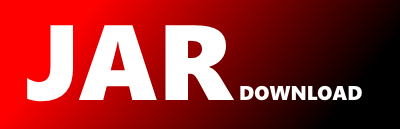
jaxx.runtime.swing.renderer.LocaleListCellRenderer Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.swing.renderer;
import java.awt.Component;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import javax.swing.DefaultListCellRenderer;
import javax.swing.Icon;
import javax.swing.JLabel;
import javax.swing.JList;
import jaxx.runtime.SwingUtil;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
*
* @author chemit
*/
public class LocaleListCellRenderer extends DefaultListCellRenderer {
public static final Log log = LogFactory.getLog(LocaleListCellRenderer.class);
private static final long serialVersionUID = 1L;
protected final Map cache = new HashMap();
protected boolean showIcon;
protected boolean showText;
public LocaleListCellRenderer() {
this(true, true);
}
public LocaleListCellRenderer(boolean showIcon, boolean showText) {
this.showIcon = showIcon;
this.showText = showText;
}
@Override
public Component getListCellRendererComponent(JList list, Object value, int index, boolean isSelected, boolean cellHasFocus) {
JLabel comp = (JLabel) super.getListCellRendererComponent(list, value, index, isSelected, cellHasFocus);
Locale locale = (Locale) value;
if (locale != null) {
Icon icon = getIcon(locale);
comp.setIcon(icon);
}
String text = getText(locale);
String tip = getToolTipText(locale);
comp.setText(text);
comp.setToolTipText(tip);
return comp;
}
public String getText(Locale locale) {
String text = null;
if (showText) {
text = getSafeText(locale);
}
return text;
}
public Icon getIcon(Locale locale) {
if (!showIcon) {
return null;
}
synchronized (cache) {
Icon icon = getSafeIcon(locale);
return icon;
}
}
public String getToolTipText(Locale locale) {
String tip = locale.getDisplayName(Locale.getDefault());
return tip;
}
public boolean isShowText() {
return showText;
}
public boolean isShowIcon() {
return showIcon;
}
public void setShowIcon(boolean showIcon) {
boolean old = this.showIcon;
this.showIcon = showIcon;
firePropertyChange("showIcon", old, showIcon);
}
public void setShowText(boolean showText) {
boolean old = this.showText;
this.showText = showText;
firePropertyChange("showText", old, showText);
}
public String getSafeText(Locale locale) {
String text = locale.getDisplayName(Locale.getDefault());
return text;
}
public synchronized Icon getSafeIcon(Locale locale) {
Icon icon = cache.get(locale);
if (icon != null) {
return icon;
}
icon = SwingUtil.getUIManagerActionIcon("i18n-" + locale.toString());
if (icon == null) {
log.warn("could not find icon action.i18n-" + locale.toString());
if (locale.getCountry() != null) {
icon = SwingUtil.getUIManagerActionIcon("i18n-" + locale.getCountry().toLowerCase());
if (icon == null) {
log.warn("could not find icon action.i18n-" + locale.getCountry().toLowerCase());
icon = SwingUtil.createActionIcon("i18n-" + locale.getCountry().toLowerCase());
if (icon == null) {
log.warn("could not find icon action.i18n-" + locale.getCountry().toLowerCase());
}
}
}
}
cache.put(locale, icon);
return icon;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy