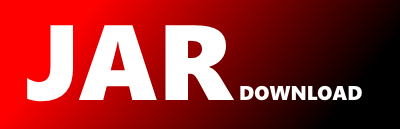
jaxx.runtime.validator.swing.SwingValidatorUtil Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.validator.swing;
import jaxx.runtime.*;
import jaxx.runtime.validator.*;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import javax.swing.JList;
import javax.swing.JTable;
import java.awt.event.MouseListener;
import javax.swing.ImageIcon;
import javax.swing.JComponent;
import javax.swing.RowSorter;
import javax.swing.SortOrder;
import static org.nuiton.i18n.I18n.n_;
/**
* The helper class for validation module.
*
* @author chemit
*/
public class SwingValidatorUtil extends BeanValidatorUtil {
static ImageIcon errorIcon;
static ImageIcon warningIcon;
static ImageIcon infoIcon;
/** to use log facility, just put in your code: log.info(\"...\"); */
static private final Log log = LogFactory.getLog(SwingValidatorUtil.class);
public static ImageIcon getErrorIcon() {
if (errorIcon == null) {
errorIcon = SwingUtil.createImageIcon("error.png");
}
return errorIcon;
}
public static ImageIcon getInfoIcon() {
if (infoIcon == null) {
infoIcon = SwingUtil.createImageIcon("info.png");
}
return infoIcon;
}
public static ImageIcon getWarningIcon() {
if (warningIcon == null) {
warningIcon = SwingUtil.createImageIcon("warning.png");
}
return warningIcon;
}
protected SwingValidatorUtil() {
// no instance
}
/**
* Prepare the ui where to display the validators messages.
*
* @param errorTable the table where to display validators messages
* @param render renderer to use
*/
public static void installUI(JTable errorTable, SwingValidatorMessageTableRenderer render) {
errorTable.setDefaultRenderer(Object.class, render);
errorTable.getRowSorter().setSortKeys(java.util.Arrays.asList(new RowSorter.SortKey(0, SortOrder.ASCENDING)));
SwingUtil.setI18nTableHeaderRenderer(errorTable,
n_("validator.scope.header"),
n_("validator.scope.header.tip"),
n_("validator.field.header"),
n_("validator.field.header.tip"),
n_("validator.message.header"),
n_("validator.message.header.tip"));
// register a single 'goto widget error' mouse listener on errorTable
registerErrorTableMouseListener(errorTable);
SwingUtil.fixTableColumnWidth(errorTable, 0, 25);
}
/**
* Register for a given validator list ui a validator mouse listener.
*
* Note: there is only one listener registred for a given list model, so
* invoking this method tiwce or more will have no effect.
*
* @param list the validation ui list
* @return the listener instanciate or found
* @see SwingValidatorMessageListMouseListener
*/
public static SwingValidatorMessageListMouseListener registerErrorListMouseListener(JList list) {
SwingValidatorMessageListMouseListener listener = getErrorListMouseListener(list);
if (listener != null) {
return listener;
}
listener = new SwingValidatorMessageListMouseListener();
if (log.isDebugEnabled()) {
log.debug(listener.toString());
}
list.addMouseListener(listener);
return listener;
}
/**
* Register for a given validator table ui a validator mouse listener
*
* Note: there is onlt one listener registred for a givne table model, so
* invokin this method twice or more will have no effect.
*
* @param table the validator table ui
* @return the listener instanciate or found
* @see SwingValidatorMessageTableMouseListener
*/
public static SwingValidatorMessageTableMouseListener registerErrorTableMouseListener(JTable table) {
SwingValidatorMessageTableMouseListener listener = getErrorTableMouseListener(table);
if (listener != null) {
return listener;
}
listener = new SwingValidatorMessageTableMouseListener();
if (log.isDebugEnabled()) {
log.debug(listener.toString());
}
table.addMouseListener(listener);
return listener;
}
/**
* @param list the validator list ui
* @return the validator list mouse listener, or null
if not found
* @see SwingValidatorMessageListMouseListener
*/
public static SwingValidatorMessageListMouseListener getErrorListMouseListener(JList list) {
if (list != null) {
for (MouseListener listener : list.getMouseListeners()) {
if (listener instanceof SwingValidatorMessageListMouseListener) {
return (SwingValidatorMessageListMouseListener) listener;
}
}
}
return null;
}
/**
* @param table the validator table ui
* @return the validator table mouse listener, or null
if not found
* @see SwingValidatorMessageTableMouseListener
*/
public static SwingValidatorMessageTableMouseListener getErrorTableMouseListener(JTable table) {
if (table != null) {
for (MouseListener listener : table.getMouseListeners()) {
if (listener instanceof SwingValidatorMessageTableMouseListener) {
return (SwingValidatorMessageTableMouseListener) listener;
}
}
}
return null;
}
public static String getMessage(SwingValidatorMessage model) {
String text = model.getMessage();
if (model.getField() != null) {
text = model.getField().getI18nError(text);
}
return text;
}
public static String getFieldName(SwingValidatorMessage model, String value) {
String text = null;
JComponent editor = model.getEditor();
if (editor != null) {
text = (String) editor.getClientProperty("validatorLabel");
/*if (l != null) {
text = I18n._(l);
} else {
// TODO should try the text
}*/
}
if (text == null) {
text = value;
}
return text;
}
public static ImageIcon getIcon(BeanValidatorScope scope) {
ImageIcon icon = null;
switch (scope) {
case ERROR:
icon = getErrorIcon();
break;
case WARNING:
icon = getWarningIcon();
break;
case INFO:
icon = getInfoIcon();
break;
}
return icon;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy