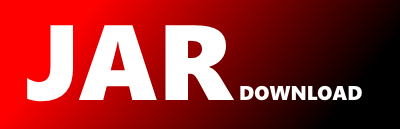
org.nuiton.wikitty.query.ListObjectOrMap Maven / Gradle / Ivy
The newest version!
package org.nuiton.wikitty.query;
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2013 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* Encapsule une liste pour permettre une iteration et une modification facile
* des valeurs de cette listes. Les valeurs peuvent etre dans des maps, il est
* donc penible de parcourrir la liste, puis les maps, puis de modifier les
* valeurs de ces maps. La method {@link iter} retourne un iterator qui permet
* d'avance aussi bien dans la liste si celle-ci ne contient pas de Map ou dans
* les valeurs des Map si la liste est constituee de Map.
* Il est alors possible de recupere la valeur courante, modifier la valeur
* courante ou recuperer une Key qui permettra de mettre a jour l'element
* plus tard. Cette Class est surtout utilise pour la methode
* {@link WikittyClient#castTo}
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class ListObjectOrMap implements List {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(ListObjectOrMap.class);
protected List list;
public ListObjectOrMap() {
this.list = new ArrayList();
}
public ListObjectOrMap(List list) {
this.list = list;
}
@Override
public String toString() {
return list.toString();
}
/**
* Ajoute une nouvelle map dans la liste avec les elements fournis en parametre
* @param key
* @param value
*/
public void add(String key, Object value) {
Map map = new HashMap();
map.put(key, value);
add(map);
}
public List getList() {
return list;
}
public ListObjectOrMapIterator iter() {
return new ListObjectOrMapIterator();
}
public interface Key {
public Object getValue();
public void setValue(Object v);
public void remove();
}
class KeyMap implements Key {
protected Map map;
protected Object key;
public KeyMap(Map map, Object key) {
this.map = map;
this.key = key;
}
@Override
public Object getValue() {
return map.get(key);
}
@Override
public void setValue(Object v) {
map.put(key, v);
}
@Override
public void remove() {
map.remove(key);
}
}
class KeyList implements Key {
protected List list;
protected int i;
public KeyList(List list, int i) {
this.list = list;
this.i = i;
}
@Override
public Object getValue() {
return list.get(i);
}
@Override
public void setValue(Object v) {
list.set(i, v);
}
@Override
public void remove() {
list.remove(i);
}
}
public class ListObjectOrMapIterator implements Iterator {
protected boolean isMap;
protected List list;
protected Object[] listEntry;
protected int listIndex;
protected Map map;
protected Map.Entry[] mapEntry;
protected int mapIndex;
protected Object next;
protected Key key;
public ListObjectOrMapIterator() {
list = ListObjectOrMap.this.list;
listEntry = list.toArray();
listIndex = 0;
}
@Override
public boolean hasNext() {
boolean result = false;
if (mapEntry != null && mapIndex < mapEntry.length) {
result = true;
} else {
while (!result && listIndex < listEntry.length) {
next = listEntry[listIndex++];
isMap = next instanceof Map;
if (isMap) {
map = (Map)next;
Set entry = map.entrySet();
if (!entry.isEmpty()) {
mapEntry = entry.toArray(new Map.Entry[entry.size()]);
mapIndex = 0;
result = true;
}
} else {
result = true;
}
}
}
return result;
}
@Override
public Object next() {
if (isMap) {
Map.Entry e = mapEntry[mapIndex++];
key = new KeyMap(map, e.getKey());
return e.getValue();
} else {
key = new KeyList(list, listIndex-1);
return next;
}
}
public Key nextKey() {
next();
return key;
}
public Key getKey() {
return key;
}
public void setValue(Object v) {
key.setValue(v);
}
@Override
public void remove() {
key.remove();
}
}
@Override
public int size() {
return list.size();
}
@Override
public boolean isEmpty() {
return list.isEmpty();
}
@Override
public boolean contains(Object o) {
return list.contains(o);
}
@Override
public Iterator iterator() {
return list.iterator();
}
@Override
public Object[] toArray() {
return list.toArray();
}
@Override
public Object[] toArray(Object[] a) {
return list.toArray(a);
}
@Override
public boolean add(Object e) {
return list.add(e);
}
@Override
public boolean remove(Object o) {
return list.remove(o);
}
@Override
public boolean containsAll(Collection c) {
return list.containsAll(c);
}
@Override
public boolean addAll(Collection c) {
return list.addAll(c);
}
@Override
public boolean addAll(int index, Collection c) {
return list.addAll(index, c);
}
@Override
public boolean removeAll(Collection c) {
return list.removeAll(c);
}
@Override
public boolean retainAll(Collection c) {
return list.retainAll(c);
}
@Override
public void clear() {
list.clear();
}
@Override
public Object get(int index) {
return list.get(index);
}
@Override
public Object set(int index, Object element) {
return list.set(index, element);
}
@Override
public void add(int index, Object element) {
list.add(index, element);
}
@Override
public Object remove(int index) {
return list.remove(index);
}
@Override
public int indexOf(Object o) {
return list.indexOf(o);
}
@Override
public int lastIndexOf(Object o) {
return list.lastIndexOf(o);
}
@Override
public ListIterator listIterator() {
return list.listIterator();
}
@Override
public ListIterator listIterator(int index) {
return list.listIterator(index);
}
@Override
public List subList(int fromIndex, int toIndex) {
return list.subList(fromIndex, toIndex);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy