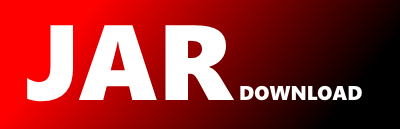
jaxx.runtime.validator.field.FieldExpressionWithParamsValidator Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.validator.field;
import com.opensymphony.xwork2.util.ValueStack;
import com.opensymphony.xwork2.validator.ValidationException;
import com.opensymphony.xwork2.validator.validators.FieldExpressionValidator;
import java.util.Map;
import java.util.StringTokenizer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.nuiton.util.ConverterUtil;
/**
* Extends {@link FieldExpressionValidator} to add some extra parameters available
* in the {@link #getExpression()}
*
* @author tony
* @since 1.3
*/
public class FieldExpressionWithParamsValidator extends FieldExpressionValidator {
protected static final Pattern EXTRA_BOOLEAN_PARAM_ENTRY_PATTERN = Pattern.compile("(\\w+)\\:(false|true)");
protected static final Pattern EXTRA_SHORT_PARAM_ENTRY_PATTERN = Pattern.compile("(\\w+)\\:(\\d+)");
protected static final Pattern EXTRA_INT_PARAM_ENTRY_PATTERN = Pattern.compile("(\\w+)\\:(\\d+)");
protected static final Pattern EXTRA_LONG_PARAM_ENTRY_PATTERN = Pattern.compile("(\\w+)\\:(\\d+)");
protected static final Pattern EXTRA_DOUBLE_PARAM_ENTRY_PATTERN = Pattern.compile("(\\w+)\\:(\\d+\\.\\d+)");
protected static final Pattern EXTRA_STRING_PARAM_ENTRY_PATTERN = Pattern.compile("(\\w+)\\:(.+)");
protected ValueStack stack;
protected String booleanParams;
protected String shortParams;
protected String intParams;
protected String longParams;
protected String doubleParams;
protected String stringParams;
protected Map booleans;
protected Map shorts;
protected Map ints;
protected Map longs;
protected Map doubles;
protected Map strings;
public String getBooleanParams() {
return booleanParams;
}
public void setBooleanParams(String booleanParams) {
this.booleanParams = booleanParams;
}
public String getDoubleParams() {
return doubleParams;
}
public void setDoubleParams(String doubleParams) {
this.doubleParams = doubleParams;
}
public String getIntParams() {
return intParams;
}
public void setIntParams(String intParams) {
this.intParams = intParams;
}
public String getLongParams() {
return longParams;
}
public void setLongParams(String longParams) {
this.longParams = longParams;
}
public String getShortParams() {
return shortParams;
}
public void setShortParams(String shortParams) {
this.shortParams = shortParams;
}
public String getStringParams() {
return stringParams;
}
public void setStringParams(String stringParams) {
this.stringParams = stringParams;
}
public Map getBooleans() {
return booleans;
}
public Map getDoubles() {
return doubles;
}
public Map getInts() {
return ints;
}
public Map getLongs() {
return longs;
}
public Map getShorts() {
return shorts;
}
public Map getStrings() {
return strings;
}
@Override
public String getValidatorType() {
return "fieldexpressionwithparams";
}
@Override
public void setValueStack(ValueStack stack) {
super.setValueStack(stack);
this.stack = stack;
}
@Override
public void validate(Object object) throws ValidationException {
booleans = initParams(Boolean.class, booleanParams, EXTRA_BOOLEAN_PARAM_ENTRY_PATTERN);
shorts = initParams(Short.class, shortParams, EXTRA_SHORT_PARAM_ENTRY_PATTERN);
ints = initParams(Integer.class, intParams, EXTRA_INT_PARAM_ENTRY_PATTERN);
longs = initParams(Long.class, longParams, EXTRA_LONG_PARAM_ENTRY_PATTERN);
doubles = initParams(Double.class, doubleParams, EXTRA_DOUBLE_PARAM_ENTRY_PATTERN);
strings = initParams(String.class, stringParams, EXTRA_STRING_PARAM_ENTRY_PATTERN);
boolean pop = false;
if (!stack.getRoot().contains(this)) {
stack.push(this);
pop = true;
}
try {
super.validate(object);
} finally {
if (pop) {
stack.pop();
}
}
}
protected Map initParams(Class klass, String extraParams, Pattern pattern) throws ValidationException {
if (extraParams == null || extraParams.isEmpty()) {
// not using
return null;
}
StringTokenizer stk = new StringTokenizer(extraParams, "|");
Map result = new java.util.TreeMap();
while (stk.hasMoreTokens()) {
String entry = stk.nextToken();
Matcher matcher = pattern.matcher(entry);
if (!matcher.matches()) {
throw new ValidationException("could not parse for extra params " + extraParams + " for type " + klass.getName());
}
String paramName = matcher.group(1);
String paramValueStr = matcher.group(2);
T paramValue = ConverterUtil.convert(klass, paramValueStr);
if (log.isDebugEnabled()) {
log.debug("detected extra param : ");
}
result.put(paramName, paramValue);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy