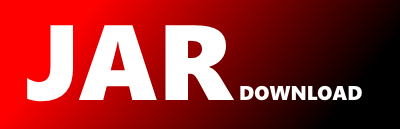
jaxx.runtime.swing.wizard.WizardOperationAction Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.swing.wizard;
import javax.swing.SwingWorker;
import jaxx.runtime.JAXXContext;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* La classe de base a implanter pour definir l'action d'une operation
* dans un wizard.
*
* @author tony
* @param le type d'étapes
* @param le type de modèle
* @since 1.3
*/
public abstract class WizardOperationAction> extends SwingWorker {
/** to use log facility, just put in your code: log.info(\"...\"); */
private static final Log log = LogFactory.getLog(WizardOperationAction.class);
E operation;
WizardOperationState operationState;
Exception error;
public WizardOperationAction(E operation) {
super();
if (!operation.isOperation()) {
throw new IllegalArgumentException("the step " + operation + " has no operation defined");
}
this.operation = operation;
}
public E getOperation() {
return operation;
}
public Exception getError() {
return error;
}
public void setError(Exception e) {
error = e;
}
public void sendMessage(String msg) {
firePropertyChange("message", null, msg);
}
public abstract void start(JAXXContext context);
public abstract void beforeAction(JAXXContext context, M model) throws Exception;
public abstract WizardOperationState doAction(M model) throws Exception;
public abstract WizardOperationState onError(M model, Exception e);
public abstract WizardOperationState onCancel(M model, Exception e);
protected abstract M getModel();
public WizardOperationState getOperationState() {
return operationState;
}
protected abstract JAXXContext getContext();
@Override
public String toString() {
return super.toString() + " < operation: " + operation + ", state: " + getState() + " >";
}
@Override
protected WizardOperationState doInBackground() throws Exception {
log.trace(this);
WizardOperationState result;
M model = getModel();
try {
beforeAction(getContext(), model);
result = doAction(model);
} catch (Exception e) {
error = e;
result = onError(model, e);
}
return result;
}
@Override
protected void done() {
log.trace(this);
WizardOperationState result = null;
try {
if (isCancelled()) {
result = onCancel(getModel(), error);
} else {
result = get();
}
} catch (Exception e) {
result = WizardOperationState.FAILED;
error = e;
// ne devrait jamais arrivé ?
log.error(e.getMessage(), e);
} finally {
// on enregistre le resultat de l'opération
this.operationState = result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy