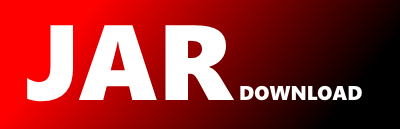
org.nuiton.topia.replication.TopiaReplicationOperationProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topia-service-replication Show documentation
Show all versions of topia-service-replication Show documentation
Hibernate based replication service
The newest version!
package org.nuiton.topia.replication;
/*
* #%L
* ToPIA :: Service Replication
* $Id$
* $HeadURL$
* %%
* Copyright (C) 2004 - 2014 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.topia.persistence.util.TopiaEntityHelper;
import org.nuiton.topia.replication.model.ReplicationOperationDef;
import java.util.ArrayList;
import java.util.List;
import java.util.ServiceLoader;
/**
* Provider of {@link TopiaReplicationOperation}.
*
* @author Tony Chemit - [email protected]
* @since 2.4.3
*/
public class TopiaReplicationOperationProvider {
/** Logger */
private static final Log log =
LogFactory.getLog(TopiaReplicationOperationProvider.class);
/**
* All available operations detected via a {@link ServiceLoader} on
* contract {@link TopiaReplicationOperation}.
*/
protected TopiaReplicationOperation[] operations;
/**
* Obtains all {@link TopiaReplicationOperation} available
* via {@link ServiceLoader}.
*
* If {@link #operations} is null, then load operations, otherwise just
* return the already computed result.
*
* @return the array of all available operations
*/
public TopiaReplicationOperation[] getOperations() {
if (operations == null) {
// chargement des operations disponibles une seule fois
ServiceLoader loader =
ServiceLoader.load(TopiaReplicationOperation.class);
List operations =
new ArrayList();
for (TopiaReplicationOperation op : loader) {
if (log.isDebugEnabled()) {
log.debug("detected operation " + op);
}
operations.add(op);
}
this.operations = operations.toArray(
new TopiaReplicationOperation[operations.size()]);
}
return operations;
}
/**
* Obtains the instanciated (and initialized) operation of the given type.
*
* @param operationClass type of searched operation
* @return the found operation, or {@code null} if not found.
*/
public TopiaReplicationOperation getOperation(
Class extends TopiaReplicationOperation> operationClass) {
TopiaEntityHelper.checkNotNull("getOperation", "operationClass",
operationClass);
TopiaReplicationOperation result = null;
for (TopiaReplicationOperation op : getOperations()) {
if (operationClass.isAssignableFrom(op.getClass())) {
result = op;
break;
}
}
return result;
}
/**
* Obtains the instanciated (and initialized) operation of the given
* operation definition.
*
* @param operationDef operation definition of searched operation
* @return the found operation, or {@code null} if not found.
*/
public TopiaReplicationOperation getOperation(ReplicationOperationDef operationDef) {
TopiaEntityHelper.checkNotNull("getOperation", "operationDef",
operationDef);
return getOperation(operationDef.getOperationClass());
}
public TopiaReplicationOperationUndoable getUndoableOperation(ReplicationOperationDef operationDef) throws IllegalArgumentException {
TopiaReplicationOperation operation = getOperation(operationDef);
if (!(operation instanceof TopiaReplicationOperationUndoable)) {
throw new IllegalArgumentException(
"the operation " + operation + " is not undoable");
}
return (TopiaReplicationOperationUndoable) operation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy