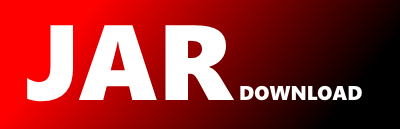
org.nuiton.topia.replication.model.ReplicationNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topia-service-replication Show documentation
Show all versions of topia-service-replication Show documentation
Hibernate based replication service
/*
* #%L
* ToPIA :: Service Replication
*
* $Id: ReplicationNode.java 2245 2011-04-14 12:47:09Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/topia/tags/topia-2.6.6/topia-service-replication/src/main/java/org/nuiton/topia/replication/model/ReplicationNode.java $
* %%
* Copyright (C) 2004 - 2010 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.topia.replication.model;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.topia.persistence.TopiaEntity;
import org.nuiton.topia.persistence.TopiaEntityEnum;
import org.nuiton.topia.persistence.util.EntityOperator;
import org.nuiton.topia.persistence.util.EntityOperatorStore;
import org.nuiton.topia.replication.TopiaReplicationOperationUndoable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Model of a replication's node.
*
* The invariant of a replication's node is his {@link #contract}, means the
* type of entity to replicate.
*
* @author tchemit
* @since 2.2.0
*/
public class ReplicationNode {
/** Logger */
private static final Log log = LogFactory.getLog(ReplicationNode.class);
/** contract of entity of the node. */
protected final TopiaEntityEnum contract;
/** entity operator. */
protected final EntityOperator super TopiaEntity> operator;
/** shell of the node. */
protected Set shell;
/**
* dictionnary of associations defined on the node (keys are association
* name, and values are target node).
*/
protected final Map associations;
/** names of association to dettach while replication. */
protected final Set associationsToDettach;
/**
* compositions defined on the node (keys are association name,
* and values are target node).
*/
protected final Map dependencies;
/** names of dependency to dettach while replication. */
protected final Set dependenciesToDettach;
/** operations to fire when replication pass on this node. */
protected final List operations;
public ReplicationNode(TopiaEntityEnum contract) {
this.contract = contract;
operator = EntityOperatorStore.getOperator(contract);
associations = new HashMap();
dependencies = new HashMap();
shell = new HashSet();
associationsToDettach = new HashSet();
dependenciesToDettach = new HashSet();
operations = new ArrayList();
if (log.isTraceEnabled()) {
log.trace("new node : " + this);
}
}
public void addAssociation(String name, ReplicationNode node) {
associations.put(name, node);
}
public void addOperation(int index, ReplicationOperationDef op) {
operations.add(index, op);
if (log.isDebugEnabled()) {
log.debug(op + " to node " + this);
}
}
public void addOperation(ReplicationOperationDef op) {
operations.add(op);
if (log.isDebugEnabled()) {
log.debug(op + " to node " + this);
}
}
public void setOperations(List operations) {
clearOperations();
this.operations.addAll(operations);
}
public ReplicationOperationDef[] getOperations() {
return operations.toArray(
new ReplicationOperationDef[operations.size()]);
}
public ReplicationOperationDef[] getUndoableOperations() {
List result =
new ArrayList();
for (ReplicationOperationDef operation : operations) {
if (TopiaReplicationOperationUndoable.class.isAssignableFrom(
operation.getOperationClass())) {
result.add(operation);
}
}
return result.toArray(new ReplicationOperationDef[result.size()]);
}
public boolean hasAssociation() {
return !associations.isEmpty();
}
public boolean hasAssociationsToDettach() {
return !associationsToDettach.isEmpty();
}
public String[] getAssociationsDettached(ReplicationNode node) {
Set result = new HashSet();
for (String name : associationsToDettach) {
ReplicationNode get = associations.get(name);
if (node.equals(get)) {
result.add(name);
}
}
return result.toArray(new String[result.size()]);
}
public String[] getDependenciesDettached(ReplicationNode node) {
Set result = new HashSet();
for (String name : dependenciesToDettach) {
ReplicationNode get = dependencies.get(name);
if (node.equals(get)) {
result.add(name);
}
}
return result.toArray(new String[result.size()]);
}
public boolean hasDependenciesToDettach() {
return !dependenciesToDettach.isEmpty();
}
public boolean hasDependency() {
return !dependencies.isEmpty();
}
public void addDependency(String name, ReplicationNode node) {
dependencies.put(name, node);
}
public void addAssociationToDettach(String key) {
associationsToDettach.add(key);
}
public void addDependencyToDettach(String key) {
dependenciesToDettach.add(key);
}
public Map getAssociations() {
return associations;
}
public Set getAssociationsToDettach() {
return associationsToDettach;
}
public Set getDependenciesToDettach() {
return dependenciesToDettach;
}
public TopiaEntityEnum getContract() {
return contract;
}
public Class extends TopiaEntity> getEntityType() {
return contract.getContract();
}
public EntityOperator super TopiaEntity> getOperator() {
return operator;
}
public Map getDependencies() {
return dependencies;
}
public Set getShell() {
return shell;
}
public void setShell(Set shell) {
this.shell = shell;
}
/**
* sort operation by their phase.
*
* @see ReplicationOperationPhase
*/
public void sortOperations() {
Collections.sort(operations);
}
/**
* Remove all operation of the node (for example when no data is associated
* with the type of the node, then no needed operations).
*/
public void clearOperations() {
operations.clear();
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
ReplicationNode other = (ReplicationNode) obj;
return contract == other.contract;
}
@Override
public int hashCode() {
int hash = 7;
hash = 37 * hash + contract.hashCode();
return hash;
}
@Override
public String toString() {
return contract.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy