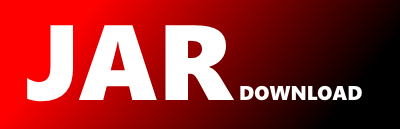
org.nuiton.wikitty.addons.WikittyLabelUtil Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.addons;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.wikitty.query.WikittyQuery;
import org.nuiton.wikitty.query.WikittyQueryMaker;
import org.nuiton.wikitty.query.WikittyQueryResult;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.entities.WikittyLabelHelper;
import org.nuiton.wikitty.entities.WikittyLabelImpl;
import org.nuiton.wikitty.WikittyClient;
import org.nuiton.wikitty.WikittyProxy;
import org.nuiton.wikitty.entities.BusinessEntityImpl;
import org.nuiton.wikitty.entities.WikittyLabel;
import org.nuiton.wikitty.search.Criteria;
import org.nuiton.wikitty.search.PagedResult;
import org.nuiton.wikitty.search.Search;
/**
* Util method to manage Label (add and find)
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class WikittyLabelUtil {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(WikittyLabelUtil.class);
/**
* Add label on this wikitty. You must store your wikitty after
* @param wikitty
* @param label
*/
static public void addLabel(Wikitty wikitty, String label) {
if (!WikittyLabelHelper.hasExtension(wikitty)) {
WikittyLabelHelper.addExtension(wikitty);
}
WikittyLabelHelper.addLabels(wikitty, label);
}
/**
* Add label on this entity. You must store your entiry after
*
* @param entity
* @param label
*/
static public void addLabel(BusinessEntityImpl entity, String label) {
Wikitty w = entity.getWikitty();
addLabel(w, label);
}
/**
* Add new lable to the wikitty object. You must only used this method, if
* you don't have the Wikitty Object because this method restore and save
* version in WikittyService. If you have Wikitty present in your space, your
* wikitty become obsolete and you lose your change :(
*
* If you have wikitty or business entity you must used {@link #}
*
* @param proxy
* @param wikittyId object'id
* @param label label to add
* @deprecated since 3.4 use {@link #addLabel(org.nuiton.wikitty.WikittyClient, java.lang.String, java.lang.String) }
*/
@Deprecated
static public void addLabel(WikittyProxy proxy, String wikittyId, String label) {
Wikitty w = proxy.restore(wikittyId);
WikittyLabelImpl l = new WikittyLabelImpl(w);
l.addLabels(label);
proxy.store(l);
}
/**
* Add new lable to the wikitty object. You must only used this method, if
* you don't have the Wikitty Object because this method restore and save
* version in WikittyService. If you have Wikitty present in your space, your
* wikitty become obsolete and you lose your change :(
*
* If you have wikitty or business entity you must used {@link #}
*
* @param client
* @param wikittyId object'id
* @param label label to add
*/
static public void addLabel(WikittyClient client, String wikittyId, String label) {
Wikitty w = client.restore(wikittyId);
WikittyLabelImpl l = new WikittyLabelImpl(w);
l.addLabels(label);
client.store(l);
}
/**
* Recherche tous les objets qui ont ce label
*
* @param proxy
* @param label
* @param firstIndex
* @param endIndex
* @return
* @deprecated since 3.4 use {@link #findAllByLabel(org.nuiton.wikitty.WikittyClient, java.lang.String, int, int) }
*/
@Deprecated
static public PagedResult findAllByLabel(WikittyProxy proxy,
String label, int firstIndex, int endIndex) {
WikittyLabelImpl l = new WikittyLabelImpl();
l.addLabels(label);
Criteria criteria = Search.query(l.getWikitty()).criteria()
.setFirstIndex(firstIndex).setEndIndex(endIndex);
PagedResult result = proxy.findAllByCriteria(criteria);
return result;
}
/**
* Recherche tous les objets qui ont ce label
*
* @param client
* @param label
* @param first
* @param limit
* @return
*/
static public WikittyQueryResult findAllByLabel(WikittyClient client,
String label, int firstIndex, int limit) {
WikittyQuery query = new WikittyQueryMaker()
.eq(WikittyLabel.ELEMENT_FIELD_WIKITTYLABEL_LABELS, label).end()
.setFirst(firstIndex).setLimit(limit);
WikittyQueryResult result = client.findAllByQuery(Wikitty.class, query);
return result;
}
/**
* Recherche le premier objet qui a ce label
* @param proxy
* @param label
* @return
* @deprecated since 3.4 use {@link #findByLabel(org.nuiton.wikitty.WikittyClient, java.lang.String) }
*/
@Deprecated
static public Wikitty findByLabel(WikittyProxy proxy, String label) {
WikittyLabelImpl l = new WikittyLabelImpl();
l.addLabels(label);
Criteria criteria = Search.query(l.getWikitty()).criteria();
Wikitty result = proxy.findByCriteria(criteria);
return result;
}
/**
* Recherche le premier objet qui a ce label
* @param proxy
* @param label
* @return
*/
static public Wikitty findByLabel(WikittyClient proxy, String label) {
WikittyQuery query = new WikittyQueryMaker()
.eq(WikittyLabel.ELEMENT_FIELD_WIKITTYLABEL_LABELS, label).end();
Wikitty result = proxy.findByQuery(Wikitty.class, query);
return result;
}
/**
* Retrieve all labels applied on a wikitty object.
*
* @param proxy
* @param wikittyId
* @return set of label
* @deprecated since 3.4 use {@link #findAllAppliedLabels(org.nuiton.wikitty.WikittyClient, java.lang.String) }
*/
@Deprecated
static public Set findAllAppliedLabels(WikittyProxy proxy, String wikittyId) {
Wikitty w = proxy.restore(wikittyId);
Set result = WikittyLabelHelper.getLabels(w);
return result;
}
/**
* Retrieve all labels applied on a wikitty object.
*
* @param client
* @param wikittyId
* @return set of label
*/
static public Set findAllAppliedLabels(WikittyClient proxy, String wikittyId) {
Wikitty w = proxy.restore(wikittyId);
Set result = WikittyLabelHelper.getLabels(w);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy