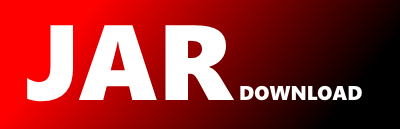
org.nuiton.wikitty.ScriptEvaluator Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2011 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.script.Bindings;
import javax.script.ScriptEngine;
import javax.script.ScriptEngineFactory;
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class ScriptEvaluator {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(ScriptEvaluator.class);
static protected ScriptEngineManager scriptEnginManager;
/**
* Return all time new ScriptEnginManager if classLoader is not null
* else return default ScriptEnginManager
*
* @param classLoader ClassLoader used to looking for ScriptEngin, can be null
* @return ScriptEnginManager
*/
static public ScriptEngineManager getScriptEnginManager(ClassLoader classLoader) {
ScriptEngineManager result;
if (classLoader != null) {
result = new ScriptEngineManager(classLoader);
} else {
if (scriptEnginManager == null) {
// create default ScriptEngineManager
scriptEnginManager = new ScriptEngineManager();
}
result = scriptEnginManager;
}
return result;
}
/**
*
* @param classLoader
* @param name only used in exception message (this help to determine what
* script failed)
* @param mimetype script engine looking for this specific mimetype
* @return ScriptEngine or exception if not available for this mimetype
*/
static public ScriptEngine getScriptEngin(ClassLoader classLoader,
String name, String mimetype) {
ScriptEngineManager scriptEnginManager = getScriptEnginManager(classLoader);
ScriptEngine scriptEngin = scriptEnginManager.getEngineByMimeType(mimetype);
if (scriptEngin == null) {
List factories =
scriptEnginManager.getEngineFactories();
String msgFactories = "";
for (ScriptEngineFactory f : factories) {
msgFactories += String.format(
"\n%s extensions: %s mimetypes: %s",
f.getEngineName(), f.getExtensions(), f.getMimeTypes());
}
throw new WikittyException(String.format(
"Can't find engine for %s(%s). Available engines: %s",
name, mimetype, msgFactories));
} else {
return scriptEngin;
}
}
/**
* Evalue le script et retourne le retour de l'evaluation
*
* @param classLoader optionnal classLoader used to find ScriptEngine
* @param name
* @param script
* @param mimetype
* @param bindings
* @return
*/
static public Object eval(ClassLoader classLoader, String name,
String script, String mimetype, Map bindings) {
ScriptEngine scriptEngin = getScriptEngin(classLoader, name, mimetype);
Bindings b = scriptEngin.createBindings();
b.putAll(bindings);
try {
Object result = scriptEngin.eval(script, b);
return result;
} catch (ScriptException eee) {
throw new WikittyException(String.format(
"Can't evaluated script %s(%s=>%s) script was\n%s",
name, mimetype,
scriptEngin.getFactory().getEngineName(), script), eee);
}
}
/**
* Evalue le script et recupere a la fin de l'evaluation les valeurs
* des variables presentes dans la map bindings. Le resultat a exactement
* les memes cles que binding.
*
* @param classLoader optionnal classLoader used to find ScriptEngine
* @param name
* @param script
* @param mimetype
* @param bindings
* @return
*/
static public Map exec(ClassLoader classLoader, String name,
String script, String mimetype, Map bindings) {
ScriptEngine scriptEngin = getScriptEngin(classLoader, name, mimetype);
Bindings b = scriptEngin.createBindings();
b.putAll(bindings);
try {
scriptEngin.eval(script, b);
Map result = new HashMap();
for (Map.Entry e : bindings.entrySet()) {
Object value = scriptEngin.get(e.getKey());
result.put(e.getKey(), value);
}
return result;
} catch (ScriptException eee) {
throw new WikittyException(String.format(
"Can't evaluated script %s(%s=>%s) script was\n%s",
name, mimetype,
scriptEngin.getFactory().getEngineName(), script), eee);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy