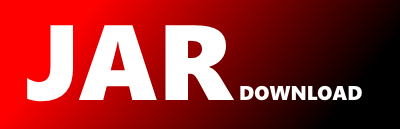
org.nuiton.topia.it.legacy.TopiaItLegacyEntityEnum Maven / Gradle / Ivy
package org.nuiton.topia.it.legacy;
import com.google.common.base.Predicate;
import com.google.common.collect.Iterables;
import com.google.common.collect.Sets;
import java.lang.reflect.Array;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import javax.annotation.Generated;
import org.apache.commons.lang3.ArrayUtils;
import org.nuiton.topia.it.legacy.test.ano1882.FrenchCompany;
import org.nuiton.topia.it.legacy.test.ano1882.SIREN;
import org.nuiton.topia.it.legacy.test.ano1882.SIRET;
import org.nuiton.topia.it.legacy.test.entities.Person;
import org.nuiton.topia.it.legacy.test.entities.Pet;
import org.nuiton.topia.it.legacy.test.entities.Race;
import org.nuiton.topia.it.legacy.topiatest.Address;
import org.nuiton.topia.it.legacy.topiatest.Bill;
import org.nuiton.topia.it.legacy.topiatest.Company;
import org.nuiton.topia.it.legacy.topiatest.Department;
import org.nuiton.topia.it.legacy.topiatest.Employe;
import org.nuiton.topia.it.legacy.topiatest.GeneralizedNaturalizedEntity;
import org.nuiton.topia.it.legacy.topiatest.NaturalizedEntity;
import org.nuiton.topia.it.legacy.topiatest.Personne;
import org.nuiton.topia.it.legacy.topiatest.Product;
import org.nuiton.topia.it.legacy.topiatest.QueriedEntity;
import org.nuiton.topia.it.legacy.topiatest.Store;
import org.nuiton.topia.it.legacy.topiatest.Type;
import org.nuiton.topia.it.legacy.topiatest.deletetest.Contact2;
import org.nuiton.topia.it.legacy.topiatest.deletetest.Party2;
import org.nuiton.topia.it.legacy.topiatest.deletetest.Telephone2;
import org.nuiton.topia.persistence.TopiaEntity;
import org.nuiton.topia.persistence.TopiaEntityEnum;
import org.nuiton.topia.persistence.TopiaException;
import org.nuiton.topia.persistence.util.EntityOperator;
import org.nuiton.topia.persistence.util.EntityOperatorStore;
import org.nuiton.topia.persistence.util.TopiaEntityHelper;
@Generated(value = "org.nuiton.topia.templates.EntityEnumTransformer", date = "Thu Oct 13 17:59:13 CEST 2016")
public enum TopiaItLegacyEntityEnum implements TopiaEntityEnum {
FrenchCompany(FrenchCompany.class, null, "frenchcompany", ArrayUtils.EMPTY_STRING_ARRAY),
SIREN(SIREN.class, null, "siren", ArrayUtils.EMPTY_STRING_ARRAY),
SIRET(SIRET.class, null, "siret", ArrayUtils.EMPTY_STRING_ARRAY),
Person(Person.class, null, "person", ArrayUtils.EMPTY_STRING_ARRAY),
Pet(Pet.class, null, "pet", ArrayUtils.EMPTY_STRING_ARRAY),
Race(Race.class, null, "race", ArrayUtils.EMPTY_STRING_ARRAY),
Address(Address.class, null, "address", ArrayUtils.EMPTY_STRING_ARRAY),
Bill(Bill.class, null, "bill", new String[]{"store", "company"}),
Company(Company.class, null, "company", ArrayUtils.EMPTY_STRING_ARRAY),
Department(Department.class, null, "department", ArrayUtils.EMPTY_STRING_ARRAY),
Employe(Employe.class, null, "employe", ArrayUtils.EMPTY_STRING_ARRAY),
GeneralizedNaturalizedEntity(GeneralizedNaturalizedEntity.class, null, "generalizednaturalizedentity", new String[]{"naturalIdNotNull"}, "naturalIdNotNull", "naturalIdNull"),
NaturalizedEntity(NaturalizedEntity.class, null, "naturalizedentity", new String[]{"naturalIdNotNull"}, "naturalIdNotNull", "naturalIdNull"),
Personne(Personne.class, null, "personne", ArrayUtils.EMPTY_STRING_ARRAY),
Product(Product.class, null, "product", ArrayUtils.EMPTY_STRING_ARRAY),
QueriedEntity(QueriedEntity.class, null, "queriedentity", ArrayUtils.EMPTY_STRING_ARRAY),
Store(Store.class, null, "store", ArrayUtils.EMPTY_STRING_ARRAY),
Type(Type.class, null, "type", ArrayUtils.EMPTY_STRING_ARRAY),
Contact2(Contact2.class, null, "contact2", ArrayUtils.EMPTY_STRING_ARRAY),
Party2(Party2.class, null, "party2", ArrayUtils.EMPTY_STRING_ARRAY),
Telephone2(Telephone2.class, null, "telephone2", ArrayUtils.EMPTY_STRING_ARRAY);
/**
* The contract of the entity.
*/
private final Class extends TopiaEntity> contract;
/**
* The optional name of database schema of the entity (if none was filled, will be {@code null}).
*/
private final String dbSchemaName;
/**
* The name of the database table for the entity.
*/
private final String dbTableName;
/**
* The fully qualified name of the implementation of the entity.
*/
private String implementationFQN;
/**
* The implementation class of the entity (will be lazy computed at runtime).
*/
private Class extends TopiaEntity> implementation;
/**
* The array of property involved in the natural key of the entity.
*/
private final String[] naturalIds;
/**
* The array of not null properties of the entity.
*/
private final String[] notNulls;
TopiaItLegacyEntityEnum(Class extends TopiaEntity> contract, String dbSchemaName, String dbTableName, String[] notNulls, String ... naturalIds) {
this.contract = contract;
this.dbSchemaName = dbSchemaName;
this.dbTableName = dbTableName;
this.notNulls = Arrays.copyOf(notNulls, notNulls.length);
this.naturalIds = naturalIds;
implementationFQN = contract.getName() + "Impl";
}
@Override
public Class extends TopiaEntity> getContract() {
return contract;
}
@Override
public String dbSchemaName() {
return dbSchemaName;
}
@Override
public String dbTableName() {
return dbTableName;
}
@Override
public String[] getNaturalIds() {
return naturalIds;
}
@Override
public boolean isUseNaturalIds() {
return naturalIds.length > 0;
}
@Override
public String[] getNotNulls() {
return notNulls;
}
@Override
public boolean isUseNotNulls() {
return notNulls.length > 0;
}
@Override
public String getImplementationFQN() {
return implementationFQN;
}
@Override
public void setImplementationFQN(String implementationFQN) {
this.implementationFQN = implementationFQN;
implementation = null;
// reinit the operators store
EntityOperatorStore.clear();
}
@Override
public boolean accept(Class extends TopiaEntity> klass) {
TopiaItLegacyEntityEnum constant = valueOf(klass);
boolean result = constant.getContract() == contract;
return result;
}
@Override
public Class extends TopiaEntity> getImplementation() {
if (implementation == null) {
try {
implementation = (Class extends TopiaEntity>) Class.forName(implementationFQN);
} catch (ClassNotFoundException e) {
throw new TopiaException("could not find class " + implementationFQN, e);
}
}
return implementation;
}
public static TopiaItLegacyEntityEnum valueOf(TopiaEntity entity) {
return valueOf(entity.getClass());
}
public static TopiaItLegacyEntityEnum valueOf(final Class> klass) {
if (klass.isInterface()) {
return valueOf(klass.getSimpleName());
}
Class> contractClass = TopiaEntityHelper.getContractClass(TopiaItLegacyEntityEnum.values(), (Class) klass);
if (contractClass != null) {
return valueOf(contractClass.getSimpleName());
}
throw new IllegalArgumentException("no entity defined for the class " + klass + " in : " + Arrays.toString(TopiaItLegacyEntityEnum.values()));
}
public static TopiaItLegacyEntityEnum[] getContracts() {
TopiaItLegacyEntityEnum[] result = values();
return result;
}
public static Class getContractClass(Class klass) {
TopiaItLegacyEntityEnum constant = valueOf(klass);
Class result = (Class) constant.getContract();
return result;
}
public static Class extends TopiaEntity>[] getContractClasses() {
TopiaItLegacyEntityEnum[] values = values();
Class extends TopiaEntity>[] result = (Class extends TopiaEntity>[]) Array.newInstance(Class.class, values.length);
for (int i = 0; i < values.length; i++) {
result[i] = values[i].getContract();
}
return result;
}
public static Class getImplementationClass(Class klass) {
TopiaItLegacyEntityEnum constant = valueOf(klass);
Class result = (Class) constant.getImplementation();
return result;
}
public static Set> getImplementationClasses() {
TopiaItLegacyEntityEnum[] values = values();
Set> result = new LinkedHashSet>();
for (int i = 0; i < values.length; i++) {
result.add(values[i].getImplementation());
}
return result;
}
public static String getImplementationClassesAsString() {
StringBuilder buffer = new StringBuilder();
for (Class extends TopiaEntity> aClass : getImplementationClasses()) {
buffer.append(',').append(aClass.getName());
}
String result = buffer.substring(1);
return result;
}
public static EntityOperator getOperator(Class klass) {
TopiaItLegacyEntityEnum constant = valueOf(klass);
EntityOperator result = EntityOperatorStore.getOperator(constant);
return result;
}
} //TopiaItLegacyEntityEnum
© 2015 - 2024 Weber Informatics LLC | Privacy Policy