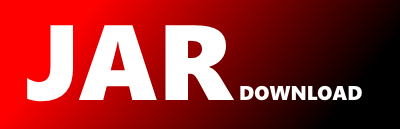
org.nuiton.jrst.JRSTUserAgent Maven / Gradle / Ivy
/*
* #%L
* JRst :: Api
* %%
* Copyright (C) 2004 - 2012 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jrst;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URL;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.xhtmlrenderer.extend.UserAgentCallback;
import org.xhtmlrenderer.layout.SharedContext;
import org.xhtmlrenderer.pdf.ITextFSImage;
import org.xhtmlrenderer.resource.CSSResource;
import org.xhtmlrenderer.resource.ImageResource;
import org.xhtmlrenderer.resource.XMLResource;
import org.xhtmlrenderer.swing.NaiveUserAgent;
import com.itextpdf.text.Image;
/**
* Class used to resolv images path for JRST documents for itext PDF generation
*
* @author jerome pages
* @since 1.6
*/
public class JRSTUserAgent implements UserAgentCallback {
/** to use log facility, just put in your code: log.info("..."); */
protected static Log log = LogFactory.getLog(JRSTUserAgent.class);
protected NaiveUserAgent delegate;
// Resources path
protected String path;
private SharedContext sharedContext;
public JRSTUserAgent(String path) {
this.path = path;
this.delegate = new NaiveUserAgent();
}
@Override
public CSSResource getCSSResource(String uri) {
return delegate.getCSSResource(uri);
}
@Override
public ImageResource getImageResource(String uri) {
uri = resolveURI(uri);
return createImageResource(uri);
}
protected ImageResource createImageResource(String uri) {
ImageResource imageResource = null;
try {
// Gets the image with its url
URL source = new URI(uri).toURL();
Image image = Image.getInstance(source);
// Sets the scale of the image
scaleToOutputResolution(image);
// Creation of the image for IText
ITextFSImage iTextFSImage = new ITextFSImage(image);
imageResource = new ImageResource(uri, iTextFSImage);
} catch (Exception eee) {
log.error("Failed to create image resource", eee);
}
return imageResource;
}
@Override
public XMLResource getXMLResource(String uri) {
return delegate.getXMLResource(uri);
}
@Override
public byte[] getBinaryResource(String uri) {
return delegate.getBinaryResource(uri);
}
@Override
public boolean isVisited(String uri) {
return delegate.isVisited(uri);
}
@Override
public void setBaseURL(String url) {
delegate.setBaseURL(url);
}
@Override
public String getBaseURL() {
return delegate.getBaseURL();
}
public String resolveURI(String uri) {
if (uri == null) return null;
String ret = null;
// If path is null, we try to set the baseURL with the uri, or if it doesn't work, with the current path
if (path == null) {
try {
URL result = new URL(uri);
setBaseURL(result.toExternalForm());
} catch (MalformedURLException e) {
try {
// Sets the current file for the base url
setBaseURL(new File(".").toURI().toURL().toExternalForm());
} catch (Exception e1) {
log.info("The default NaiveUserAgent doesn't know how to resolve the base URL for " + uri);
return null;
}
}
}
try {
// If the URI is absolute, we return the string of the url
return new URL(uri).toString();
} catch (MalformedURLException e) {
// If we are here, the uri is relative so we must build the absolute link with the path
try {
File filePath = new File(path);
URI resourcePath = filePath.toURI();
resourcePath = resourcePath.resolve(uri);
// Builds the full resource path
URL result = resourcePath.toURL();
ret = result.toURI().toString();
} catch (Exception e1) {
log.error("The default NaiveUserAgent cannot resolve the URL " + uri + " with base URL " + path, e1);
}
}
return ret;
}
private void scaleToOutputResolution(Image image) {
float factor = this.sharedContext.getDotsPerPixel();
image.scaleAbsolute(image.getPlainWidth() * factor, image.getPlainHeight() * factor);
}
public SharedContext getSharedContext() {
return this.sharedContext;
}
public void setSharedContext(SharedContext sharedContext) {
this.sharedContext = sharedContext;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy