
import jaxx.runtime.JAXXContext;
import jaxx.runtime.JAXXObject;
import jaxx.runtime.swing.CardLayout2;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import javax.swing.JPanel;
import java.awt.Component;
import java.util.List;
/**
* Simple {@link NavigationTreeHandler} implementation with a {@link CardLayout2} to manage components to
* associated with tree's nodes.
*
* For each node, the ui associated has a constraints in a cardlayout which is the node context path.
*
* A single container managed by the cardlayout is used to display the components associated with tree's nodes.
*
* @author chemit
*/
public abstract class NavigationTreeHandlerWithCardLayout extends NavigationTreeHandler {
/**
* Logger
*/
static private final Log log = LogFactory.getLog(NavigationTreeHandlerWithCardLayout.class);
/**
* All components associated with a tree's node is displayed in a single container.
*
* @return the containter of components
*/
protected abstract JPanel getContentContainer();
/**
* the cardlayout managing components associated with tree node. The constraints
* of each component is the node contextPath.
*
* @return the layout used to display components associated with tree's nodes.
*/
protected abstract CardLayout2 getContentLayout();
public NavigationTreeHandlerWithCardLayout(String contextPrefix, JAXXObject context, Strategy strategy) {
super(contextPrefix, context, strategy);
if (getContentContainer() == null) {
throw new IllegalArgumentException("could not have a null 'contentContainer' in ui " + context);
}
if (getContentLayout() == null) {
throw new IllegalArgumentException("could not have a null 'contentLayout' in ui " + context);
}
}
@Override
protected Component getCurrentUI() {
CardLayout2 layout = getContentLayout();
JPanel container = getContentContainer();
return layout.getVisibleComponent(container);
}
@Override
protected Component getUI(NavigationTreeNode node) {
CardLayout2 layout = getContentLayout();
JPanel container = getContentContainer();
String constraints = strategy.getId(node);
return layout.contains(constraints) ? layout.getComponent(container, constraints) : null;
}
@Override
protected void openUI(Component newUI, NavigationTreeNode node) throws Exception {
CardLayout2 layout = getContentLayout();
JPanel container = getContentContainer();
// switch layout
String constraints = strategy.getId(node);
layout.show(container, constraints);
}
@Override
protected boolean closeUI(Component component) throws Exception {
// by default, we says that component was succesfull closed
return true;
}
@Override
protected Component createUI(NavigationTreeNode node) throws Exception {
JAXXContext uiContext = createUIContext(node);
JAXXObject newUI = node.getUIClass().getConstructor(JAXXContext.class).newInstance(uiContext);
if (log.isDebugEnabled()) {
log.debug("instanciate new ui " + newUI);
}
String constraints = strategy.getId(node);
getContentContainer().add((Component) newUI, constraints);
return (Component) newUI;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy