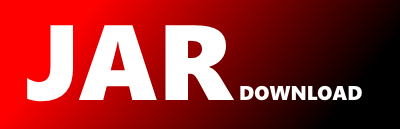
org.nuiton.wikitty.search.RestrictionHelper Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.search;
import org.nuiton.wikitty.search.operators.False;
import org.nuiton.wikitty.search.operators.Like;
import org.nuiton.wikitty.search.operators.RestrictionName;
import org.nuiton.wikitty.search.operators.Restriction;
import org.nuiton.wikitty.search.operators.StartsWith;
import org.nuiton.wikitty.search.operators.Not;
import org.nuiton.wikitty.search.operators.EndsWith;
import org.nuiton.wikitty.search.operators.And;
import org.nuiton.wikitty.search.operators.Or;
import org.nuiton.wikitty.search.operators.Less;
import org.nuiton.wikitty.search.operators.Greater;
import org.nuiton.wikitty.search.operators.GreaterOrEqual;
import org.nuiton.wikitty.search.operators.NotEquals;
import org.nuiton.wikitty.search.operators.LessOrEqual;
import org.nuiton.wikitty.search.operators.Keyword;
import org.nuiton.wikitty.search.operators.Between;
import org.nuiton.wikitty.search.operators.Null;
import org.nuiton.wikitty.search.operators.Contains;
import org.nuiton.wikitty.search.operators.Equals;
import org.nuiton.wikitty.search.operators.Element;
import java.text.SimpleDateFormat;
import java.util.LinkedList;
import java.util.List;
import java.util.Locale;
import java.util.TimeZone;
import org.nuiton.wikitty.search.operators.In;
import org.nuiton.wikitty.search.operators.True;
import org.nuiton.wikitty.search.operators.Unlike;
/**
* @author "Nicolas Chapurlat"
*
* This class allow you to simply create restriction.
*
* Examples :
*
* RestrictionHelper.and(
* RestrictionHelper.eq(
* new ElementDto(ElementName.CONTENT_DEFINITION, OperandName.ID, OperandType.ID), "myContentDefId"),
* RestrictionHelper.between(
* new ElementDto(ElementName.CONTENT, OperandName.CREATION_DATE, OperandType.DATE),
* RestrictionHelper.DATE_FORMAT.format (new Date(2008,1,25)),
* RestrictionHelper.DATE_FORMAT.format (new Date(2008,6,15))))
* );
*
*
* example 2 : I search all content witch content definition id is
* "myContentDefId" and attribute def 'ref' witch id is ATT_REF_ID is not equals
* to "REF1234567890"
*
* RestrictionHelper.and(
* RestrictionHelper.eq(
* new ElementDto(ElementName.CONTENT_DEFINITION, OperandName.ID, OperandType.ID), "myContentDefId"),
* RestrictionHelper.neq(
* new ElementDto(ElementName.ATTRIBUTE, "ATT_REF_ID", OperandType.STRING), "REF1234567890"))
* );
*
* @deprecated since 3.3 use new query api {@link org.nuiton.wikitty.query.WikittyQuery}
*/
@Deprecated
public class RestrictionHelper {
public static Restriction eq(Element element, String value) {
Equals eq = new Equals();
eq.setName(RestrictionName.EQUALS);
eq.setElement(element);
eq.setValue(value);
return eq;
}
public static Restriction neq(Element element, String value) {
NotEquals neq = new NotEquals();
neq.setName(RestrictionName.NOT_EQUALS);
neq.setElement(element);
neq.setValue(value);
return neq;
}
public static Restriction less(Element element, String value) {
Less less = new Less();
less.setName(RestrictionName.LESS);
less.setElement(element);
less.setValue(value);
return less;
}
public static Restriction lessEq(Element element, String value) {
LessOrEqual lessEq = new LessOrEqual();
lessEq.setName(RestrictionName.LESS_OR_EQUAL);
lessEq.setElement(element);
lessEq.setValue(value);
return lessEq;
}
public static Restriction great(Element element, String value) {
Greater great = new Greater();
great.setName(RestrictionName.GREATER);
great.setElement(element);
great.setValue(value);
return great;
}
public static Restriction greatEq(Element element, String value) {
GreaterOrEqual greatEq = new GreaterOrEqual();
greatEq.setName(RestrictionName.GREATER_OR_EQUAL);
greatEq.setElement(element);
greatEq.setValue(value);
return greatEq;
}
public static Restriction start(Element element, String value) {
StartsWith start = new StartsWith();
start.setName(RestrictionName.STARTS_WITH);
start.setElement(element);
start.setValue(value);
return start;
}
public static Restriction end(Element element, String value) {
EndsWith end = new EndsWith();
end.setName(RestrictionName.ENDS_WITH);
end.setElement(element);
end.setValue(value);
return end;
}
public static Restriction between(Element element, String min,
String max) {
Between between = new Between(element, min, max);
between.setName(RestrictionName.BETWEEN);
return between;
}
public static Restriction contains(Element element, String value1,
String... otherValues) {
List values = new LinkedList();
values.add(value1);
for (String val : otherValues) {
values.add(val);
}
return contains(element, values);
}
public static Restriction contains(Element element,
List values) {
Contains contains = new Contains(element, values);
contains.setName(RestrictionName.CONTAINS);
return contains;
}
public static Restriction in(Element element, String value1,
String... otherValues) {
List values = new LinkedList();
values.add(value1);
for (String val : otherValues) {
values.add(val);
}
return in(element, values);
}
public static Restriction in(Element element,
List values) {
In in = new In(element, values);
in.setName(RestrictionName.IN);
return in;
}
public static Restriction not(Restriction restriction) {
Not not = new Not(restriction);
not.setName(RestrictionName.NOT);
return not;
}
public static Restriction and(Restriction restriction1,
Restriction restriction2, Restriction... otherRestrictions) {
List restrictions = new LinkedList();
restrictions.add(restriction1);
restrictions.add(restriction2);
for (Restriction rest : otherRestrictions) {
restrictions.add(rest);
}
return and(restrictions);
}
public static And and(List restrictions) {
And and = new And(restrictions);
and.setName(RestrictionName.AND);
return and;
}
public static Or or(Restriction restriction1,
Restriction restriction2, Restriction... otherRestrictions) {
List restrictions = new LinkedList();
restrictions.add(restriction1);
restrictions.add(restriction2);
for (Restriction rest : otherRestrictions) {
restrictions.add(rest);
}
return or(restrictions);
}
public static Or or(List restrictions) {
Or or = new Or(restrictions);
or.setName(RestrictionName.OR);
return or;
}
public static True rTrue() {
True rTrue = new True();
rTrue.setName(RestrictionName.TRUE);
return rTrue;
}
public static False rFalse() {
False rFalse = new False();
rFalse.setName(RestrictionName.FALSE);
return rFalse;
}
public static Keyword keyword(String value) {
Keyword keyword = new Keyword();
keyword.setName(RestrictionName.KEYWORD);
keyword.setValue(value);
return keyword;
}
public static Null isNull(String fieldName) {
Null isNull = new Null();
isNull.setName(RestrictionName.IS_NULL);
isNull.setFieldName(fieldName);
return isNull;
}
public static Null isNotNull(String fieldName) {
Null isNotNull = new Null();
isNotNull.setName(RestrictionName.IS_NOT_NULL);
isNotNull.setFieldName(fieldName);
return isNotNull;
}
public static Restriction like(Element element, String value, Like.SearchAs searchAs) {
Like like = new Like();
like.setName(RestrictionName.LIKE);
like.setElement(element);
like.setValue(value);
like.setSearchAs(searchAs);
return like;
}
public static Restriction unlike(Element element, String value, Like.SearchAs searchAs) {
Unlike unlike = new Unlike();
unlike.setName(RestrictionName.UNLIKE);
unlike.setElement(element);
unlike.setValue(value);
unlike.setSearchAs(searchAs);
return unlike;
}
static ThreadLocal myFormats = new ThreadLocal() {
@Override
protected SimpleDateFormat initialValue() {
SimpleDateFormat ret = new SimpleDateFormat(
"yyyy-MM-dd'T'00:00:00.000'Z/DAY'", Locale.US);
ret.setTimeZone(TimeZone.getTimeZone("UTC"));
return ret;
}
};
public static SimpleDateFormat getDateFormat() {
return myFormats.get();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy