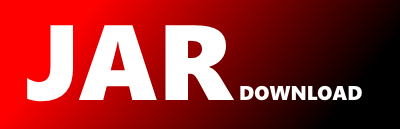
jaxx.runtime.swing.navigation.NavigationTreeTableModel Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.swing.navigation;
import jaxx.runtime.JAXXContext;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jdesktop.swingx.tree.TreeModelSupport;
import org.jdesktop.swingx.treetable.DefaultTreeTableModel;
import org.jdesktop.swingx.treetable.TreeTableNode;
import javax.swing.tree.MutableTreeNode;
import javax.swing.tree.TreeNode;
import javax.swing.tree.TreePath;
import java.util.Enumeration;
import java.util.List;
import java.util.StringTokenizer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Model of the tree table used for a navigation tree table.
*
* Il est composé de {@link NavigationTreeNode}
*
* FIXME : Essayer d'enlever les copier coller {@link NavigationTreeModel}
*
* @author sletellier
* @since 2.0.0
*/
public class NavigationTreeTableModel extends DefaultTreeTableModel implements NavigationModel {
static private final long serialVersionUID = 1L;
/**
* Logger
*/
static private final Log log = LogFactory.getLog(NavigationTreeTableModel.class);
/**
* The path separator used to build the {@link NavigationTreeNode#fullPath}.
*
* @see NavigationTreeNode#getNodePath()
* @see NavigationTreeNode#getFullPath()
*/
protected final String pathSeparator;
/**
* Context to retrieve beans
*/
private JAXXContext context;
protected List columnsName;
public NavigationTreeTableModel(String pathSeparator, JAXXContext context, List columnsName) {
super(null);
this.pathSeparator = pathSeparator;
this.context = context;
this.columnsName = columnsName;
}
/**
* @see NavigationModel#getRoot()
*/
public NavigationTreeTableNode getRoot() {
return (NavigationTreeTableNode) super.root;
}
public TreeNode[] getPathToRoot(TreeNode aNode) {
if (aNode == null){
return null;
}
return super.getPathToRoot((TreeTableNode)aNode);
}
public void setRoot(NavigationTreeTableNode root) {
this.root = root;
getModelSupport().fireNewRoot();
}
/**
* Message this to remove node from its parent. This will message
* nodesWereRemoved to create the appropriate event. This is the preferred
* way to remove a node as it handles the event creation for you.
*/
public void removeNodeFromParent(NavigationTreeNode node) {
NavigationTreeTableNode parent = (NavigationTreeTableNode)node.getParent();
if (parent == null) {
throw new IllegalArgumentException("node does not have a parent.");
}
int index = parent.getIndex(node);
node.removeFromParent();
modelSupport.fireChildRemoved(new TreePath(getPathToRoot(parent)),
index, node);
}
/**
* @see NavigationModel#findNode(String)
*/
public NavigationTreeNode findNode(String path) {
return findNode(getRoot(), path, (Pattern) null);
}
/**
* @see NavigationModel#findNode(String, String)
*/
public NavigationTreeNode findNode(String path, String regex) {
return findNode(getRoot(), path, regex);
}
/**
* @see NavigationModel#findNode(String, Pattern)
*/
public NavigationTreeNode findNode(String path, Pattern regex) {
return findNode(getRoot(), path, regex);
}
/**
* @see NavigationModel#findNode(NavigationTreeNode, String)
*/
public NavigationTreeNode findNode(NavigationTreeNode root, String path) {
return findNode(root, path, (Pattern) null);
}
/**
* @see NavigationModel#findNode(NavigationTreeNode, String, String)
*/
public NavigationTreeNode findNode(NavigationTreeNode root, String path, String regex) {
return findNode(root, path, regex == null ? null : Pattern.compile(regex));
}
/**
* @see NavigationModel#findNode(NavigationTreeNode, String, Pattern)
*/
public NavigationTreeNode findNode(NavigationTreeNode root, String path, Pattern regex) {
if (regex != null) {
Matcher matcher = regex.matcher(path);
if (!matcher.matches() || matcher.groupCount() < 1) {
log.warn("no matching regex " + regex + " to " + path);
return null;
}
path = matcher.group(1);
if (log.isDebugEnabled()) {
log.debug("matching regex " + regex + " : " + path);
}
}
StringTokenizer stk = new StringTokenizer(path, pathSeparator);
NavigationTreeNode result = root;
// pas the first token (matches the root node)
if (root.isRoot() && stk.hasMoreTokens()) {
String rootPath = stk.nextToken();
if (!rootPath.equals(root.getNodePath())) {
return null;
}
}
while (stk.hasMoreTokens()) {
result = result.getChild(stk.nextToken());
}
return result;
}
/**
* @see NavigationModel#getContext()
*/
public JAXXContext getContext() {
return context;
}
/**
* @see NavigationModel#getBean(String)
*/
public Object getBean(String navigationPath) {
Object result;
NavigationTreeNode node = findNode(navigationPath, (Pattern) null);
result = getBean(node);
return result;
}
/**
* @see NavigationModel#getBean(NavigationTreeNode)
*/
public Object getBean(NavigationTreeNode node) {
if (node == null) {
return null;
//fixme should throw a NPE exception
//throw new NullPointerException("node can not be null");
}
return node.getBean(getContext());
}
/**
* Accessor to tree model support.
*
* @return tree model support
*/
protected TreeModelSupport getModelSupport() {
return modelSupport;
}
/**
* @see NavigationModel#nodeChanged(TreeNode)
*/
public void nodeChanged(TreeNode node) {
if (node != null){
MutableTreeNode parent = (MutableTreeNode)node.getParent();
TreeNode[] treeNodes = getPathToRoot(parent);
if (treeNodes != null){
modelSupport.fireChildChanged(new TreePath(treeNodes), parent.getIndex(node), node);
} else {
log.error("[Node changed] Path to root is null !");
}
reload((NavigationTreeTableNode)node, true);
} else {
log.error("Node is null !");
}
}
/**
* @see NavigationModel#nodeStructureChanged(TreeNode)
*/
public void nodeStructureChanged(TreeNode node) {
if (node != null){
MutableTreeNode parent = (MutableTreeNode)node.getParent();
TreeNode[] treeNodes = getPathToRoot(parent);
if (treeNodes != null){
modelSupport.fireTreeStructureChanged(new TreePath(treeNodes));
} else {
log.error("[Node structure changed] Path to root is null !");
}
reload((NavigationTreeTableNode)node, true);
} else {
log.error("Node is null !");
}
}
/**
* @see NavigationModel#nodeChanged(TreeNode, boolean)
*/
public void nodeChanged(TreeNode node, boolean deep) {
if (node != null){
MutableTreeNode parent = (MutableTreeNode)node.getParent();
TreeNode[] treeNodes = getPathToRoot(parent);
if (treeNodes != null){
modelSupport.fireChildChanged(new TreePath(treeNodes), parent.getIndex(node), node);
} else {
log.error("[Node changed] Path to root is null !");
}
reload((NavigationTreeTableNode)node, deep);
} else {
log.error("Node is null !");
}
}
protected void reload(NavigationTreeTableNode node) {
reload(node, false);
}
protected void reload(NavigationTreeTableNode node, boolean deep) {
if (node == null) {
return;
}
node.reload(getContext());
if (deep) {
Enumeration> childs = node.children();
while (childs.hasMoreElements()) {
NavigationTreeTableNode o = (NavigationTreeTableNode) childs.nextElement();
reload(o, true);
}
}
}
/**
* @see NavigationModel#getPathSeparator()
*/
public String getPathSeparator() {
return pathSeparator;
}
@Override
public int getColumnCount(){
return columnsName.size();
}
@Override
public String getColumnName(int column){
return columnsName.get(column);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy