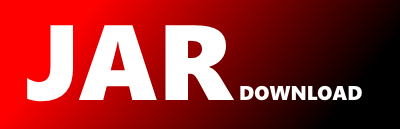
org.nuiton.topia.it.legacy.topiatest.CompanyAbstract Maven / Gradle / Ivy
package org.nuiton.topia.it.legacy.topiatest;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import javax.annotation.Generated;
import org.nuiton.i18n.I18n;
import org.nuiton.topia.it.legacy.AbstractTopiaItLegacyEntity;
import org.nuiton.topia.persistence.TopiaEntity;
import org.nuiton.topia.persistence.TopiaEntityVisitor;
import org.nuiton.topia.persistence.TopiaException;
import org.nuiton.topia.persistence.util.TopiaEntityHelper;
@Generated(value = "org.nuiton.topia.templates.EntityTransformer", date = "Thu Oct 13 17:59:13 CEST 2016")
public abstract class CompanyAbstract extends AbstractTopiaItLegacyEntity implements Company {
/**
* Nom de l'attribut en BD : name
*/
protected String name;
/**
* Nom de l'attribut en BD : siret
*/
protected int siret;
/**
* Nom de l'attribut en BD : employe
*/
protected List employe;
/**
* Nom de l'attribut en BD : store
*/
protected Collection storeBill;
/**
* Nom de l'attribut en BD : department
*/
protected Collection department;
private static final long serialVersionUID = 4121691295267055154L;
static {
I18n.n("topia.test.common.company");
I18n.n("topia.test.common.name");
I18n.n("topia.test.common.siret");
I18n.n("topia.test.common.employe");
I18n.n("topia.test.common.store");
I18n.n("topia.test.common.department");
}
@Override
public void accept(TopiaEntityVisitor visitor) throws TopiaException {
visitor.start(this);
accept0(visitor);
visitor.end(this);
}
protected void accept0(TopiaEntityVisitor visitor) throws TopiaException {
visitor.visit(this, PROPERTY_NAME, String.class, name);
visitor.visit(this, PROPERTY_SIRET, int.class, siret);
visitor.visit(this, PROPERTY_EMPLOYE, List.class, Employe.class, employe);
visitor.visit(this, PROPERTY_STORE_BILL, Collection.class, Bill.class, storeBill);
visitor.visit(this, PROPERTY_DEPARTMENT, Collection.class, Department.class, department);
}
@Override
public void setName(String name) {
String oldValue = this.name;
fireOnPreWrite(PROPERTY_NAME, oldValue, name);
this.name = name;
fireOnPostWrite(PROPERTY_NAME, oldValue, name);
}
@Override
public String getName() {
fireOnPreRead(PROPERTY_NAME, name);
String result = this.name;
fireOnPostRead(PROPERTY_NAME, name);
return result;
}
@Override
public void setSiret(int siret) {
int oldValue = this.siret;
fireOnPreWrite(PROPERTY_SIRET, oldValue, siret);
this.siret = siret;
fireOnPostWrite(PROPERTY_SIRET, oldValue, siret);
}
@Override
public int getSiret() {
fireOnPreRead(PROPERTY_SIRET, siret);
int result = this.siret;
fireOnPostRead(PROPERTY_SIRET, siret);
return result;
}
@Override
public void addEmploye(Employe employe) {
fireOnPreWrite(PROPERTY_EMPLOYE, null, employe);
if (this.employe == null) {
this.employe = new LinkedList();
}
this.employe.add(employe);
fireOnPostWrite(PROPERTY_EMPLOYE, this.employe.size(), null, employe);
}
@Override
public void addEmploye(int index, Employe employe) {
fireOnPreWrite(PROPERTY_EMPLOYE, null, employe);
if (this.employe == null) {
this.employe = new LinkedList();
}
this.employe.add(index, employe);
fireOnPostWrite(PROPERTY_EMPLOYE, index, null, employe);
}
@Override
public void addAllEmploye(Iterable employe) {
if (employe == null) {
return;
}
for (Employe item : employe) {
addEmploye(item);
}
}
@Override
public void setEmploye(List employe) {
// Copy elements to keep data for fire with new reference
