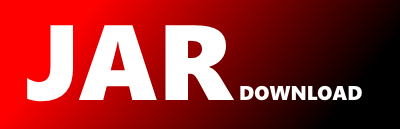
org.nuiton.web.security.SecurityUserAbstract Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nuiton-security Show documentation
Show all versions of nuiton-security Show documentation
Security module based on ToPIA
package org.nuiton.web.security;
/*-
* #%L
* Nuiton Web :: Nuiton Security
* %%
* Copyright (C) 2010 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Collection;
import java.util.LinkedList;
import javax.annotation.Generated;
import org.nuiton.topia.persistence.TopiaEntity;
import org.nuiton.topia.persistence.TopiaEntityVisitor;
import org.nuiton.topia.persistence.TopiaException;
import org.nuiton.topia.persistence.internal.AbstractTopiaEntity;
import org.nuiton.topia.persistence.util.TopiaEntityHelper;
@Generated(value = "org.nuiton.topia.templates.EntityTransformer", date = "Thu Sep 07 10:56:15 CEST 2017")
public abstract class SecurityUserAbstract extends AbstractTopiaEntity implements SecurityUser {
/**
* Nom de l'attribut en BD : login
*/
protected String login;
/**
* Nom de l'attribut en BD : password
*/
protected String password;
/**
* Nom de l'attribut en BD : externalId
*/
protected String externalId;
/**
* Nom de l'attribut en BD : securityRole
*/
protected Collection securityRole;
private static final long serialVersionUID = 3631647531095843635L;
@Override
public void accept(TopiaEntityVisitor visitor) throws TopiaException {
visitor.start(this);
accept0(visitor);
visitor.end(this);
}
protected void accept0(TopiaEntityVisitor visitor) throws TopiaException {
visitor.visit(this, PROPERTY_LOGIN, String.class, login);
visitor.visit(this, PROPERTY_PASSWORD, String.class, password);
visitor.visit(this, PROPERTY_EXTERNAL_ID, String.class, externalId);
visitor.visit(this, PROPERTY_SECURITY_ROLE, Collection.class, SecurityRole.class, securityRole);
}
@Override
public void setLogin(String login) {
this.login = login;
}
@Override
public String getLogin() {
String result = this.login;
return result;
}
@Override
public void setPassword(String password) {
this.password = password;
}
@Override
public String getPassword() {
String result = this.password;
return result;
}
@Override
public void setExternalId(String externalId) {
this.externalId = externalId;
}
@Override
public String getExternalId() {
String result = this.externalId;
return result;
}
@Override
public void addSecurityRole(SecurityRole securityRole) {
if (this.securityRole == null) {
this.securityRole = new LinkedList();
}
this.securityRole.add(securityRole);
}
@Override
public void addAllSecurityRole(Iterable securityRole) {
if (securityRole == null) {
return;
}
for (SecurityRole item : securityRole) {
addSecurityRole(item);
}
}
@Override
public void setSecurityRole(Collection securityRole) {
this.securityRole = securityRole;
}
@Override
public void removeSecurityRole(SecurityRole securityRole) {
if (this.securityRole == null || !this.securityRole.remove(securityRole)) {
throw new IllegalArgumentException("List does not contain given element");
}
}
@Override
public void clearSecurityRole() {
if (this.securityRole == null) {
return;
}
this.securityRole.clear();
}
@Override
public Collection getSecurityRole() {
return securityRole;
}
@Override
public SecurityRole getSecurityRoleByTopiaId(String topiaId) {
return TopiaEntityHelper.getEntityByTopiaId(securityRole, topiaId);
}
@Override
public Collection getSecurityRoleTopiaIds() {
Collection topiaIds = new LinkedList();
Collection tmp = getSecurityRole();
if (tmp != null) {
for (TopiaEntity topiaEntity : tmp) {
topiaIds.add(topiaEntity.getTopiaId());
}
}
return topiaIds;
}
@Override
public int sizeSecurityRole() {
if (securityRole == null) {
return 0;
}
return securityRole.size();
}
@Override
public boolean isSecurityRoleEmpty() {
int size = sizeSecurityRole();
return size == 0;
}
@Override
public boolean isSecurityRoleNotEmpty() {
boolean empty = isSecurityRoleEmpty();
return ! empty;
}
@Override
public boolean containsSecurityRole(SecurityRole securityRole) {
boolean contains = this.securityRole !=null && this.securityRole.contains(securityRole);
return contains;
}
} //SecurityUserAbstract
© 2015 - 2025 Weber Informatics LLC | Privacy Policy