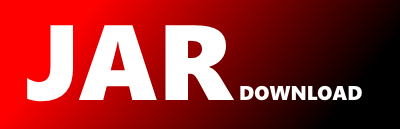
org.nuiton.topia.junit.AbstractDatabaseResource Maven / Gradle / Ivy
package org.nuiton.topia.junit;
/*
* #%L
* ToPIA :: JUnit
* $Id: AbstractDatabaseResource.java 2981 2014-01-17 17:38:55Z athimel $
* $HeadURL: http://svn.nuiton.org/svn/topia/tags/topia-3.0-alpha-11/topia-junit/src/main/java/org/nuiton/topia/junit/AbstractDatabaseResource.java $
* %%
* Copyright (C) 2004 - 2014 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.commons.lang3.reflect.FieldUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.cfg.Configuration;
import org.junit.rules.TestWatcher;
import org.junit.runner.Description;
import org.nuiton.topia.persistence.TopiaConfigurationConstants;
import org.nuiton.topia.persistence.internal.AbstractTopiaApplicationContext;
import org.nuiton.topia.persistence.internal.HibernateProvider;
import org.nuiton.topia.persistence.TopiaException;
import org.nuiton.topia.persistence.TopiaPersistenceContext;
import java.io.File;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.util.Properties;
/**
* Put this class as a Rule in test to obtain a new isolated db for each test.
*
* Here is a simple example of usage :
*
* public class MyTest {
*
* \@Rule
* public final TopiaDatabase db = new TopiaDatabase();
*
* \@Test
* public void testMethod() throws TopiaException {
*
* TopiaContext tx = db.beginTransaction();
* ...
* }
*
* The db created will be unique for each test method (and for each build also).
*
* You don't need to close any transaction, it will be done for you and the end
* of each method test.
*
* Created on 11/22/13.
*
* @author Tony Chemit
* @since 3.0
*/
public abstract class AbstractDatabaseResource> extends TestWatcher {
/** Logger. */
private static final Log log = LogFactory.getLog(AbstractDatabaseResource.class);
private File testBasedir;
private Properties dbConfiguration;
private ApplicationContext applicationContext;
private Configuration hibernateCfg;
private final String configurationPath;
protected abstract ApplicationContext createApplicationContext(Properties dbConfiguration);
protected abstract String getImplementationClassesAsString();
public AbstractDatabaseResource(String configurationPath) {
this.configurationPath = configurationPath;
}
@Override
protected void starting(Description description) {
// get test directory
testBasedir = ConfigurationHelper.getTestSpecificDirectory(
description.getTestClass(),
description.getMethodName());
if (log.isDebugEnabled()) {
log.debug("testBasedir = " + testBasedir);
}
// create the root context
try {
dbConfiguration = new Properties();
InputStream stream =
getClass().getResourceAsStream(configurationPath);
try {
dbConfiguration.load(stream);
} finally {
stream.close();
}
dbConfiguration.setProperty(
TopiaConfigurationConstants.CONFIG_PERSISTENCE_CLASSES,
getImplementationClassesAsString());
// make sure we always use a different directory
String dbPath = new File(testBasedir, "db").getAbsolutePath();
if (log.isDebugEnabled()) {
log.debug("dbPath = " + dbPath);
}
dbConfiguration.setProperty(
TopiaConfigurationConstants.CONFIG_URL, "jdbc:h2:file:" + dbPath);
onDbConfigurationCreate(dbConfiguration, testBasedir, dbPath);
applicationContext = createApplicationContext(dbConfiguration);
Field field = FieldUtils.getField(AbstractTopiaApplicationContext.class, "hibernateProvider", true);
HibernateProvider hibernateProvider = (HibernateProvider) field.get(applicationContext);
hibernateCfg = hibernateProvider.getHibernateConfiguration();
} catch (Exception e) {
throw new IllegalStateException(
"Could not start db at " + testBasedir, e);
}
}
@Override
public void finished(Description description) {
if (applicationContext != null && !applicationContext.isClosed()) {
try {
applicationContext.closeContext();
} catch (TopiaException e) {
if (log.isErrorEnabled()) {
log.error("Could not close topia root context", e);
}
}
}
applicationContext = null;
dbConfiguration = null;
hibernateCfg = null;
}
public File getTestBasedir() {
return testBasedir;
}
public Properties getDbConfiguration() {
return dbConfiguration;
}
public ApplicationContext getApplicationContext() {
return applicationContext;
}
public Configuration getHibernateCfg() {
return hibernateCfg;
}
public PersistenceContext beginTransaction() throws TopiaException {
return applicationContext.newPersistenceContext();
}
protected void onDbConfigurationCreate(Properties configuration,
File testDir,
String dbPath) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy