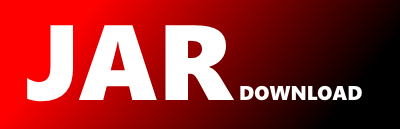
org.nuiton.topia.it.mapping.test6.A62Abstract Maven / Gradle / Ivy
The newest version!
package org.nuiton.topia.it.mapping.test6;
/*-
* #%L
* ToPIA :: IT
* %%
* Copyright (C) 2004 - 2024 Code Lutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.LinkedList;
import java.util.List;
import org.nuiton.topia.persistence.TopiaEntity;
import org.nuiton.topia.persistence.TopiaEntityVisitor;
import org.nuiton.topia.persistence.TopiaException;
import org.nuiton.topia.persistence.internal.AbstractTopiaEntity;
import org.nuiton.topia.persistence.util.TopiaEntityHelper;
public abstract class A62Abstract extends AbstractTopiaEntity implements A62 {
/**
* Nom de l'attribut en BD : b62
*/
protected List b62;
private static final long serialVersionUID = 4123437091997836087L;
@Override
public void accept(TopiaEntityVisitor visitor) throws TopiaException {
visitor.start(this);
accept0(visitor);
visitor.end(this);
}
protected void accept0(TopiaEntityVisitor visitor) throws TopiaException {
visitor.visit(this, PROPERTY_B62, List.class, B62.class, b62);
}
@Override
public void addB62(B62 b62) {
fireOnPreWrite(PROPERTY_B62, null, b62);
if (this.b62 == null) {
this.b62 = new LinkedList();
}
this.b62.add(b62);
fireOnPostWrite(PROPERTY_B62, this.b62.size(), null, b62);
}
@Override
public void addB62(int index, B62 b62) {
fireOnPreWrite(PROPERTY_B62, null, b62);
if (this.b62 == null) {
this.b62 = new LinkedList();
}
this.b62.add(index, b62);
fireOnPostWrite(PROPERTY_B62, index, null, b62);
}
@Override
public void addAllB62(Iterable b62) {
if (b62 == null) {
return;
}
for (B62 item : b62) {
addB62(item);
}
}
@Override
public void setB62(List b62) {
// Copy elements to keep data for fire with new reference
List oldValue = this.b62 != null ? new LinkedList(this.b62) : null;
fireOnPreWrite(PROPERTY_B62, oldValue, b62);
this.b62 = b62;
fireOnPostWrite(PROPERTY_B62, oldValue, b62);
}
@Override
public void removeB62(B62 b62) {
fireOnPreWrite(PROPERTY_B62, b62, null);
if (this.b62 == null || !this.b62.remove(b62)) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_B62, this.b62.size() + 1, b62, null);
}
@Override
public void removeB62(int index) {
fireOnPreWrite(PROPERTY_B62, b62, null);
if (this.b62 == null) {
throw new IllegalArgumentException("List does not contain given element");
}
B62 oldValue = this.b62.remove(index);
if (oldValue == null) {
throw new IllegalArgumentException("List does not contain given element");
}
fireOnPostWrite(PROPERTY_B62, index, oldValue, null);
}
@Override
public void clearB62() {
if (this.b62 == null) {
return;
}
List oldValue = new LinkedList(this.b62);
fireOnPreWrite(PROPERTY_B62, oldValue, this.b62);
this.b62.clear();
fireOnPostWrite(PROPERTY_B62, oldValue, this.b62);
}
@Override
public List getB62() {
return b62;
}
@Override
public B62 getB62(int index) {
return TopiaEntityHelper.getEntityByIndex(b62, index);
}
@Override
public B62 getB62ByTopiaId(String topiaId) {
return TopiaEntityHelper.getEntityByTopiaId(b62, topiaId);
}
@Override
public List getB62TopiaIds() {
List topiaIds = new LinkedList();
List tmp = getB62();
if (tmp != null) {
for (TopiaEntity topiaEntity : tmp) {
topiaIds.add(topiaEntity.getTopiaId());
}
}
return topiaIds;
}
@Override
public int sizeB62() {
if (b62 == null) {
return 0;
}
return b62.size();
}
@Override
public boolean isB62Empty() {
int size = sizeB62();
return size == 0;
}
@Override
public boolean isB62NotEmpty() {
boolean empty = isB62Empty();
return ! empty;
}
@Override
public boolean containsB62(B62 b62) {
boolean contains = this.b62 !=null && this.b62.contains(b62);
return contains;
}
} //A62Abstract
© 2015 - 2025 Weber Informatics LLC | Privacy Policy