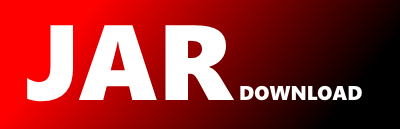
org.nuiton.topia.service.servers.RMIServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topia-soa Show documentation
Show all versions of topia-soa Show documentation
Service Oriented Architecture module
/* *##%
* ToPIA :: SOA
* Copyright (C) 2004 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*/
package org.nuiton.topia.service.servers;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.rmi.Remote;
import java.rmi.RemoteException;
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
import java.util.Arrays;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.topia.service.TopiaApplicationService;
import org.nuiton.topia.service.TopiaServiceServerAbstract;
import sun.rmi.server.UnicastServerRef;
/**
* RMIServer.java
*
* @author chatellier
* @version $Revision: 1715 $
*
* Last update : $Date: 2009-12-15 01:26:16 +0100 (mar. 15 déc. 2009) $ By : $Author: tchemit $
*/
public class RMIServer extends TopiaServiceServerAbstract implements Runnable,
InvocationHandler {
/** Logger (common logging) */
private static final Log logger = LogFactory.getLog(RMIServer.class);
/** Port de rmiregistry */
protected final static int DEFAULT_PORT = 1099;
/** instance de registry */
protected Registry registry;
/** Server already launched */
protected boolean alreadyLaunched = false;
/**
* Constructeur
* @throws RemoteException si la creation echoue
*/
public RMIServer() throws RemoteException {
this(DEFAULT_PORT);
}
/**
* Constructeur
* @throws RemoteException si la creation echoue
*/
public RMIServer(int port) throws RemoteException {
// lance rmiregistry
registry = LocateRegistry.createRegistry(port);
logger.info("Starting RMIRegistry on port " + port + "...");
}
/*
* (non-Javadoc)
*
* @see org.nuiton.topia.service.TopiaServiceServer#addService(java.lang.Class)
*/
public void addService(final Class extends TopiaApplicationService> clazz) {
// /lDelegationInterfaces.add(clazz);
// meProxy est le proxy que l'on va bind dans RMI
// meProxy pointe sur this
// create class that implements Remote and throws RemoteException
// for all methods
try {
Class clazz2 = RemoteClassLoader.getRemoteClass(clazz);
// logger.debug("Interface list = " +
// Arrays.toString(clazz2.getInterfaces()));
Remote meProxy = (Remote) Proxy.newProxyInstance(clazz2
.getClassLoader(), new Class[] { clazz2 }, this);
Remote stub = new UnicastServerRef(false).exportObject(meProxy,
null, false);
registry.rebind(clazz.getName(), stub);
logger.info("Binding new rmi service = " + clazz.getName());
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (RemoteException e) {
e.printStackTrace();
} catch (SecurityException e) {
e.printStackTrace();
}
}
/*
* (non-Javadoc)
*
* @see java.lang.Runnable#run()
*/
public void run() {
logger.info("RMI Server running...");
while (true) {
// TODO change (attente passive)
try {
Thread.sleep(Long.MAX_VALUE);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
/*
* (non-Javadoc)
*
* @see java.lang.reflect.InvocationHandler#invoke(java.lang.Object,
* java.lang.reflect.Method, java.lang.Object[])
*/
public Object invoke(Object proxy, Method method, Object[] args)
throws Throwable {
// log
logger.debug("Invoke RMIServer = " + method.getName() + "("
+ Arrays.toString(args) + ")");
Class clazzToCall = method.getDeclaringClass();
// for(Class extends TopiaApplicationService> localClazz :
// lDelegationInterfaces) {
// Class[] interfaces = proxy.getClass().getInterfaces();
// List asList = Arrays.asList(interfaces);
// if (asList.contains(localClazz)) { // instanceOf marche pas ici
// clazzToCall = localClazz;
// }
// }
Class realClass = Class.forName(RemoteClassLoader
.getOriginClassName(clazzToCall));
Method realMethod = realClass.getMethod(method.getName(),
(Class[]) method.getParameterTypes());
Object result = null;
if (clazzToCall != null) {
logger.debug("Requested class = " + realClass.getName());
result = super.invoke(realMethod, args);
}
return result;
}
/*
* (non-Javadoc)
*
* @see org.nuiton.topia.service.TopiaServiceServer#launch()
*/
public void launch() {
if (!alreadyLaunched) {
(new Thread(this)).start();
alreadyLaunched = true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy