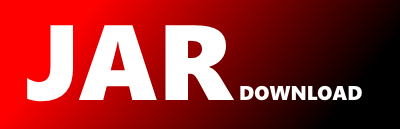
org.nuiton.wikitty.query.conditions.AbstractCondition Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2012 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.query.conditions;
import org.nuiton.wikitty.WikittyException;
import org.nuiton.wikitty.query.WikittyQueryVisitor;
import org.nuiton.wikitty.query.WikittyQueryVisitorCopy;
import org.nuiton.wikitty.query.WikittyQueryVisitorToString;
/**
* This element is a symbolic interface implemented by all operators used to
* request contents (And, Or, Not, Equals, NotEquals, EndsWith, ...).
*
* @author poussin
* @version $Revision$
* @since 3.3
*
* Last update: $Date$
* by : $Author$
*/
public abstract class AbstractCondition implements Condition {
// serialVersionUID is used for serialization.
private static final long serialVersionUID = 1L;
@Override
public Condition copy() {
WikittyQueryVisitorCopy v = new WikittyQueryVisitorCopy();
accept(v);
return v.getCondition();
}
/**
* For non terminal condition this method add subCondition.
* For terminal this method must return an exception
*
* When you override this method, you must call {@link #checkAddCondition}
* in first
*
* @param c
*/
@Override
public Condition addCondition(Condition c) {
throw new WikittyException(String.format(
"Add condition is not allowed in this condition type '%s'",
getClass().getSimpleName()));
}
@Override
public void accept(WikittyQueryVisitor visitor) {
visitor.visit(this);
}
/**
* Equality test based on class equality
*
* @param other Value to compare
*/
@Override
public boolean equals(Object other) {
boolean result;
if (other == null) {
result = false;
} else if (this == other) {
result = true;
}else if (this.getClass().equals(other.getClass())) {
result = equalsDeep(other);
} else {
return false;
}
return result;
}
/**
* Sub class must override this method to check if internal state is same
* in two object (this and other)
*
* @param other other parameter is same type that this object
* @return true if other and this are equals
*/
abstract boolean equalsDeep(Object other);
@Override
public int hashCode() {
// equals use objects that are not constant through time
// then, unable to create hashCode from those objects
// returning a constant hash-code
return this.getClass().hashCode();
}
@Override
public String toString() {
WikittyQueryVisitorToString v = new WikittyQueryVisitorToString();
accept(v);
String result = v.getText();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy