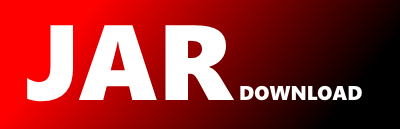
org.nuiton.wikitty.query.conditions.TerminalNaryOperator Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2012 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.query.conditions;
import org.nuiton.wikitty.entities.Element;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.apache.commons.lang3.ClassUtils;
import org.apache.commons.lang3.ObjectUtils;
import org.nuiton.wikitty.WikittyException;
import org.nuiton.wikitty.query.WikittyQueryVisitor;
/**
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class TerminalNaryOperator extends TerminalOperator {
// serialVersionUID is used for serialization.
private static final long serialVersionUID = 1L;
protected Element element;
protected List values;
/**
* Constructor with all parameters initialized
*
* @param element
*/
public TerminalNaryOperator(Element element) {
this(element, (Collection)null);
}
/**
* Constructor with all parameters initialized
*
* @param element
* @param values value is internaly copied to prevent external modification
*/
public TerminalNaryOperator(Element element, Collection values) {
this.element = element;
if (values != null) {
this.values = new ArrayList(values);
}
}
@Override
public boolean waitCondition() {
return true;
}
@Override
public Condition addCondition(Condition c) {
if (c instanceof ConditionValue) {
getValues().add((ConditionValue)c);
} else {
throw new WikittyException(String.format(
"Only ConditionValue can be add to %s, but you try to add: %s",
this.getClass().getSimpleName(),
ClassUtils.getShortCanonicalName(c, "null")));
}
return this;
}
@Override
public void accept(WikittyQueryVisitor visitor) {
boolean walk = visitor.visitEnter(this);
if (walk && values != null) {
boolean notFirst = false;
for (Condition r : values) {
if (notFirst) {
walk = visitor.visitMiddle(this);
if (!walk) {
// le visiteur demande l'arret de la visite
break;
}
} else {
notFirst = true;
}
r.accept(visitor);
}
}
visitor.visitLeave(this, walk);
}
public Element getElement() {
return element;
}
public List getValues() {
if (values == null) {
values = new ArrayList();
}
return values;
}
@Override
boolean equalsDeep(Object other) {
TerminalNaryOperator op = (TerminalNaryOperator)other;
boolean result = ObjectUtils.equals(this.getElement(), op.getElement())
&& ObjectUtils.equals(this.getValues(), op.getValues());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy