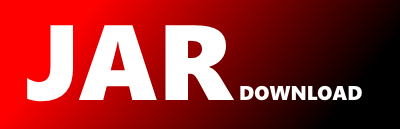
org.nuiton.math.matrix.DoubleBigVector Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Nuiton Matrix :: API
* %%
* Copyright (C) 2004 - 2010 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.math.matrix;
import java.util.Arrays;
/**
* DoubleBigVector.
*
* Utilise un tableau en mémoire, qui ne supporte pas plus de Integer.MAX_VALUE
* Donc lors de la création si la capacité demandée est supérieur une exception est levée.
*
* Created: 6 octobre 2005 02:54:36 CEST
*
* @author Benjamin POUSSIN <[email protected]>
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class DoubleBigVector implements Vector { // DoubleBigVector
protected double[] data = null;
public DoubleBigVector() {
}
public DoubleBigVector(long capacity) {
init(capacity);
}
@Override
public void init(long capacity) {
if (capacity > Integer.MAX_VALUE) {
throw new IllegalArgumentException(String.format("Dense vector max capacity is '%s' asked '%s'", Integer.MAX_VALUE, capacity));
}
if (data == null) {
try {
data = new double[(int)capacity];
} catch (OutOfMemoryError eee) {
throw new MatrixException("Can't create vector with capacity = " + capacity, eee);
}
}
}
@Override
public String getInfo() {
return "Double vector dense: " + size();
}
@Override
public long getNumberOfAssignedValue() {
return size();
}
@Override
public long size() {
return data.length;
}
@Deprecated
@Override
public double getMaxOccurence() {
return getMaxOccurrence();
}
@Override
public double getMaxOccurrence() {
return MatrixHelper.maxOccurrence(data);
}
@Override
public double getValue(long pos) {
return data[(int) pos];
}
@Override
public void setValue(long pos, double value) {
data[(int) pos] = value;
}
@Override
public boolean equals(Object o) {
boolean result = false;
if (o instanceof DoubleBigVector) {
DoubleBigVector other = (DoubleBigVector) o;
result = Arrays.equals(this.data, other.data);
} else if (o instanceof Vector) {
Vector other = (Vector) o;
result = size() == other.size();
for (int i = 0; i < size() && result; i++) {
result = getValue(i) == other.getValue(i);
}
}
return result;
}
/**
* Seule la longueur du tableau est constante
*/
@Override
public int hashCode() {
return Long.hashCode(size());
}
@Override
public boolean isImplementedPaste(Vector v) {
return v instanceof DoubleBigVector;
}
@Override
public boolean isImplementedMap() {
return true;
}
@Override
public void paste(Vector src) {
DoubleBigVector fbv = (DoubleBigVector) src;
System.arraycopy(fbv.data, 0, this.data, 0, (int)this.size());
}
@Override
public void map(MapFunction f) {
for (int i = 0; i < data.length; i++) {
data[i] = f.apply(data[i]);
}
}
@Override
public VectorIterator iterator() {
return new VectorIteratorImpl(this);
}
@Override
public VectorIterator iteratorNotZero() {
return new VectorIteratorImpl(this, 0);
}
} // DoubleBigVector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy