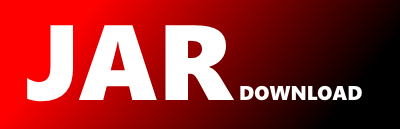
org.nuiton.wikitty.search.TreeNodeResult Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2011 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.search;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.List;
import javax.swing.tree.DefaultMutableTreeNode;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* L'iteration se fait en profondeur
*
* @author poussin
* @version $Revision$
* @since 3.1
*
* Last update: $Date$
* by : $Author$
* @deprecated since 3.3 use new query api {@link org.nuiton.wikitty.query.WikittyQuery}
*/
@Deprecated
public class TreeNodeResult extends DefaultMutableTreeNode implements Iterable> {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(TreeNodeResult.class);
private static final long serialVersionUID = 31L;
/** optional user object */
protected T object;
/**
* Visitor for TreeNodeResult
* @param
*/
static public interface Visitor {
/**
*
* @param node node to visit
* @return if true visit this element
*/
public boolean visitEnter(TreeNodeResult node);
/**
*
* @param node node visited
* @return if true visit next element
*/
public boolean visitLeave(TreeNodeResult node);
}
protected int attCount;
/**
*
* @param object L'id, le wikitty ou le BusinessEntity suivant le type de T
* @param attCount le nombre d'attachment pour ce noeud (avec les sous noeud)
*/
public TreeNodeResult(T object, int attCount) {
this(object);
this.attCount = attCount;
}
/**
* sletellier 20110516 : override all methods using userObject,
* because this one is transient and getter and setters are not used
*/
/**
* Creates a tree node with no parent, no children, but which allows
* children, and initializes it with the specified user object.
*
* @param userObject an Object provided by the user that constitutes
* the node's data
*/
public TreeNodeResult(T userObject) {
this(userObject, true);
}
/**
* Creates a tree node with no parent, no children, initialized with
* the specified user object, and that allows children only if
* specified.
*
* @param userObject an Object provided by the user that constitutes
* the node's data
* @param allowsChildren if true, the node is allowed to have child
* nodes -- otherwise, it is always a leaf node
*/
public TreeNodeResult(T userObject, boolean allowsChildren) {
super(userObject, allowsChildren);
this.object = userObject;
}
/**
* Sets the user object for this node to userObject
.
*
* @param userObject the Object that constitutes this node's
* user-specified data
* @see #getUserObject
* @see #toString
*/
public void setUserObject(T userObject) {
super.setUserObject(userObject);
this.object = userObject;
}
/**
* Returns this node's user object.
*
* @return the Object stored at this node by the user
* @see #toString
*/
@Override
public T getUserObject() {
return object;
}
/**
* Returns the result of sending toString()
to this node's
* user object, or null if this node has no user object.
*
* @see #getUserObject
*/
@Override
public String toString() {
T userObject = getUserObject();
if (userObject == null) {
return null;
} else {
return userObject.toString();
}
}
/**
* Visite en profondeur de l'arbre, il est possible d'arreter la visite
* soit en entrant dans le noeud soit en sortant du noeud, si respectivement
* visitEnter ou visitLeave retourne false.
*
* @param visitor
*/
public boolean acceptVisitor(Visitor visitor) {
if (visitor.visitEnter(this)) {
for (Enumeration e = children(); e.hasMoreElements();) {
TreeNodeResult child = (TreeNodeResult) e.nextElement();
if (!child.acceptVisitor(visitor)) {
break;
}
}
}
boolean result = visitor.visitLeave(this);
return result;
}
/**
* Get direct children of this node
*
* @return
*/
public List> getChildren() {
List> result;
if (children == null) {
result = Collections.emptyList();
} else {
result = Collections.unmodifiableList(
new ArrayList>(children));
}
return result;
}
/**
* Iterate on all children or sub-children, in depth first
* @return
*/
@Override
public Iterator> iterator() {
Iterator> result = new Iterator>() {
protected Enumeration enumDepth = TreeNodeResult.this.depthFirstEnumeration();
@Override
public boolean hasNext() {
return enumDepth.hasMoreElements();
}
@Override
public TreeNodeResult next() {
TreeNodeResult result = (TreeNodeResult)enumDepth.nextElement();
return result;
}
@Override
public void remove() {
throw new UnsupportedOperationException("Not supported yet.");
}
};
return result;
}
/**
* Retourne l'objet associe avec ce noeud (id, wikitty ou BusinessEntity)
* @return l'objet associe avec ce noeud (id, wikitty ou BusinessEntity)
*/
public T getObject() {
return getUserObject();
}
/**
* Return TreeNodeResult where object in TreeNodeResult equals child in
* parameter
* @param child
* @return
*/
public TreeNodeResult getChild(T child) {
TreeNodeResult result = null;
if (child != null) {
for (Enumeration e = children(); e.hasMoreElements();) {
TreeNodeResult r = (TreeNodeResult) e.nextElement();
if (child.equals(r.getObject())) {
result = r;
break;
}
}
}
return result;
}
/**
* Retourn le nombre d'attachment pour ce noeud (avec les sous noeud)
* @return
*/
public int getAttCount() {
return attCount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy