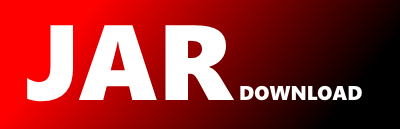
org.nuiton.topia.service.clients.RMIProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topia-soa Show documentation
Show all versions of topia-soa Show documentation
Service Oriented Architecture module
/* *##%
* ToPIA :: SOA
* Copyright (C) 2004 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*/
package org.nuiton.topia.service.clients;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URI;
import java.rmi.Remote;
import java.rmi.registry.LocateRegistry;
import java.util.Arrays;
import org.apache.commons.beanutils.MethodUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.topia.TopiaContext;
import org.nuiton.topia.TopiaException;
import org.nuiton.topia.service.TopiaApplicationService;
import org.nuiton.topia.service.TopiaProxy;
/**
* RMIProxy.java
*
* @author chatellier
* @version $Revision: 1715 $
*
* Last update : $Date: 2009-12-15 01:26:16 +0100 (mar. 15 déc. 2009) $ By : $Author: tchemit $
*/
public class RMIProxy implements TopiaProxy {
/** Logger (common logging) */
private static final Log logger = LogFactory.getLog(RMIProxy.class);
/** location du service */
protected String serviceLocation = null;
/** La classe geree par le proxy */
protected Class extends TopiaApplicationService> clazz;
/**
* Constructeur
*
*/
public RMIProxy() {
}
/*
* (non-Javadoc)
*
* @see java.lang.reflect.InvocationHandler#invoke(java.lang.Object,
* java.lang.reflect.Method, java.lang.Object[])
*/
public Object invoke(Object proxy, Method method, Object[] args)
throws Throwable {
Object result = null;
logger.debug("Invoke : " + clazz.getName() + "." + method.getName()
+ "(" + Arrays.toString(args) + ")");
Remote rObject = LocateRegistry.getRegistry().lookup(clazz.getName());
logger.debug("Interfaces : "
+ Arrays.toString(rObject.getClass().getInterfaces()));
logger.debug("Lookup for rmi service : rmi://" + this.serviceLocation
+ "/" + clazz.getName() + " is " + rObject);
logger.warn(Arrays.toString(rObject.getClass().getInterfaces()));
try {
// l'objet Remote est en fait du type de la classe
// Remote generee sur le serveur
// on ne l'a pas ici
// invocation via MethodUtils de commons beanutils
result = MethodUtils.invokeMethod(rObject, method.getName(), args);
} catch (IllegalAccessException e) {
new TopiaException("Illegal Access to method (" + method.getName()
+ ") in interface " + clazz.getName());
} catch (InvocationTargetException e) {
new TopiaException("Can't call method (" + method.getName()
+ ") in interface " + clazz.getName());
}
return result;
}
/*
* (non-Javadoc)
*
* @see org.nuiton.topia.service.clients.TopiaProxy#setURI(java.net.URI)
*/
public void setURI(URI uri) {
// serviceLocation = uri.getRawSchemeSpecificPart();
serviceLocation = uri.getAuthority(); // = host + port si specifie
}
/*
* (non-Javadoc)
*
* @see org.nuiton.topia.service.clients.TopiaProxy#setClass(java.lang.Class)
*/
public void setClass(Class extends TopiaApplicationService> clazz) {
this.clazz = clazz;
}
public void destroy() {
}
public void init(TopiaContext context) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy