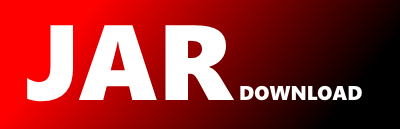
org.nuiton.wikitty.entities.BusinessEntityImpl Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2011 CodeLutin, Benjamin Poussin, Chatellier Eric
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.entities;
import org.nuiton.wikitty.WikittyException;
import org.nuiton.wikitty.WikittyUtil;
import java.beans.PropertyChangeListener;
import java.beans.PropertyChangeSupport;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
/**
*
* @author poussin
* @version $Revision$
*
* Each business object will inherit of this class.
*
* Last update: $Date$
* by : $Author$
*/
public class BusinessEntityImpl implements BusinessEntity {
/** serialVersionUID. */
private static final long serialVersionUID = -4399752739887114180L;
/**
* Property change support.
*
* Warning, this transient field is null after deserialization.
*/
protected transient PropertyChangeSupport propertyChangeSupport;
/**
* Only used by meta-extension, but all extension need it, bacause
* extension can require meta-extension, and then this attribute
* the metaExtension operations target this extension, may be null
*/
protected WikittyExtension extensionForMetaExtension;
protected Wikitty wikitty;
public BusinessEntityImpl(Wikitty wi) {
setWikitty(wi);
}
public BusinessEntityImpl() {
this(new WikittyImpl());
}
protected PropertyChangeSupport getPropertyChangeSupport() {
if (propertyChangeSupport == null) {
propertyChangeSupport = new PropertyChangeSupport(this);
}
return propertyChangeSupport;
}
@Override
public String getWikittyId() {
String result = getWikitty().getWikittyId();
return result;
}
@Override
public String getWikittyVersion() {
String result = getWikitty().getWikittyVersion();
return result;
}
@Override
public void setWikittyVersion(String version) {
getWikitty().setWikittyVersion(version);
}
public void setWikitty(Wikitty wikitty) {
if(wikitty != null) {
for (WikittyExtension ext : getStaticExtensions()) {
if (!wikitty.getExtensions().contains(ext)){
wikitty.addExtension(ext);
}
}
}
this.wikitty = wikitty;
}
public Wikitty getWikitty() {
return wikitty;
}
/**
* this copy used introspection, you can override it in generated class
* to optimize it
* @param source
*/
@Override
public void copyFrom(BusinessEntity source) {
try {
WikittyUtil.copyBean(source, this);
} catch(Exception eee) {
throw new WikittyException(String.format(
"Can't copy source object %s", source), eee);
}
}
@Override
public Collection getExtensionNames() {
Collection result = getWikitty().getExtensionNames();
return result;
}
@Override
public Collection getExtensionFields(String ext) {
Collection result = getWikitty().getExtension(ext).getFieldNames();
return result;
}
//@Override
public FieldType getFieldType(String ext, String fieldName) {
FieldType result = getWikitty().getExtension(ext).getFieldType(fieldName);
return result;
}
@Override
public Object getFieldAsObject(String ext, String fieldName) {
Object result = getWikitty().getFieldAsObject(ext, fieldName);
return result;
}
@Override
public Object getField(String ext, String fieldName) {
return getFieldAsObject(ext, fieldName);
}
@Override
public void setField(String ext, String fieldName, Object value) {
getWikitty().setField(ext, fieldName, value);
}
/**
* Return list of all extension that this entity has by definition
* (design time) and not extension added during execution time
* @return static extension
*/
public Collection getStaticExtensions() {
return Collections.emptyList();
}
@Override
public Collection getStaticExtensionNames() {
ArrayList result = new ArrayList();
for (WikittyExtension ext : getStaticExtensions()) {
result.add(ext.getName());
}
return result;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (!BusinessEntityImpl.class.isAssignableFrom(obj.getClass())) {
return false;
}
BusinessEntityImpl w = (BusinessEntityImpl) obj;
return getWikittyId().equals(w.getWikittyId());
}
@Override
public int hashCode() {
return getWikittyId().hashCode();
}
/*
* @see org.nuiton.wikitty.BusinessEntity#addPropertyChangeListener(java.beans.PropertyChangeListener)
*/
@Override
public void addPropertyChangeListener(PropertyChangeListener listener) {
getPropertyChangeSupport().addPropertyChangeListener(listener);
}
/*
* @see org.nuiton.wikitty.BusinessEntity#removePropertyChangeListener(java.beans.PropertyChangeListener)
*/
@Override
public void removePropertyChangeListener(PropertyChangeListener listener) {
getPropertyChangeSupport().removePropertyChangeListener(listener);
}
/*
* @see org.nuiton.wikitty.BusinessEntity#addPropertyChangeListener(java.lang.String, java.beans.PropertyChangeListener)
*/
@Override
public void addPropertyChangeListener(String propertyName,
PropertyChangeListener listener) {
getPropertyChangeSupport().addPropertyChangeListener(propertyName, listener);
}
/*
* @see org.nuiton.wikitty.BusinessEntity#removePropertyChangeListener(java.lang.String, java.beans.PropertyChangeListener)
*/
@Override
public void removePropertyChangeListener(String propertyName,
PropertyChangeListener listener) {
getPropertyChangeSupport().removePropertyChangeListener(propertyName, listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy