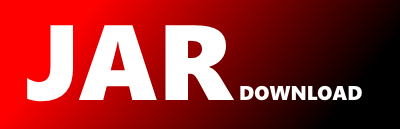
docbook.install.sh Maven / Gradle / Ivy
#!/bin/bash
# $Id: install.sh 7942 2008-03-26 06:08:08Z xmldoc $
# $Source$ #
# install.sh - Set up user environment for a XML/XSLT distribution
# This is as an interactive installer for updating your
# environment to use an XML/XSLT distribution such as the DocBook
# XSL Stylesheets. Its main purpose is to configure your
# environment with XML catalog data and schema "locating rules"
# data provided in the XML/XSLT distribution.
#
# Although this installer was created for the DocBook project, it
# is a general-purpose tool that can be used with any XML/XSLT
# distribution that provides XML/SGML catalogs and locating rules.
#
# This script is mainly intended to make things easier for you if
# you want to install a particular XML/XSLT distribution that has
# not (yet) been packaged for your OS distro (Debian, Fedora,
# whatever), or to use "snapshot" or development releases
#
# It works by updating your shell startup file (e.g., .bashrc and
# .cshrc) and .emacs file and by finding or creating a writable
# CatalogManager.properties file to update.
#
# It makes backup copies of any files it touches, and also
# generates a uninstall.sh script for reverting its changes.
#
# In the same directory where it is located, it expects to find
# the following four files:
# - locatingrules.xml
# - catalog.xml
# - catalog
# - .urilist
# And if it's unable to locate a CatalogManager.properties file in
# your environment, it expects to find an "example" one in the
# same directory as itself, which it copies over to your
# ~/.resolver directory.
#
# If the distribution contains any executables, change the value
# of the thisBinDir to a colon-separated list of the pathnames of
# the directories that contain those executables.
# mydir is the "canonical" absolute pathname for install.sh
mydir=$(cd -P $(dirname $0) && pwd -P) || exit 1
thisLocatingRules=$mydir/locatingrules.xml
thisXmlCatalog=$mydir/catalog.xml
thisSgmlCatalog=$mydir/catalog
# .urilist file contains a list of pairs of local pathnames and
# URIs to test for catalog resolution
thisUriList=$mydir/.urilist
exampleCatalogManager=$mydir/.CatalogManager.properties.example
thisCatalogManager=$HOME/.resolver/CatalogManager.properties
# thisBinDir directory is a colon-separated list of the pathnames
# to all directories that contain executables provided with the
# distribution (for example, the DocBook XSL Stylesheets
# distribution contains a "docbook-xsl-update" convenience script
# for rsync'ing up to the latest docbook-xsl snapshot). The
# install.sh script adds the value of thisBinDir to your PATH
# environment variable
thisBinDir=$mydir/tools/bin
emit_message() {
echo "$1" 1>&2
}
if [ ! "${*#--batch}" = "$*" ]; then
batchmode="Yes";
else
batchmode="No";
emit_message
if [ ! "$1" = "--test" ]; then
emit_message "NOTE: For non-interactive installs/uninstalls, use --batch"
if [ ! "$1" = "--uninstall" ]; then
emit_message
fi
fi
fi
osName="Unidentified"
if uname -s | grep -qi "cygwin"; then
osName="Cygwin"
fi
classPathSeparator=":"
if [ "$osName" = "Cygwin" ]; then
thisJavaXmlCatalog=$(cygpath -m $thisXmlCatalog)
classPathSeparator=";"
else
thisJavaXmlCatalog=$thisXmlCatalog
fi
main() {
removeOldFiles
checkRoot
updateCatalogManager
checkForResolver
writeDotFiles
updateUserStartupFiles
updateUserDotEmacs
writeUninstallFile
writeTestFile
printExitMessage
}
removeOldFiles() {
rm -f $mydir/.profile.incl
rm -f $mydir/.cshrc.incl
rm -f $mydir/.emacs.el
}
checkRoot() {
if [ $(id -u) == "0" ]; then
cat 1>&2 <&2 <&2 <&2 <&2 < $myCatalogManager || exit 1
emit_message "NOTE: Successfully updated the following file:"
emit_message " $myCatalogManager"
emit_message " Backup written to:"
emit_message " $catalogBackup"
fi
else
mv $myCatalogManager $catalogBackup || exit 1
cp $catalogBackup $myCatalogManager
echo "catalogs=$thisJavaXmlCatalog;$etcXmlCatalog" \
| sed 's/;\+/;/' | sed 's/;$//' >> $myCatalogManager || exit 1
emit_message "NOTE: \"catalogs=\" line added to $myCatalogManager."
emit_message " Backup written to $catalogBackup"
fi
;;
esac
# end of backing up and updating CatalogManager.properties
fi
fi
# end of CatalogManager.properties updates
if [ "$osName" = "Cygwin" ]; then
myCatalogManager=$(cygpath -m $myCatalogManager)
fi
return 0
}
writeDotFiles() {
while read; do
echo "$REPLY" >> $mydir/.profile.incl
done <> $mydir/.cshrc.incl
done <> $mydir/.profile.incl
done <> $mydir/.cshrc.incl
done <> $mydir/.emacs.el
done <&2 <&2 <> $HOME/$file || exit 1
cat 1>&2 <&2 <&2 <&2 <&2 <&2 <> $myEmacsFile || exit 1
cat 1>&2 <&2 <&2 <&2 < $myCatalogManager || exit 1
cat 1>&2 <&2 <&2 <&2 <&2 <&2 < $uninstallFile || exit 1
echo 'mydir=$(cd -P $(dirname $0) && pwd -P)' >> $uninstallFile || exit 1
echo "\$mydir/install.sh \\" >> $uninstallFile || exit 1
echo " --uninstall \\" >> $uninstallFile || exit 1
echo " --catalogManager=$myCatalogManager \\" >> $uninstallFile || exit 1
echo " --dotEmacs='$myEmacsFile' \\" >> $uninstallFile || exit 1
echo ' $@' >> $uninstallFile || exit 1
chmod 755 $uninstallFile || exit 1
}
writeTestFile() {
testFile=$mydir/test.sh
echo "#!/bin/bash" > $testFile || exit 1
echo 'mydir=$(cd -P $(dirname $0) && pwd -P)' >> $testFile || exit 1
echo '$mydir/install.sh --test' >> $testFile || exit 1
chmod 755 $testFile || exit 1
}
printExitMessage() {
cat 1>&2 <&2 <&2 <&2 <&2 <
#
# Permission is hereby granted, free of charge, to any person
# obtaining a copy of this software and associated documentation
# files (the "Software"), to deal in the Software without
# restriction, including without limitation the rights to use, copy,
# modify, merge, publish, distribute, sublicense, and/or sell copies
# of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be
# included in all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
# HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
# WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
# vim: number
© 2015 - 2025 Weber Informatics LLC | Privacy Policy