List oldValue = this.employe != null ? new LinkedList(this.employe) : null;
fireOnPreWrite(PROPERTY_EMPLOYE, oldValue, employe);
this.employe = employe;
fireOnPostWrite(PROPERTY_EMPLOYE, oldValue, employe);
}
@Override
public void removeEmploye(Employe employe) {
fireOnPreWrite(PROPERTY_EMPLOYE, employe, null);
if (this.employe == null || !this.employe.remove(employe)) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_EMPLOYE, this.employe.size() + 1, employe, null);
}
@Override
public void removeEmploye(int index) {
fireOnPreWrite(PROPERTY_EMPLOYE, employe, null);
if (this.employe == null) {
throw new IllegalArgumentException("List does not contain given element");
}
Employe oldValue = this.employe.remove(index);
if (oldValue == null) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_EMPLOYE, index, oldValue, null);
}
@Override
public void clearEmploye() {
if (this.employe == null) {
return;
}
List oldValue = new LinkedList(this.employe);
fireOnPreWrite(PROPERTY_EMPLOYE, oldValue, this.employe);
this.employe.clear();
fireOnPostWrite(PROPERTY_EMPLOYE, oldValue, this.employe);
}
@Override
public List getEmploye() {
return employe;
}
@Override
public Employe getEmploye(int index) {
return TopiaEntityHelper.getEntityByIndex(employe, index);
}
@Override
public Employe getEmployeByTopiaId(String topiaId) {
return TopiaEntityHelper.getEntityByTopiaId(employe, topiaId);
}
@Override
public List getEmployeTopiaIds() {
List topiaIds = new LinkedList();
List tmp = getEmploye();
if (tmp != null) {
for (TopiaEntity topiaEntity : tmp) {
topiaIds.add(topiaEntity.getTopiaId());
}
}
return topiaIds;
}
@Override
public int sizeEmploye() {
if (employe == null) {
return 0;
}
return employe.size();
}
@Override
public boolean isEmployeEmpty() {
int size = sizeEmploye();
return size == 0;
}
@Override
public boolean isEmployeNotEmpty() {
boolean empty = isEmployeEmpty();
return ! empty;
}
@Override
public boolean containsEmploye(Employe employe) {
boolean contains = this.employe !=null && this.employe.contains(employe);
return contains;
}
@Override
public void addStoreBill(Bill storeBill) {
fireOnPreWrite(PROPERTY_STORE_BILL, null, storeBill);
if (this.storeBill == null) {
this.storeBill = new LinkedList();
}
this.storeBill.add(storeBill);
fireOnPostWrite(PROPERTY_STORE_BILL, this.storeBill.size(), null, storeBill);
}
@Override
public void addAllStoreBill(Iterable storeBill) {
if (storeBill == null) {
return;
}
for (Bill item : storeBill) {
addStoreBill(item);
}
}
@Override
public void setStoreBill(Collection storeBill) {
// Copy elements to keep data for fire with new reference
Collection oldValue = this.storeBill != null ? new LinkedList(this.storeBill) : null;
fireOnPreWrite(PROPERTY_STORE_BILL, oldValue, storeBill);
this.storeBill = storeBill;
fireOnPostWrite(PROPERTY_STORE_BILL, oldValue, storeBill);
}
@Override
public void removeStoreBill(Bill storeBill) {
fireOnPreWrite(PROPERTY_STORE_BILL, storeBill, null);
if (this.storeBill == null || !this.storeBill.remove(storeBill)) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_STORE_BILL, this.storeBill.size() + 1, storeBill, null);
}
@Override
public void clearStoreBill() {
if (this.storeBill == null) {
return;
}
Collection oldValue = new LinkedList(this.storeBill);
fireOnPreWrite(PROPERTY_STORE_BILL, oldValue, this.storeBill);
this.storeBill.clear();
fireOnPostWrite(PROPERTY_STORE_BILL, oldValue, this.storeBill);
}
@Override
public Collection getStoreBill() {
return storeBill;
}
@Override
public Bill getStoreBillByTopiaId(String topiaId) {
return TopiaEntityHelper.getEntityByTopiaId(storeBill, topiaId);
}
@Override
public Collection getStoreBillTopiaIds() {
Collection topiaIds = new LinkedList();
Collection tmp = getStoreBill();
if (tmp != null) {
for (TopiaEntity topiaEntity : tmp) {
topiaIds.add(topiaEntity.getTopiaId());
}
}
return topiaIds;
}
@Override
public int sizeStoreBill() {
if (storeBill == null) {
return 0;
}
return storeBill.size();
}
@Override
public boolean isStoreBillEmpty() {
int size = sizeStoreBill();
return size == 0;
}
@Override
public boolean isStoreBillNotEmpty() {
boolean empty = isStoreBillEmpty();
return ! empty;
}
@Override
public boolean containsStoreBill(Bill storeBill) {
boolean contains = this.storeBill !=null && this.storeBill.contains(storeBill);
return contains;
}
@Override
public void addDepartment(Department department) {
fireOnPreWrite(PROPERTY_DEPARTMENT, null, department);
if (this.department == null) {
this.department = new LinkedList();
}
department.setCompany(this);
this.department.add(department);
fireOnPostWrite(PROPERTY_DEPARTMENT, this.department.size(), null, department);
}
@Override
public void addAllDepartment(Iterable department) {
if (department == null) {
return;
}
for (Department item : department) {
addDepartment(item);
}
}
@Override
public void setDepartment(Collection department) {
// Copy elements to keep data for fire with new reference
Collection oldValue = this.department != null ? new LinkedList(this.department) : null;
fireOnPreWrite(PROPERTY_DEPARTMENT, oldValue, department);
this.department = department;
fireOnPostWrite(PROPERTY_DEPARTMENT, oldValue, department);
}
@Override
public void removeDepartment(Department department) {
fireOnPreWrite(PROPERTY_DEPARTMENT, department, null);
if (this.department == null || !this.department.remove(department)) {
throw new IllegalArgumentException("List does not contain given element");
}
department.setCompany(null);
fireOnPostWrite(PROPERTY_DEPARTMENT, this.department.size() + 1, department, null);
}
@Override
public void clearDepartment() {
if (this.department == null) {
return;
}
for (Department item : this.department) {
item.setCompany(null);
}
Collection oldValue = new LinkedList(this.department);
fireOnPreWrite(PROPERTY_DEPARTMENT, oldValue, this.department);
this.department.clear();
fireOnPostWrite(PROPERTY_DEPARTMENT, oldValue, this.department);
}
@Override
public Collection getDepartment() {
return department;
}
@Override
public Department getDepartmentByTopiaId(String topiaId) {
return TopiaEntityHelper.getEntityByTopiaId(department, topiaId);
}
@Override
public Collection getDepartmentTopiaIds() {
Collection topiaIds = new LinkedList();
Collection tmp = getDepartment();
if (tmp != null) {
for (TopiaEntity topiaEntity : tmp) {
topiaIds.add(topiaEntity.getTopiaId());
}
}
return topiaIds;
}
@Override
public int sizeDepartment() {
if (department == null) {
return 0;
}
return department.size();
}
@Override
public boolean isDepartmentEmpty() {
int size = sizeDepartment();
return size == 0;
}
@Override
public boolean isDepartmentNotEmpty() {
boolean empty = isDepartmentEmpty();
return ! empty;
}
@Override
public boolean containsDepartment(Department department) {
boolean contains = this.department !=null && this.department.contains(department);
return contains;
}
} //CompanyAbstract
© 2015 - 2024 Weber Informatics LLC | Privacy Policy