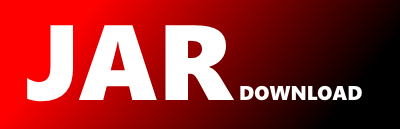
org.nuiton.wikitty.addons.WikittyI18nImpl Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.addons;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.util.StringUtil;
import org.nuiton.wikitty.entities.BusinessEntityImpl;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.entities.WikittyExtension;
import org.nuiton.wikitty.entities.WikittyI18nAbstract;
/**
* WikittyI18n permet de gerer les traductions des champs des extensions.
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class WikittyI18nImpl extends WikittyI18nAbstract
implements PropertyChangeListener {
private static final long serialVersionUID = 3824481585361443459L;
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(WikittyI18nImpl.class);
/** contient les translations sous une forme plus simple a utiliser */
transient protected Map> translationsCache = null;
/**
* WikittyI18nImpl :
*/
public WikittyI18nImpl() {
super();
}
/**
* WikittyI18nImpl :
* @param wikitty
*/
public WikittyI18nImpl(Wikitty wikitty) {
super(wikitty);
}
/**
* WikittyI18nImpl :
* @param businessEntityWikitty
*/
public WikittyI18nImpl(BusinessEntityImpl businessEntityWikitty) {
super(businessEntityWikitty.getWikitty());
}
/**
* WikittyAuthorisationImpl :
* @param extension
* @param wikitty
*/
public WikittyI18nImpl(WikittyExtension extension, Wikitty wikitty) {
this(wikitty);
setExtensionForMetaExtension(extension);
}
/**
* On surcharge la methode pour se mettre listener des modifications de
* translation pour pouvoir vider le cache de translation
* @param wikitty
*/
@Override
public void setWikitty(Wikitty wikitty) {
if (this.wikitty != null) {
this.wikitty.removePropertyChangeListener(
FQ_FIELD_WIKITTYI18N_TRANSLATIONS, this);
}
super.setWikitty(wikitty);
if (this.wikitty != null) {
this.wikitty.addPropertyChangeListener(
FQ_FIELD_WIKITTYI18N_TRANSLATIONS, this);
}
}
/**
* Retourne la translation pour un champs donne pour une lang donnee
* @param lang la langue souhaitee (ex: fr)
* @param field le champs souhaite (ex: name)
* @return la traduction
*/
@Override
public String getTranslation(String lang, String field) {
parseAndCacheTranslations();
String result = null;
// If cache is null, no translation is availlable
if (translationsCache != null) {
Map l = translationsCache.get(lang);
if (l != null) {
result = l.get(field);
}
}
if (result == null) {
// no translation for this field, default return field in parameter
result = field;
}
return result;
}
/**
* Modifie la traduction d'un champs
* @param lang
* @param field
* @param trad
*/
@Override
public void setTranslation(String lang, String field, String trad) {
parseAndCacheTranslations();
putInTranslationCache(lang, field, trad);
// on sauve temporairement le cache, car il va etre supprime
// suite au setTranslations, vu qu'il est a jour se serait dommage de
// devoir le reconstruire
Map> tmp = translationsCache;
String trans = convertToString(tmp);
setTranslations(trans);
// remet le cache sauvegarde en place
translationsCache = tmp;
// on indique que la lang a des traductions si besoin
if (getLang() == null || !getLang().contains(lang)) {
addLang(lang);
}
}
/**
* Parse les traductions et le met en cache
*
* Translations est de la forme:
* [fr:"name"="nom","firstname"="prenom"],[en:"name="name","firstname"="firstname"]
*/
protected void parseAndCacheTranslations() {
if (translationsCache == null) {
String trans = getTranslations();
String[] langsFields = StringUtil.split(trans, ",");
for (String langFields : langsFields) {
// suppression des [ ]
langFields = langFields.substring(1, langFields.length() - 1);
int colonPos = langFields.indexOf(":");
// recuperation de la langue
String lang = langFields.substring(0, colonPos);
langFields = langFields.substring(colonPos + 1);
String[] fields = StringUtil.split(langFields, ",");
for (String field : fields) {
String[] fieldNameAndTrad = StringUtil.split(field, "=");
String fieldName = fieldNameAndTrad[0];
String fieldTrad = fieldNameAndTrad[1];
// suppression des "
fieldName = fieldName.substring(1, fieldName.length() - 1);
fieldTrad = fieldTrad.substring(1, fieldTrad.length() - 1);
putInTranslationCache(lang, fieldName, fieldTrad);
}
}
}
}
/**
* met un traduction en plus dans le cache
* @param lang
* @param field
* @param trans
*/
protected void putInTranslationCache(String lang, String field, String trans) {
if (translationsCache == null) {
translationsCache = new HashMap>();
}
Map l = translationsCache.get(lang);
if (l == null) {
l = new HashMap();
translationsCache.put(lang, l);
}
l.put(field, trans);
}
/**
* converti le cache dans une representation string
* @param trans
* @return
*/
protected String convertToString(Map> trans) {
StringBuilder result = new StringBuilder();
for (Map.Entry> l : trans.entrySet()) {
String lang = l.getKey();
result.append("[" + lang + ":");
for(Map.Entry t : l.getValue().entrySet()) {
result.append("\"" + t.getKey() + "\"=\"" + t.getValue() + "\",");
}
// suppression de la derniere ,
if (result.charAt(result.length() - 1) == ',') {
result.deleteCharAt(result.length() - 1);
}
result.append("],");
}
if (result.charAt(result.length() - 1) == ',') {
result.deleteCharAt(result.length() - 1);
}
return result.toString();
}
/**
* Ecoute les events pour devalider le cache
* @param evt
*/
@Override
public void propertyChange(PropertyChangeEvent evt) {
String propName = evt.getPropertyName();
if (FQ_FIELD_WIKITTYI18N_TRANSLATIONS.equals(propName)) {
// la valeur a change on vide le cache
translationsCache = null;
}
}
} //WikittyI18nImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy