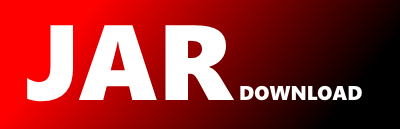
org.nuiton.math.matrix.viewer.renderer.MatrixPanelRenderer Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Nuiton Matrix :: GUI
* %%
* Copyright (C) 2010 - 2023 Codelutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.math.matrix.viewer.renderer;
import static org.nuiton.i18n.I18n.t;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
import javax.swing.Icon;
import javax.swing.JButton;
import javax.swing.JPanel;
import jaxx.runtime.FileChooserUtil;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.math.matrix.MatrixND;
import org.nuiton.math.matrix.gui.MatrixPanelEditor;
import org.nuiton.math.matrix.viewer.MatrixRenderer;
import org.nuiton.util.FileUtil;
import org.nuiton.util.Resource;
/**
* Matrix panel renderer.
*
* @author chatellier
* @version $Revision$
*
* Last update : $Date$
* By : $Author$
*/
public class MatrixPanelRenderer implements ActionListener, MatrixRenderer {
/** Class logger. */
private static Log log = LogFactory.getLog(MatrixPanelRenderer.class);
/** Renderer main component. */
protected JPanel panel;
/** Matrix editor. */
protected MatrixPanelEditor editor;
/** Export button. */
protected JButton exportButton;
/** Current matrix. */
protected MatrixND matrix;
public MatrixPanelRenderer() {
panel = new JPanel(new BorderLayout());
// main component
editor = new MatrixPanelEditor();
panel.add(editor, BorderLayout.CENTER);
// export button
exportButton = new JButton(t("nuitonmatrix.viewer.renderer.exportascsv"));
exportButton.addActionListener(this);
exportButton.setActionCommand("exportascsv");
exportButton.setEnabled(false);
panel.add(exportButton, BorderLayout.SOUTH);
}
/**
* Get editor instance to allow configuration.
*
* @return internal editor reference
*/
public MatrixPanelEditor getEditor() {
return editor;
}
@Override
public Component getComponent(MatrixND matrix) {
this.matrix = matrix;
editor.setMatrix(matrix);
exportButton.setEnabled(matrix != null);
return panel;
}
@Override
public Icon getIcon() {
return Resource.getIcon("/icons/fatcow/table.png");
}
@Override
public String getName() {
return t("nuitonmatrix.viewer.renderer.panel");
}
@Override
public void actionPerformed(ActionEvent e) {
if ("exportascsv".equals(e.getActionCommand())) {
Writer writer = null;
try {
File file = FileChooserUtil.getFile(".+\\.csv", "CSV file");
if (file != null) {
// add csv extension
if (!file.getName().endsWith(".csv")) {
file = new File(file.getAbsolutePath() + ".csv");
}
writer = new OutputStreamWriter(new FileOutputStream(file), StandardCharsets.UTF_8);
matrix.exportCSV(writer, true);
}
} catch (IOException eee) {
if (log.isErrorEnabled()) {
log.error("Error during export", eee);
}
}
finally {
if (writer != null) {
try {
writer.close();
} catch (IOException ex) {
if (log.isErrorEnabled()) {
log.error("Can't close writer", ex);
}
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy