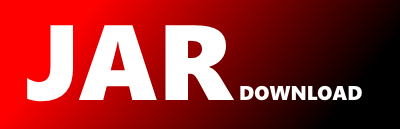
org.nuiton.wikitty.services.WikittyCacheSimple Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.services;
import java.util.Collections;
import org.nuiton.wikitty.entities.Wikitty;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.collections.map.ReferenceMap;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.config.ApplicationConfig;
import org.nuiton.wikitty.WikittyConfig;
import org.nuiton.wikitty.WikittyConfigOption;
import org.nuiton.wikitty.entities.WikittyExtension;
/**
* Cette classe sert a introduire du cache dans wikitty. Elle sert a centraliser
* tous les appels au cache pour pouvoir simplement changer de librairie de
* cache si necessaire, meme si pour l'instant on s'appuie le JDK
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class WikittyCacheSimple implements WikittyCache {
static private Log log = LogFactory.getLog(WikittyCacheSimple.class);
protected Set priorityExtensions = new HashSet();
/**
* cache ou sont stocke les objects qui doivent rester le plus possible
* en memoire.
* ATTENTION: ce ne sont que des references HARD, donc il ne faut pas
* qu'il y ait trop d'objet prioritaire, sinon l'application risque de
* manquer de memoire
* keys are wikitty ids
*/
protected Map priorityCache = new HashMap();
/**
* cache pour les autres wikitties
* keys are wikitty ids
*/
protected Map wikittyCache;
protected Map extensionCache;
/**
*
* @param config not used currently but necessary in futur to configure the cache
* Create a soft cache.
*/
public WikittyCacheSimple(ApplicationConfig config) {
List priorityExtensions = config.getOptionAsList(
WikittyConfigOption.WIKITTY_CACHE_PRIORITY_EXTENSIONS.getKey()).getOption();
this.priorityExtensions.addAll(priorityExtensions);
String refType = config.getOption(
WikittyConfigOption.WIKITTY_CACHE_SIMPLE_REFERENCE_TYPE.getKey());
if ("soft".equalsIgnoreCase(refType)) {
wikittyCache = new ReferenceMap(ReferenceMap.HARD, ReferenceMap.SOFT);
extensionCache = new ReferenceMap(ReferenceMap.HARD, ReferenceMap.SOFT);
} else {
// HARD reference use normal map
wikittyCache = new HashMap();
extensionCache = new HashMap();
}
}
@Override
public boolean existsWikitty(String id) {
Object o = priorityCache.get(id);
boolean result = (o != null);
if (!result) {
o = wikittyCache.get(id);
result = (o != null);
}
return result;
}
/**
* Return wikitty object if is in the cache, null otherwize.
*
* @param id
* @return wikitty object or null
*/
@Override
public Wikitty getWikitty(String id) {
Wikitty result = (Wikitty)priorityCache.get(id);
if (result == null) {
result = (Wikitty) wikittyCache.get(id);
}
return result;
}
/**
* Only realy put wikitty in cache, if not in cache or version is newer than
* one in cache
* @param e
*/
@Override
public void putWikitty(Wikitty e) {
if (e != null) {
if (Collections.disjoint(priorityExtensions, e.getExtensionNames())) {
// le wikitty ne contient pas d'extension prioritaire
// on le met dans le cache commun
wikittyCache.put(e.getWikittyId(), e);
} else {
priorityCache.put(e.getWikittyId(), e);
}
}
}
/**
* Remove wikitty from cache.
*
* @param id wikitty id to remove
*/
@Override
public void removeWikitty(String id) {
priorityCache.remove(id);
wikittyCache.remove(id);
}
/**
* Clear all cache.
*/
@Override
public void clearWikitty() {
priorityCache.clear();
wikittyCache.clear();
}
@Override
public boolean existsExtension(String id) {
Object o = extensionCache.get(id);
boolean result = (o != null);
return result;
}
@Override
public WikittyExtension getExtension(String id) {
WikittyExtension result = (WikittyExtension)extensionCache.get(id);
return result;
}
@Override
public void putExtension(WikittyExtension e) {
if (e != null) {
extensionCache.put(e.getId(), e);
}
}
@Override
public void removeExtension(String id) {
extensionCache.remove(id);
}
@Override
public void clearExtension() {
extensionCache.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy