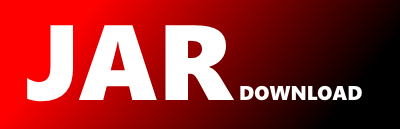
jaxx.runtime.validator.BeanValidatorField Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.validator;
import static org.nuiton.i18n.I18n._;
import javax.swing.event.EventListenerList;
import java.util.ArrayList;
import java.util.EnumMap;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.StringTokenizer;
/**
* Definition of a field to be handled in a {@link BeanValidator}.
*
* A such class is only registred in {@link BeanValidator } when the field of the bean
* was found in validator xml configuration file for a {@link com.opensymphony.xwork2.validator.FieldValidator} only.
*
* This class use properties {@link #beanClass}, {@link #name} to define
* his naturel order.
*
* @author chemit
* @param the type of the bean handled by the validator and this field of validation.
* @since 1.3
*/
public class BeanValidatorField {
/** the class of bean */
protected final Class beanClass;
/** name of field in bean */
protected final String name;
protected EnumMap> messages;
/** A list of event listeners for this validators */
protected EventListenerList listenerList = new EventListenerList();
public BeanValidatorField(Class beanClass, String name, List scopes) {
this.beanClass = beanClass;
this.name = name;
messages = new EnumMap>(BeanValidatorScope.class);
for (BeanValidatorScope scope : scopes) {
messages.put(scope, new HashSet());
}
}
public String getName() {
return name;
}
public Class getBeanClass() {
return beanClass;
}
/**
* @return true
if this field is valid (says is in error
* scope and has errors), false
otherwise.
*/
public boolean isValid() {
return !hasErrors();
}
public BeanValidatorScope getScope() {
if (hasErrors()) {
return BeanValidatorScope.ERROR;
}
if (hasWarnings()) {
return BeanValidatorScope.WARNING;
}
if (hasInfos()) {
return BeanValidatorScope.INFO;
}
return null;
}
public Set getScopes() {
return messages.keySet();
}
public boolean hasErrors() {
return hasMessages(BeanValidatorScope.ERROR);
}
public boolean hasWarnings() {
return hasMessages(BeanValidatorScope.WARNING);
}
public boolean hasInfos() {
return hasMessages(BeanValidatorScope.INFO);
}
public Set getErrors() {
return getMessages(BeanValidatorScope.ERROR);
}
public Set getWarnings() {
return getMessages(BeanValidatorScope.WARNING);
}
public Set getInfos() {
return getMessages(BeanValidatorScope.INFO);
}
public boolean hasMessages(BeanValidatorScope scope) {
return messages.containsKey(scope) && !getMessages(scope).isEmpty();
}
public Set getMessages(BeanValidatorScope scope) {
return messages.get(scope);
}
public void updateMessages(BeanValidator validator, BeanValidatorScope scope, List messages) {
if (scope == null) {
// special case to reset all messages from all scopes
for (BeanValidatorScope s : getScopes()) {
clearMessages(s, validator);
}
return;
}
if (!this.messages.containsKey(scope)) {
throw new IllegalArgumentException("the scope " + scope + " was not registred for " + this);
}
if (messages == null || messages.isEmpty()) {
// no incoming message for this scope
clearMessages(scope, validator);
return;
}
// build the diff of messages (the one to delete, the one to add)
boolean hasChanged = false;
Set currentMessages = getMessages(scope);
// detect messages to delete
Set toDelete = new java.util.HashSet(currentMessages);
toDelete.removeAll(messages);
if (!toDelete.isEmpty()) {
// apply delete
currentMessages.removeAll(toDelete);
hasChanged = true;
}
// detect messages to add
Set toAdd = new java.util.HashSet(messages);
toAdd.removeAll(currentMessages);
if (!toAdd.isEmpty()) {
// apply add
currentMessages.addAll(toAdd);
hasChanged = true;
}
if (hasChanged) {
// something has changed, fire notifications
String[] del = toDelete.toArray(new String[toDelete.size()]);
String[] add = toAdd.toArray(new String[toAdd.size()]);
validator.fireFieldChanged(this, scope, add, del);
}
toAdd.clear();
toDelete.clear();
}
public String getI18nError(String error) {
String text;
if (error.indexOf("##") == -1) {
text = _(error);
} else {
StringTokenizer stk = new StringTokenizer(error, "##");
String errorName = stk.nextToken();
List args = new ArrayList();
while (stk.hasMoreTokens()) {
args.add(stk.nextToken());
}
text = _(errorName, args.toArray());
}
return text;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof BeanValidatorField>)) {
return false;
}
BeanValidatorField> that = (BeanValidatorField>) o;
return beanClass.equals(that.beanClass) && name.equals(that.name);
}
@Override
public int hashCode() {
int result = beanClass.hashCode();
result = 31 * result + name.hashCode();
return result;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("<").append(super.toString());
sb.append(" beanClass:").append(beanClass);
sb.append(", name:").append(name);
sb.append(", scopes:").append(messages == null ? "[]" : messages.keySet());
sb.append(", scope:").append(getScope());
//sb.append(", errors:").append(errors);
sb.append('>');
return sb.toString();
}
protected void clearMessages(BeanValidatorScope scope, BeanValidator validator) {
// remove all messages
Set toDelete = getMessages(scope);
if (!toDelete.isEmpty()) {
// there is some messages to delete
String[] toDel = toDelete.toArray(new String[toDelete.size()]);
// apply deletion
toDelete.clear();
// fire
validator.fireFieldChanged(this, scope, null, toDel);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy