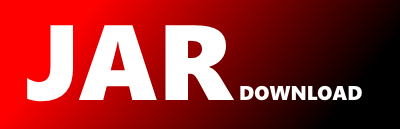
jaxx.runtime.awt.visitor.GetCompopentAtPointVisitor Maven / Gradle / Ivy
package jaxx.runtime.awt.visitor;
/*
* #%L
* JAXX :: Runtime
* $Id: GetCompopentAtPointVisitor.java 2630 2013-03-17 18:33:49Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/jaxx/tags/jaxx-2.5.19/jaxx-runtime/src/main/java/jaxx/runtime/awt/visitor/GetCompopentAtPointVisitor.java $
* %%
* Copyright (C) 2008 - 2013 CodeLutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.collect.Lists;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.awt.Component;
import java.awt.Point;
import java.util.List;
/**
* A visitor to get the deepest component given a point in a tree.
*
* To obtain the component use the method {@link #get(ComponentTreeNode, Point)}.
*
* @author tchemit
* @since 2.5.14
*/
public class GetCompopentAtPointVisitor implements ComponentTreeNodeVisitor {
/** Logger. */
private static final Log log =
LogFactory.getLog(GetCompopentAtPointVisitor.class);
int currentX;
int currentY;
final List components = Lists.newArrayList();
public static Component get(ComponentTreeNode node, Point p) {
GetCompopentAtPointVisitor v = new GetCompopentAtPointVisitor(p);
node.visit(v);
Component result = v.getLastComponent();
v.components.clear();
return result;
}
public GetCompopentAtPointVisitor(Point p) {
currentX = (int) p.getX();
currentY = (int) p.getY();
}
public Component getLastComponent() {
Component result = null;
if (!components.isEmpty()) {
ComponentTreeNode node = components.get(components.size() - 1);
result = node.getUserObject();
}
return result;
}
@Override
public void startNode(ComponentTreeNode node) {
Component component = node.getUserObject();
Point point = component.getLocation();
currentX -= point.x;
currentY -= point.y;
// check parent is ok
if (node.isRoot() || components.contains(node.getParent())) {
boolean containsPoint = component.contains(currentX, currentY);
if (containsPoint) {
if (log.isDebugEnabled()) {
log.debug("Accept component: " + component);
}
// keep this node
components.add(node);
}
}
}
@Override
public void endNode(ComponentTreeNode componentTree) {
Point point = componentTree.getUserObject().getLocation();
currentX += point.x;
currentY += point.y;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy