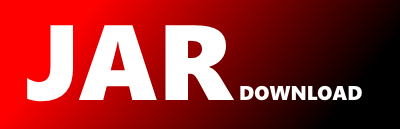
org.nuiton.wikitty.entities.Wikitty Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2011 CodeLutin, Benjamin Poussin, Chatellier Eric
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.entities;
import java.beans.PropertyChangeListener;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Wikitty object, containing data as map.
*
* @author chatellier
* @version $Revision$
*
* Last update : $Date$
* By : $Author$
*/
public interface Wikitty extends Cloneable, Serializable {
/**
* Add property change listener.
*
* @param listener listener to add
*/
void addPropertyChangeListener(PropertyChangeListener listener);
/**
* Remove property change listener.
*
* @param listener listener to remove
*/
void removePropertyChangeListener(PropertyChangeListener listener);
/**
* Add property change listener on property.
*
* @param propertyName property to listen
* @param listener listener to add
*/
void addPropertyChangeListener(String propertyName,
PropertyChangeListener listener);
/**
* Remove property change listener on property.
*
* @param propertyName property to listen
* @param listener listener to remove
*/
void removePropertyChangeListener(String propertyName,
PropertyChangeListener listener);
/**
* Retourne le pattern de preload demande lors du restore ou null si aucun
* preload n'a ete demande.
* @return
*/
String getPreloadPattern();
/**
* Modifie le pattern de preload
* @param preloadPattern un nouveau pattern ou null
*/
void setPreloadPattern(String preloadPattern);
/**
* Retourne tous les patterns de preload, celui demande lors du restore et
* tout ceux defini par le tag value 'preload' sur les extensions. La
* Collection contient une liste de chaine qui n'ont comme separateur que
* des ','. Car chaque separateur ';' donne une entre differente dans la
* collection.
* @return Collection with pattern or empty collection if no pattern
*/
Set getAllPreloadPattern();
/**
* Retourne la map des wikitties precharges, si aucun objet n'est precharge
* alors retourne une map vide. La collection retournee est non modifiable.
* @return Retourne toujours une map au pire elle est vide
*/
Map getPreloaded();
/**
* Modifie la liste des wikitties prechargee
* @param preloaded
*/
void setPreloaded(Map preloaded);
/**
* Ajoute un wikitty comme preloaded dans la liste des wikitties prechargee.
* @param w le wikitty a ajouter
*/
void addPreloaded(Wikitty w);
/**
* Replace all field of current wikitty with field found in w.
* This two wikitty must have same id.
*
* @param w wikitty where we take information
*/
void replaceWith(Wikitty w);
/**
* Replace all field of current wikitty with field found in w.
* This two wikitty must have same id if force is false
* @param w wikitty where we take information
* @param force
*/
void replaceWith(Wikitty w, boolean force);
/**
* Get wikkity id.
*
* @return wikytty id
* @deprecated use getWikittyIdNNN
*/
@Deprecated
String getId();
/**
* Get wikkity id.
*
* @return wikytty id
* @since 3.8
*/
String getWikittyId();
/**
* Return {@code true} is wikitty is deleted.
*
* @return {@code true} is wikitty is deleted
*/
boolean isDeleted();
/**
* Return deletion date.
*
* @return deletion date or {@code null} if not deleted
*/
Date getDeleteDate();
/**
* Set deletion date.
*
* Server only used.
*
* @param date deletion date
*/
void setDeleteDate(Date date);
/**
* Add new extension.
*
* @param ext extension to add
*/
void addExtension(WikittyExtension ext);
/**
* Add multiples extensions.
*
* @param exts extensions to add
*/
void addExtension(Collection exts);
/**
* Remove extension in argument and all dependants extensions.
* Fields of extension is removed too.
* If extension in argument is not found, nothing is done
*
* @param ext extension to remove
* @since 3.9
*/
void removeExtension(String ext);
/**
* Remove extension in argument and all dependants extensions.
* Fields of extension is removed too.
* If extension in argument is not found, nothing is done
*
* @param exts extensions to remove
* @since 3.10
*/
void removeExtensions(Collection exts);
/**
* Check that the wikitty has a metaExtension about a given extension.
*
* @param metaExtensionName the metaExtension to be checked
* @param extensionName an extension already added to the wikitty
* @return {@code true} if current wikitty has meta extension
* @since 2.2.0
*/
boolean hasMetaExtension(String metaExtensionName,
String extensionName);
/**
* Add a meta-extension about the given extension to this wikitty.
*
* @param metaExtension the metaExtension to add
* @param extension an extension already added to the wikitty
* @since 2.1
*/
void addMetaExtension(WikittyExtension metaExtension,
WikittyExtension extension);
/**
* Add a meta-extension on the given extension to this wikitty.
*
* @param metaExtension the metaExtension to add
* @param extensionFqn the name of the extension already added to the wikitty
* @since 2.1
*/
void addMetaExtension(WikittyExtension metaExtension, String extensionFqn);
/**
* Check if current wikitty has extension.
*
* @param extName extension name to check
* @return {@code true} if current wikitty has extension.
*/
boolean hasExtension(String extName);
/**
* Check if current wikitty has requested field.
*
* @param extName extension name
* @param fieldName field name on extension
* @return {@code true} if wikitty has field
*/
boolean hasField(String extName, String fieldName);
/**
* Check if current wikitty has requested fully qualified field.
*
* @param fqfieldName fully qualified field name
* @return {@code true} if wikitty has field
*/
boolean hasField(String fqfieldName);
WikittyExtension getExtension(String ext);
Collection getExtensionNames();
Collection getExtensions();
/**
* Retourne tous les noms de champs pour un nom d'extension donne.
* @param ext le nom de l'extension
* @return la liste des noms de champs
* @since 3.8
*/
Collection getExtensionFields(String ext);
/**
* Recherche toutes les extensions de ce wikitty qui dependent d'une
* extension particuliere
*
* @param ext le nom d'une extension
* @param recursively si la dependance doit aussi etre recherche recursivement
* @return la liste des extensions de ce Wikitty qui depande de l'extension
* dont le nom est passé en parametre (de facon direct ou indirect)
*/
Collection getExtensionDependencies(String ext,
boolean recursively);
/**
* Return field type for the given fieldName.
*
* @param fqfieldName fully qualified fieldName extension.fieldname
* @return field type
*/
FieldType getFieldType(String fqfieldName);
/**
* Return field type for the given fieldName.
*
* @param extName extension name
* @param fieldName field name
* @return field type
*/
FieldType getFieldType(String extName, String fieldName);
/**
* Set field value.
*
* @param ext extension
* @param fieldName field name
* @param value
*/
void setField(String ext, String fieldName, Object value);
/**
* Get field value. if fieldName doesn't exists on this extension an
* exception is throw
*
* @param ext extension name
* @param fieldName field name
* @return
*/
Object getFieldAsObject(String ext, String fieldName);
byte[] getFieldAsBytes(String ext, String fieldName);
boolean getFieldAsBoolean(String ext, String fieldName);
BigDecimal getFieldAsBigDecimal(String ext, String fieldName);
int getFieldAsInt(String ext, String fieldName);
long getFieldAsLong(String ext, String fieldName);
float getFieldAsFloat(String ext, String fieldName);
double getFieldAsDouble(String ext, String fieldName);
String getFieldAsString(String ext, String fieldName);
Date getFieldAsDate(String ext, String fieldName);
/**
* return wikitty id and not wikitty objet because this method can be call
* on server or client side and it's better to keep conversion between id
* and objet to the caller
* @param ext extension name where this field must to be
* @param fieldName the field name
* @return id of wikitty object or null
* @throws org.nuiton.wikitty.WikittyException
*/
String getFieldAsWikitty(String ext, String fieldName);
/**
* return Wikitty for field only if this wikitty is preloaded, null or
* exception otherwize.
* @param extName extension name where this field must to be
* @param fieldName the field name
* @param exceptionIfNotLoaded if true and this field is not preloaded
* an exception is throw, otherwize null is returned. But if field is null
* (never set or set to null) and exceptionIfNotLoaded is true. This method
* must return null and not throw exception.
* @return Wikitty object or null
*/
Wikitty getFieldAsWikitty(String extName, String fieldName, boolean exceptionIfNotLoaded);
/**
* If object is a set, it is automatically transform to list.
* @param
* @param clazz
* @return unmodifiable list
*/
List getFieldAsList(String ext, String fieldName, final Class clazz);
List getFieldAsWikittyList(String ext, String fieldName, boolean exceptionIfNotLoaded);
/**
*
* @param
* @param clazz
* @return unmodifiable list
*/
Set getFieldAsSet(String ext, String fieldName, final Class clazz);
Set getFieldAsWikittySet(String ext, String fieldName, boolean exceptionIfNotLoaded);
void addToField(String ext, String fieldName, Object value);
void removeFromField(String ext, String fieldName, Object value);
void clearField(String ext, String fieldName);
/**
* Add an element in a field
* @param fqFieldName the fully qualified name of a collection field
* @param value the value to be added to the collection
* @since 2.2.0
*/
void addToField(String fqFieldName, Object value);
/**
* Remove an element from a field
* @param fqFieldName the fully qualified name of a collection field
* @param value the value to be removed to the collection
* @since 2.2.0
*/
void removeFromField(String fqFieldName, Object value);
/**
* Clear a field
* @param fqFieldName the fully qualified name of a collection field
* @since 2.2.0
*/
void clearField(String fqFieldName);
/**
* Return only used fieldNames
* @return
*/
Set fieldNames();
/**
* All field value in unmodifiable map
* @return
*/
Map getFieldValue();
/**
* Return all fieldName available in all extension. Field is fq.
* @return
*
* @since 3.1
*/
Set getAllFieldNames();
/**
* get the value of and field given its fqn, if this field doesn't exists
* null is returned
* @param fqFieldName
* @return
*/
Object getFqField(String fqFieldName);
/**
*
* @deprecated use getWikittyVersionNNN
*/
@Deprecated
String getVersion();
/**
* Return wikitty version (x.y).
*
* @return wikitty version
* @since 3.8
*/
String getWikittyVersion();
/**
* Server only used
* @param version
* @deprecated use setWikittyVersionNNN
*/
@Deprecated
void setVersion(String version);
/**
* Server only used
* @param version
* @since 3.8
*/
void setWikittyVersion(String version);
/**
* get the field modified after wikitty was restored
* @return a set of fully qualified field names
* @since 2.2.0
*/
Set getDirty();
/** clear the lists of modified field since last restore. */
void clearDirty();
/** set the value of a field given is fqn.
* @param fieldName fqn (ex: extensionName.fieldName)
* @param value new value
*/
void setFqField(String fieldName, Object value);
boolean isEmpty();
/**
* @see Object#clone()
*/
Wikitty clone() throws CloneNotSupportedException;
/**
* Return String representation of this wikitty with toString specific
* format of extension passed in argument. If this extension doesn't have
* specifique toString, normal toString is called {@link #toString()}
*/
public String toString(String extName);
/**
* Print all field of all extension
*/
public String toStringAllField();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy