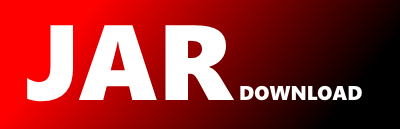
org.nuiton.topia.service.TopiaApplicationServiceFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topia-soa Show documentation
Show all versions of topia-soa Show documentation
Service Oriented Architecture module
/* *##%
* ToPIA :: SOA
* Copyright (C) 2004 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*/
/**
*
*/
package org.nuiton.topia.service;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.topia.TopiaContext;
import org.nuiton.topia.TopiaException;
import org.nuiton.topia.TopiaNotFoundException;
import org.nuiton.topia.framework.TopiaUtil;
import org.nuiton.topia.service.clients.RMIProxy;
import org.nuiton.topia.service.clients.SOAPProxy;
import org.nuiton.topia.service.clients.XMLRPCProxy;
import java.lang.reflect.Proxy;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
/**
* TopiaServiceFactory.java
*
* Classe utilisee pour charger les services.
*
* Deux utilisations possibles :
* client: pour avoir une interface sur un service local ou distant
* serveur: pour avoir un service local au serveur
*
* Sert aussi au serveur pour declarer des services
*
* @author chatellier
* @version $Revision: 1715 $
*
* Last update : $Date: 2009-12-15 01:26:16 +0100 (mar. 15 déc. 2009) $ By : $Author: tchemit $
*/
public class TopiaApplicationServiceFactory {
/** Fichier de configuration par defaut */
public static final String DEFAULT_CONFIG_PROPERTIES = "TopiaContextImpl.properties";
/** Nom de la propriete de definition des services utilises */
public static final String TOPIA_APPLICATION_SERVICE_BEGIN = "topia.application.service.";
/** Nom de la propriete de definition des services fournit */
public static final String TOPIA_APPLICATION_PROVIDE_BEGIN = "topia.application.provide.";
/** Nom de la propriete de definition des ports suivant les protocoles */
public static final String TOPIA_APPLICATION_SERVER_PORT_BEGIN = "topia.application.server.port.";
/**
* Nom du dossier ou sont generer certains fichiers (doit etre dans le
* classpath )
*/
public static String TOPIA_GENERATION_DIRECTORY = "topiagen";
/** Fichier de configuration */
protected static Properties config;
/** Dispatcher (servers) */
protected static final TopiaServiceProvider mainDispatcher = new TopiaServiceProvider();
/**
* Stockage des services deja instancies
*/
private static Map, TopiaApplicationService> mapServiceCache = new HashMap, TopiaApplicationService>();
/** Logger (common logging) */
private static final Log log = LogFactory
.getLog(TopiaApplicationServiceFactory.class);
protected static TopiaContext defaultServiceContext;
/**
* Retourne la configuration. Charge le fichier s'il n'a pas deja ete
* charge.
*
* @throws TopiaNotFoundException
* si le fichier de configuration ne peut pas etre charge
* @return la configuration (et la charge si cela n'est pas déjà fait)
*/
static Properties getConfiguration() throws TopiaNotFoundException {
if (config == null) {
config = TopiaUtil.getProperties(DEFAULT_CONFIG_PROPERTIES);
}
return config;
}
/**
* Charge et lance tous les services contenus dans le fichier de
* configuration
*
* @param config
* les proprietes du fichier de configuration
* @param context
* le contexte pere des contextes fournis aux services
* @throws TopiaException if any pb with topia
*/
public static void loadServices(Properties config, TopiaContext context)
throws TopiaException {
if (context == null) {
throw new NullPointerException(
"I need a valid TopiaContext to initialise application services");
}
defaultServiceContext = context;
// lecture du fichier de configuration
if (config == null) {
try {
config = getConfiguration();
} catch (TopiaNotFoundException e) {
throw new TopiaNotFoundException(
"Can't find configuration file "
+ DEFAULT_CONFIG_PROPERTIES);
}
}
// pour chaque service applicatif
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy