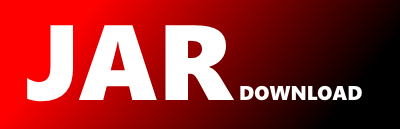
org.nuiton.web.gwt.table.AbstractGWTTableModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nuiton-gwt Show documentation
Show all versions of nuiton-gwt Show documentation
Extra classes for GWT based applications
The newest version!
/*
* #%L
* Nuiton Web :: Nuiton GWT
* %%
* Copyright (C) 2010 - 2011 CodeLutin, Jean Couteau
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.web.gwt.table;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.EventListener;
import java.util.List;
/**
* @author jcouteau
* @since 1.1
*/
public abstract class AbstractGWTTableModel implements GWTTableModel {
private static final long serialVersionUID = -5798593159423650347L;
/**
* Storage for the listeners registered with this model.
*/
protected List listenerList = new ArrayList();
/**
* Creates a default instance.
*/
public AbstractGWTTableModel() {
// no setup required here
}
/**
* Returns the name of the specified column. This method generates default
* names in a sequence (starting with column 0): A, B, C, ..., Z, AA, AB,
* AC, ..., AZ, BA, BB, BC, and so on. Subclasses may override this method
* to allow column names to be specified on some other basis.
*
* @param columnIndex the column index.
*
* @return The name of the column.
*/
public String getColumnName(int columnIndex) {
StringBuffer buffer = new StringBuffer();
while (columnIndex >= 0) {
buffer.insert(0, (char) ('A' + columnIndex % 26));
columnIndex = columnIndex / 26 - 1;
}
return buffer.toString();
}
/**
* Return the index of the specified column, or -1
if there is
* no column with the specified name.
*
* @param columnName the name of the column (null
not permitted).
*
* @return The index of the column, -1 if not found.
*
* @see #getColumnName(int)
* @throws NullPointerException if columnName
is
* null
.
*/
public int findColumn(String columnName) {
int count = getColumnCount();
for (int index = 0; index < count; index++) {
String name = getColumnName(index);
if (columnName.equals(name)) {
return index;
}
}
// Unable to locate.
return -1;
}
/**
* Returns the Class
for all Object
instances
* in the specified column.
*
* @param columnIndex the column index.
*
* @return The class.
*/
public Class> getColumnClass(int columnIndex) {
return Object.class;
}
/**
* Returns true
if the specified cell is editable, and
* false
if it is not. This implementation returns
* false
for all arguments, subclasses should override the
* method if necessary.
*
* @param rowIndex the row index of the cell.
* @param columnIndex the column index of the cell.
*
* @return false
.
*/
public boolean isCellEditable(int rowIndex, int columnIndex) {
return false;
}
/**
* Sets the value of the given cell. This implementation ignores all
* arguments and does nothing, subclasses should override the
* method if necessary.
*
* @param value the new value (null
permitted).
* @param rowIndex the row index of the cell.
* @param columnIndex the column index of the cell.
*/
public void setValueAt(Object value, int rowIndex, int columnIndex) {
// Do nothing...
}
/**
* Adds a listener to the table model. The listener will receive notification
* of all changes to the table model.
*
* @param listener the listener.
*/
public void addTableModelListener(TableModelListener listener) {
listenerList.add(listener);
}
/**
* Removes a listener from the table model so that it will no longer receive
* notification of changes to the table model.
*
* @param listener the listener to remove.
*/
public void removeTableModelListener(TableModelListener listener) {
listenerList.remove(listener);
}
/**
* Returns an array containing the listeners that have been added to the
* table model.
*
* @return Array of {@link TableModelListener} objects.
*
* @since 1.4
*/
public TableModelListener[] getTableModelListeners() {
TableModelListener[] listeners = new TableModelListener[]{};
listeners = listenerList.toArray(listeners);
return listeners;
}
/**
* Sends a {@link TableModelEvent} to all registered listeners to inform
* them that the table data has changed.
*/
public void fireTableDataChanged() {
fireTableChanged(new TableModelEvent(this, 0, Integer.MAX_VALUE));
}
/**
* Sends a {@link TableModelEvent} to all registered listeners to inform
* them that the table structure has changed.
*/
public void fireTableStructureChanged() {
fireTableChanged(new TableModelEvent(this, TableModelEvent.HEADER_ROW));
}
/**
* Sends a {@link TableModelEvent} to all registered listeners to inform
* them that some rows have been inserted into the model.
*
* @param firstRow the index of the first row.
* @param lastRow the index of the last row.
*/
public void fireTableRowsInserted(int firstRow, int lastRow) {
fireTableChanged(new TableModelEvent(this, firstRow, lastRow,
TableModelEvent.ALL_COLUMNS, TableModelEvent.INSERT));
}
/**
* Sends a {@link TableModelEvent} to all registered listeners to inform
* them that some rows have been updated.
*
* @param firstRow the index of the first row.
* @param lastRow the index of the last row.
*/
public void fireTableRowsUpdated(int firstRow, int lastRow) {
fireTableChanged(new TableModelEvent(this, firstRow, lastRow,
TableModelEvent.ALL_COLUMNS, TableModelEvent.UPDATE));
}
/**
* Sends a {@link TableModelEvent} to all registered listeners to inform
* them that some rows have been deleted from the model.
*
* @param firstRow the index of the first row.
* @param lastRow the index of the last row.
*/
public void fireTableRowsDeleted(int firstRow, int lastRow) {
fireTableChanged(new TableModelEvent(this, firstRow, lastRow,
TableModelEvent.ALL_COLUMNS, TableModelEvent.DELETE));
}
/**
* Sends a {@link TableModelEvent} to all registered listeners to inform
* them that a single cell has been updated.
*
* @param row the row index.
* @param column the column index.
*/
public void fireTableCellUpdated(int row, int column) {
fireTableChanged(new TableModelEvent(this, row, row, column));
}
/**
* Sends the specified event to all registered listeners.
*
* @param event the event to send.
*/
public void fireTableChanged(TableModelEvent event) {
TableModelListener[] list = getTableModelListeners();
for (TableModelListener listener:list){
listener.tableChanged(event);
}
}
/**
* Returns an array of listeners of the given type that are registered with
* this model.
*
* @param listenerType the listener class.
*
* @return An array of listeners (possibly empty).
*/
public T[] getListeners(Class listenerType) {
if (listenerType.getName().equals(TableModelListener.class.getName())){
return (T[])getTableModelListeners();
} else {
return (T[])new Object[]{};
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy