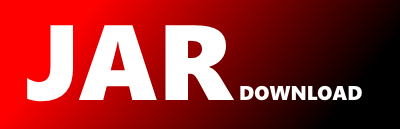
org.nuiton.wikitty.search.PagedResult Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2011 CodeLutin, Benjamin Poussin, Chatellier Eric
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.search;
import java.util.Iterator;
import static org.nuiton.i18n.I18n.t;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.wikitty.entities.BusinessEntityImpl;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.WikittyException;
import org.nuiton.wikitty.entities.WikittyExtension;
import org.nuiton.wikitty.WikittyProxy;
import org.nuiton.wikitty.WikittyService;
import org.nuiton.wikitty.WikittyUtil;
/**
* Paged result containing result collection and facet topics.
*
* @param paged result value type
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
* @deprecated since 3.3 use new query api {@link org.nuiton.wikitty.query.WikittyQuery}
*/
@Deprecated
public class PagedResult implements Serializable, Iterable {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(PagedResult.class);
/** serialVersionUID. */
private static final long serialVersionUID = 8518774558223121855L;
/** nom du critere qui a ete uitilise (peut-etre null) si le critete n'avait pas de nom */
protected String criteriaName;
/** indice element in global search result */
protected int firstIndice;
/** total number of result if we call the query for all possible result */
protected int numFound;
/** query really executed */
protected String queryString;
/** list of result in the wanted interval */
protected List results;
/** facet asked or null if no facet */
protected Map> facets;
/** facet asked of null if no facet, FacetTopic are put in map with key topic name,
* To use this variable, you must used getter, because, this variable is
* lazy loaded from facets variable.
*/
protected Map> facetsAsMap = null;
/**
* Init paged result.
*
* we don't initialize securityToken
*
* @param firstIndice indice element in global search result
* @param numFound total number of result if we call the query for all possible result
* @param queryString query really executed
* @param facets facet asked or null if no facet
* @param results list of result in the wanted interval
*/
public PagedResult(String criteriaName,
int firstIndice, int numFound, String queryString,
Map> facets, List results) {
this.criteriaName = criteriaName;
this.firstIndice = firstIndice;
this.numFound = numFound;
this.queryString = queryString;
this.facets = facets;
this.results = Collections.unmodifiableList(results);
}
/**
* Call {@link #cast(WikittyProxy, Class, boolean)} with
* autoconvert = true
*
* @param proxy used to retrieve securityToken and WikittyService
* @param clazz target PagedResult type
* @return new PagedResult, this result can have less elements that original
* for some reason (security, ...)
*/
public PagedResult cast(
WikittyProxy proxy, Class clazz) {
return cast(proxy, clazz, true);
}
/**
* Convert all result to the wikitty type and return new PagedResult with
* this new result list.
*
* @param securityToken security token
* @param ws wikitty service
*
* @return new PagedResult, this result can have less elements that original
* for some reason (security, ...)
*/
public PagedResult cast(String securityToken, WikittyService ws) {
List castedResult;
if (results.size() == 0) {
castedResult = new ArrayList();
} else {
if (results.get(0) instanceof String) {
// le pagedresult courant contient des Ids
// Si ce n'est pas le cas, ca veut dire que le developpeur utilisant
// ce PagedResult ne sait pas ce qu'il fait :)
List ids = (List) results;
castedResult = ws.restore(securityToken, ids);
} else {
throw new ClassCastException("PagedResult don't contains" +
" wikitty String id but " + results.get(0).getClass());
}
}
PagedResult result = new PagedResult(criteriaName,
firstIndice, numFound, queryString, facets, castedResult);
return result;
}
/**
* Convert all result to the wanted type and return new PagedResult with
* this new result list. If some result don't have the right extension (clazz)
* this extension is automatically added if autoconvert is true. Else
* an exception is thrown when result without extension is found.
*
* When you used autoconvert = false, you have a potentially problem when
* you have modified a BusinessEntity to have new extension and all your
* wikitty object are not uptodate in database.
*
* @param class to cast into
* @param proxy used to retrieve securityToken and WikittyService
* @param autoconvert if autoconvert is false and object don't all needed
* extension, object is not put in the result
* @return new PagedResult, this result can have less elements that original
* for some reason (security, ...)
*/
public PagedResult cast(
WikittyProxy proxy, Class clazz, boolean autoconvert) {
List castedResult;
if (results.size() > 0 && results.get(0) instanceof String) {
// le pagedresult courant contient des Ids
// Si ce n'est pas le cas, ca veut dire que le developpeur utilisant
// ce PagedResult ne sait pas ce qu'il fait :)
List ids = (List)results;
castedResult = proxy.restore(clazz, ids, !autoconvert);
} else {
castedResult = new ArrayList(results.size());
E sample = WikittyUtil.newInstance(clazz);
Collection wantedExtension = sample.getStaticExtensions();
for (T t : results) {
if (t == null) {
castedResult.add(null);
} else {
Wikitty w = null;
if (t instanceof Wikitty) {
w = (Wikitty) t;
} else if (t instanceof BusinessEntityImpl) {
w = ((BusinessEntityImpl) t).getWikitty();
} else {
throw new WikittyException(String.format(
"Illegal object result class '%s' can't convert it to class '%s'",
t.getClass().getName(), clazz.getName()));
}
Collection wikittyExtension = w.getExtensions();
if (autoconvert || wikittyExtension.containsAll(wantedExtension)) {
E e = WikittyUtil.newInstance(proxy.getSecurityToken(),
proxy.getWikittyService(), clazz, (Wikitty) t);
castedResult.add(e);
} else {
// silently pass current object, this object is not put
// in result
if (log.isDebugEnabled()) {
log.debug(t(
"Illegal object result class '%s' can't convert it to '%s'" +
"there is no same extension %s != %s",
t.getClass().getName(), clazz.getName(),
wikittyExtension, wantedExtension));
}
}
}
}
}
PagedResult result = new PagedResult(criteriaName,
firstIndice, numFound, queryString, facets, castedResult);
return result;
}
public String getCriteriaName() {
return criteriaName;
}
public int getFirstIndice() {
return firstIndice;
}
public int getNumFound() {
return numFound;
}
public String getQueryString() {
return queryString;
}
/**
* Return name of all facet used in query.
*
* @return result's facets names
*/
public Collection getFacetNames() {
Collection result = facets.keySet();
return result;
}
/**
* Return all topic for the specified facet.
*
* @param facetName name of the wanted facet
* @return facet's topics
*/
public List getTopic(String facetName) {
List result = facets.get(facetName);
return result;
}
/**
* Return topic for the specified facet and topic name.
*
* @param facetName name of the wanted facet
* @param topicName name of the wanted topic
* @return topic
*/
public FacetTopic getTopic(String facetName, String topicName) {
FacetTopic result = getFacetsAsMap().get(facetName).get(topicName);
return result;
}
/**
* Return topic count for the specified facet and topic name. If facet or
* topic don't exist, return 0.
*
* @param facetName name of the wanted facet
* @param topicName name of the wanted topic
* @return topic count or 0
*/
public int getTopicCount(String facetName, String topicName) {
int result = 0;
if (getFacetsAsMap() != null) {
Map topics = getFacetsAsMap().get(facetName);
if (topics != null) {
FacetTopic topic = topics.get(topicName);
if (topic != null) {
result = topic.getCount();
}
}
}
return result;
}
/**
* Get map represent facets.
*
* @return all facets
*/
public Map> getFacets() {
return facets;
}
public Map> getFacetsAsMap() {
if (facetsAsMap == null && facets != null) {
// use local variable to prevent multi-thread problem (multiple add)
Map> localFacetsAsMap =
new HashMap>();
for (Map.Entry> e : getFacets().entrySet()) {
Map topics = new HashMap();
localFacetsAsMap.put(e.getKey(), topics);
for (FacetTopic t : e.getValue()) {
topics.put(t.getTopicName(), t);
}
}
facetsAsMap = localFacetsAsMap;
}
return facetsAsMap;
}
/**
* Return the first element in result
*
* Can throw an exception if no element available
* @return first element
*/
public T getFirst() {
T result = get(0);
return result;
}
/**
* Return element at index.
*
* @param i index
* @return element at index
*/
public T get(int i) {
T result = results.get(i);
return result;
}
/**
* Return unmodifiable list of all result.
*
* @return all results
*/
public List getAll() {
return results;
}
/**
* Return the number of result in this object.
*
* @return result number
*/
public int size() {
int result = results.size();
return result;
}
/**
* Iterate on result, same as getAll().iterator().
* @return
*/
public Iterator iterator() {
Iterator result = getAll().iterator();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy