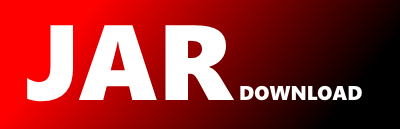
org.nuiton.wikitty.query.function.FunctionYear Maven / Gradle / Ivy
The newest version!
package org.nuiton.wikitty.query.function;
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2013 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.time.DateUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.util.DateUtil;
import org.nuiton.wikitty.WikittyUtil;
import org.nuiton.wikitty.entities.Element;
import org.nuiton.wikitty.query.ListObjectOrMap;
import org.nuiton.wikitty.query.WikittyQuery;
/**
* Permet de recuperer l'annee d'un champs DATE, les Maps retournees contiennent
* seulement un champs
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class FunctionYear extends WikittyQueryFunction {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(FunctionYear.class);
public FunctionYear(String name) {
super("year", name, null);
}
public FunctionYear(String name, WikittyQueryFunction arg) {
super("year", name, Collections.singletonList(arg));
}
public FunctionYear(String methodName, String name, List args) {
super(methodName, name, args);
if (args.size() != 1) {
throw new IllegalArgumentException("Year accept only one argument");
}
}
@Override
public int getNumArg() {
return 1;
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy