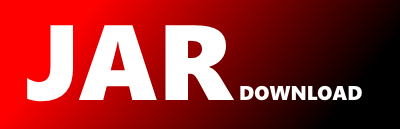
org.nuiton.topia.it.legacy.topiatest.PersonneAbstract Maven / Gradle / Ivy
The newest version!
package org.nuiton.topia.it.legacy.topiatest;
/*-
* #%L
* ToPIA :: IT
* %%
* Copyright (C) 2004 - 2024 Code Lutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import org.nuiton.i18n.I18n;
import org.nuiton.topia.it.legacy.topiatest.deletetest.Party2Impl;
import org.nuiton.topia.persistence.TopiaEntityVisitor;
import org.nuiton.topia.persistence.TopiaException;
import org.nuiton.topia.persistence.util.TopiaEntityHelper;
public abstract class PersonneAbstract extends Party2Impl implements Personne {
/**
* Nom de l'attribut en BD : name
*/
protected String name;
/**
* Nom de l'attribut en BD : otherNames
*/
protected Collection otherNames;
/**
* Nom de l'attribut en BD : gender
*/
protected Gender gender;
/**
* Nom de l'attribut en BD : otherGender
*/
protected Gender otherGender;
/**
* Nom de l'attribut en BD : address
*/
protected Address address;
/**
* Nom de l'attribut en BD : title
*/
protected List title;
private static final long serialVersionUID = 7364566692353290854L;
static {
I18n.n("topia.test.common.personne");
I18n.n("topia.test.common.name");
I18n.n("topia.test.common.otherNames");
I18n.n("topia.test.common.gender");
I18n.n("topia.test.common.otherGender");
I18n.n("topia.test.common.address");
I18n.n("topia.test.common.title");
}
@Override
public void accept(TopiaEntityVisitor visitor) throws TopiaException {
visitor.start(this);
accept0(visitor);
visitor.end(this);
}
@Override
protected void accept0(TopiaEntityVisitor visitor) throws TopiaException {
super.accept0(visitor);
visitor.visit(this, PROPERTY_NAME, String.class, name);
visitor.visit(this, PROPERTY_OTHER_NAMES, Collection.class, String.class, otherNames);
visitor.visit(this, PROPERTY_GENDER, Gender.class, gender);
visitor.visit(this, PROPERTY_OTHER_GENDER, Gender.class, otherGender);
visitor.visit(this, PROPERTY_ADDRESS, Address.class, address);
visitor.visit(this, PROPERTY_TITLE, List.class, Title.class, title);
}
@Override
public void setName(String name) {
String oldValue = this.name;
fireOnPreWrite(PROPERTY_NAME, oldValue, name);
this.name = name;
fireOnPostWrite(PROPERTY_NAME, oldValue, name);
}
@Override
public String getName() {
fireOnPreRead(PROPERTY_NAME, name);
String result = this.name;
fireOnPostRead(PROPERTY_NAME, name);
return result;
}
@Override
public void addOtherNames(String otherNames) {
fireOnPreWrite(PROPERTY_OTHER_NAMES, null, otherNames);
if (this.otherNames == null) {
this.otherNames = new LinkedList();
}
this.otherNames.add(otherNames);
fireOnPostWrite(PROPERTY_OTHER_NAMES, this.otherNames.size(), null, otherNames);
}
@Override
public void addAllOtherNames(Iterable otherNames) {
if (otherNames == null) {
return;
}
for (String item : otherNames) {
addOtherNames(item);
}
}
@Override
public void setOtherNames(Collection otherNames) {
// Copy elements to keep data for fire with new reference
Collection oldValue = this.otherNames != null ? new LinkedList(this.otherNames) : null;
fireOnPreWrite(PROPERTY_OTHER_NAMES, oldValue, otherNames);
this.otherNames = otherNames;
fireOnPostWrite(PROPERTY_OTHER_NAMES, oldValue, otherNames);
}
@Override
public void removeOtherNames(String otherNames) {
fireOnPreWrite(PROPERTY_OTHER_NAMES, otherNames, null);
if (this.otherNames == null || !this.otherNames.remove(otherNames)) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_OTHER_NAMES, this.otherNames.size() + 1, otherNames, null);
}
@Override
public void clearOtherNames() {
if (this.otherNames == null) {
return;
}
Collection oldValue = new LinkedList(this.otherNames);
fireOnPreWrite(PROPERTY_OTHER_NAMES, oldValue, this.otherNames);
this.otherNames.clear();
fireOnPostWrite(PROPERTY_OTHER_NAMES, oldValue, this.otherNames);
}
@Override
public Collection getOtherNames() {
return otherNames;
}
@Override
public int sizeOtherNames() {
if (otherNames == null) {
return 0;
}
return otherNames.size();
}
@Override
public boolean isOtherNamesEmpty() {
int size = sizeOtherNames();
return size == 0;
}
@Override
public boolean isOtherNamesNotEmpty() {
boolean empty = isOtherNamesEmpty();
return ! empty;
}
@Override
public boolean containsOtherNames(String otherNames) {
boolean contains = this.otherNames !=null && this.otherNames.contains(otherNames);
return contains;
}
@Override
public void setGender(Gender gender) {
Gender oldValue = this.gender;
fireOnPreWrite(PROPERTY_GENDER, oldValue, gender);
this.gender = gender;
fireOnPostWrite(PROPERTY_GENDER, oldValue, gender);
}
@Override
public Gender getGender() {
fireOnPreRead(PROPERTY_GENDER, gender);
Gender result = this.gender;
fireOnPostRead(PROPERTY_GENDER, gender);
return result;
}
@Override
public void setOtherGender(Gender otherGender) {
Gender oldValue = this.otherGender;
fireOnPreWrite(PROPERTY_OTHER_GENDER, oldValue, otherGender);
this.otherGender = otherGender;
fireOnPostWrite(PROPERTY_OTHER_GENDER, oldValue, otherGender);
}
@Override
public Gender getOtherGender() {
fireOnPreRead(PROPERTY_OTHER_GENDER, otherGender);
Gender result = this.otherGender;
fireOnPostRead(PROPERTY_OTHER_GENDER, otherGender);
return result;
}
@Override
public void setAddress(Address address) {
Address oldValue = this.address;
fireOnPreWrite(PROPERTY_ADDRESS, oldValue, address);
this.address = address;
fireOnPostWrite(PROPERTY_ADDRESS, oldValue, address);
}
@Override
public Address getAddress() {
fireOnPreRead(PROPERTY_ADDRESS, address);
Address result = this.address;
fireOnPostRead(PROPERTY_ADDRESS, address);
return result;
}
@Override
public void addTitle(Title title) {
fireOnPreWrite(PROPERTY_TITLE, null, title);
if (this.title == null) {
this.title = new LinkedList();
}
this.title.add(title);
fireOnPostWrite(PROPERTY_TITLE, this.title.size(), null, title);
}
@Override
public void addTitle(int index, Title title) {
fireOnPreWrite(PROPERTY_TITLE, null, title);
if (this.title == null) {
this.title = new LinkedList();
}
this.title.add(index, title);
fireOnPostWrite(PROPERTY_TITLE, index, null, title);
}
@Override
public void addAllTitle(Iterable title) {
if (title == null) {
return;
}
for (Title item : title) {
addTitle(item);
}
}
@Override
public void setTitle(List title) {
// Copy elements to keep data for fire with new reference
List oldValue = this.title != null ? new LinkedList(this.title) : null;
fireOnPreWrite(PROPERTY_TITLE, oldValue, title);
this.title = title;
fireOnPostWrite(PROPERTY_TITLE, oldValue, title);
}
@Override
public void removeTitle(Title title) {
fireOnPreWrite(PROPERTY_TITLE, title, null);
if (this.title == null || !this.title.remove(title)) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_TITLE, this.title.size() + 1, title, null);
}
@Override
public void removeTitle(int index) {
fireOnPreWrite(PROPERTY_TITLE, title, null);
if (this.title == null) {
throw new IllegalArgumentException("List does not contain given element");
}
Title oldValue = this.title.remove(index);
if (oldValue == null) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_TITLE, index, oldValue, null);
}
@Override
public void clearTitle() {
if (this.title == null) {
return;
}
List oldValue = new LinkedList(this.title);
fireOnPreWrite(PROPERTY_TITLE, oldValue, this.title);
this.title.clear();
fireOnPostWrite(PROPERTY_TITLE, oldValue, this.title);
}
@Override
public List getTitle() {
return title;
}
@Override
public Title getTitle(int index) {
return TopiaEntityHelper.getEntityByIndex(title, index);
}
@Override
public int sizeTitle() {
if (title == null) {
return 0;
}
return title.size();
}
@Override
public boolean isTitleEmpty() {
int size = sizeTitle();
return size == 0;
}
@Override
public boolean isTitleNotEmpty() {
boolean empty = isTitleEmpty();
return ! empty;
}
@Override
public boolean containsTitle(Title title) {
boolean contains = this.title !=null && this.title.contains(title);
return contains;
}
} //PersonneAbstract
© 2015 - 2025 Weber Informatics LLC | Privacy Policy