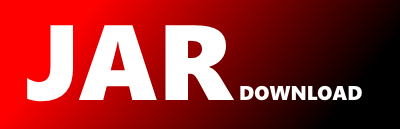
jaxx.runtime.swing.editor.ClassCellEditor Maven / Gradle / Ivy
/*
* #%L
* JAXX :: Runtime
*
* $Id: ClassCellEditor.java 2118 2010-10-26 17:44:57Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/jaxx/tags/jaxx-2.3/jaxx-runtime/src/main/java/jaxx/runtime/swing/editor/ClassCellEditor.java $
* %%
* Copyright (C) 2008 - 2010 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package jaxx.runtime.swing.editor;
import org.apache.commons.beanutils.Converter;
import org.nuiton.util.converter.ConverterUtil;
import javax.swing.DefaultCellEditor;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.event.CellEditorListener;
import javax.swing.table.TableCellEditor;
import java.awt.Component;
import java.util.EventObject;
/**
* A class cell editor (fork from comandline project).
*
* @author tchemit
*/
public class ClassCellEditor implements TableCellEditor {
protected TableCellEditor delegate;
@Override
public Component getTableCellEditorComponent(JTable table, Object value, boolean isSelected, int row, int column) {
String valStr = (value + "").trim();
if (valStr.equals("null")) {
valStr = "";
} else if (valStr.startsWith("class ")) {
valStr = valStr.substring(6);
}
Component comp;
comp = getDelegate().getTableCellEditorComponent(table, valStr, isSelected, row, column);
return comp;
}
@Override
public Object getCellEditorValue() {
Object o = !hasDelegate() ? null : delegate.getCellEditorValue();
if (o == null) {
return null;
}
Converter converter = ConverterUtil.getConverter(Class.class);
try {
if (converter != null) {
return converter.convert(Class.class, o);
}
o = Class.forName(o + "");
} catch (Exception e) {
o = null;
}
return o;
}
@Override
public boolean isCellEditable(EventObject anEvent) {
return !hasDelegate() || delegate.isCellEditable(anEvent);
}
@Override
public boolean shouldSelectCell(EventObject anEvent) {
return hasDelegate() && delegate.shouldSelectCell(anEvent);
}
@Override
public boolean stopCellEditing() {
return !hasDelegate() || delegate.stopCellEditing();
}
@Override
public void cancelCellEditing() {
if (hasDelegate()) {
delegate.cancelCellEditing();
}
}
@Override
public void addCellEditorListener(CellEditorListener l) {
if (hasDelegate()) {
delegate.addCellEditorListener(l);
}
}
@Override
public void removeCellEditorListener(CellEditorListener l) {
if (hasDelegate()) {
delegate.removeCellEditorListener(l);
}
}
protected TableCellEditor getDelegate() {
if (delegate == null) {
delegate = new DefaultCellEditor(new JTextField());
}
return delegate;
}
protected boolean hasDelegate() {
return delegate != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy