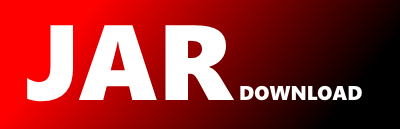
org.nuiton.wikitty.services.WikittyEvent Maven / Gradle / Ivy
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.services;
import com.thoughtworks.xstream.XStream;
import java.util.Date;
import java.util.EnumSet;
import java.util.EventObject;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import org.nuiton.wikitty.WikittyService;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.entities.WikittyExtension;
/**
* Wikitty service event.
*
* Contains :
*
* - Wikitty service as source
*
- wikitties : wikitty added if type contains PUT_WIKITTY
*
- ids & remove date : if type contains REMOVE_WIKITTY
*
- extensions : extension added if type contains PUT_EXTENSION
*
*
* @author chatellier
* @version $Revision$
*
* Last update : $Date$
* By : $Author$
*/
// FIXME poussin 20120823 probleme de securte certain event remonte des wikitties
// que les utilisateurs n'auraient potentiellement pas le droit de lire. Il ne faudrait
// jamais remonter des objets mais seulement des ids. Voir l'impact sur le fonctionne
// global avant de faire quoi que se soit
public class WikittyEvent extends EventObject {
/** serialVersionUID. */
private static final long serialVersionUID = 9017732163643700599L;
/** Message type (put, remove, clear...). */
static public enum WikittyEventType {
PUT_WIKITTY(WikittyListener.PUT_WIKITTY_METHOD),
REMOVE_WIKITTY(WikittyListener.REMOVE_WIKITTY_METHOD),
CLEAR_WIKITTY(WikittyListener.CLEAR_WIKITTY_METHOD),
PUT_EXTENSION(WikittyListener.PUT_EXTENSION_METHOD),
REMOVE_EXTENSION(WikittyListener.REMOVE_EXTENSION_METHOD),
CLEAR_EXTENSION(WikittyListener.CLEAR_EXTENSION_METHOD);
/** le nom de la methode du listener a appeler pour ce type d'event */
public String listenerMethodName;
WikittyEventType(String listenerMethodName) {
this.listenerMethodName = listenerMethodName;
}
}
/** unique event id, each event must have eventId, and event is sequence
* without hole. (ex: 0,1,2,3,4,5,6,...) */
protected long eventId;
/** Remote event (received from server). */
protected boolean remote;
/** event type, one event can have multiple type
* (ex: PUT_WIKITTY + PUT_EXTENSION */
protected EnumSet type;
/** heure de creation de l'event */
protected long time;
/** Use by PUT_WIKITTY, all wikitties added */
protected Map wikitties;
/** Use by REMOVE_WIKITTY. key: wikittyId, value: removed date */
protected Map removeDate;
/** Use by PUT_EXTENSION, all extensions added */
protected Map extensions;
/** Use by REMOVE_EXTENSION, all extensions id deleted */
protected Set deletedExtensions;
/**
* Constructor with source {@link WikittyService}.
*
* @param source wikitty service
*/
public WikittyEvent(Object source) {
super(source);
this.time = System.currentTimeMillis();
this.type = EnumSet.noneOf(WikittyEventType.class);
}
/**
* Return time of event creation.
*
* @return
*/
public long getTime() {
return time;
}
/**
* To allow set transient source after deserialisation.
*
* @param source source
*/
public void setSource(Object source) {
this.source = source;
}
public EnumSet getType() {
return type;
}
/**
* @param type
*/
public void addType(WikittyEventType type) {
this.type.add(type);
}
/**
* Is event remote.
*
* @return remote event
*/
public boolean isRemote() {
return remote;
}
/**
* Change remote event property.
*
* @param remote remote
*/
public void setRemote(boolean remote) {
this.remote = remote;
}
public long getEventId() {
return eventId;
}
/**
* This method must be call with right id, just before send message
* notification
* @param eventId
*/
public void setEventId(long eventId) {
this.eventId = eventId;
}
public Map getWikitties() {
return wikitties;
}
public void addWikitty(Wikitty wikitty) {
if (wikitties == null) {
wikitties = new LinkedHashMap();
addType(WikittyEventType.PUT_WIKITTY);
}
this.wikitties.put(wikitty.getWikittyId(), wikitty);
}
public Map getRemoveDate() {
return removeDate;
}
public void addRemoveDate(String wikittyId, Date date) {
if (removeDate == null) {
removeDate = new HashMap();
addType(WikittyEventType.REMOVE_WIKITTY);
}
removeDate.put(wikittyId, date);
}
public Map getExtensions() {
return extensions;
}
public void addExtension(WikittyExtension extension) {
if (extensions == null) {
extensions = new LinkedHashMap();
addType(WikittyEventType.PUT_EXTENSION);
}
extensions.put(extension.getId(), extension);
}
public Set getDeletedExtensions() {
return deletedExtensions;
}
public void addDeletedExtension(String id) {
if (deletedExtensions == null) {
deletedExtensions = new LinkedHashSet();
addType(WikittyEventType.REMOVE_EXTENSION);
}
deletedExtensions.add(id);
}
/**
* Merge this event with event passed in arguement. Merged datas are:
* type
* wikitties
* extensions
* removeDate
*
* @param e
*/
public void add(WikittyEvent e) {
getType().addAll(e.getType());
if (e.getWikitties() != null) {
for (Map.Entry i : e.getWikitties().entrySet()) {
addWikitty(i.getValue());
}
}
if (e.getExtensions() != null) {
for (Map.Entry i : e.getExtensions().entrySet()) {
addExtension(i.getValue());
}
}
if (e.getRemoveDate() != null) {
for (Map.Entry i : e.getRemoveDate().entrySet()) {
addRemoveDate(i.getKey(), i.getValue());
}
}
}
/**
* Update data directly in object passed in argument.
* Actually only version and deletion date are updated.
*
* rem: during store action, no migration has done. Migration is only
* done during restore process. This implies that extension don't change
* after store. But another client, may can load wikitty with migration
* and store it, or add manually some extension. In that case, stored wikitty
* has new/more extension that another client.
*
* And internally wikitty object is marked clean (not dirty)
* @param e
* @return wikitty passed in argument or null, if event is CLEAR_WIKITTY
*/
public Wikitty update(Wikitty e) {
if (e != null) {
// update version
String id = e.getWikittyId();
if (type.contains(WikittyEventType.CLEAR_WIKITTY)) {
e = null;
} else {
if (type.contains(WikittyEventType.PUT_WIKITTY)) {
Wikitty newWikitty = getWikitties().get(id);
// can be null if wikitty is already saved (uptodate), then this wikitty is not re-saved
if (newWikitty != null) {
e.replaceWith(newWikitty);
}
}
if (type.contains(WikittyEventType.REMOVE_WIKITTY)) {
Date date = getRemoveDate().get(id);
e.setDeleteDate(date);
}
e.clearDirty();
}
}
return e;
}
@Override
public String toString() {
String toString = getClass().getName()
+ "[source=" + source
+ ", eventId=" + eventId
+ ", time=" + time
+ ", type=" + type
+ ", remote=" + remote
+ ", wikitties=" + wikitties
+ ", removeDate=" + removeDate
+ ", extensions=" + extensions
+ "]";
return toString;
}
/**
* Permet de serializer en XML l'event. Pourrait etre utilise pour l'envoi
* sur un transporteur qui ne permet pas la serialisation java
* @return
*/
public String toXML() {
XStream xstream = new XStream();
xstream.setMode(XStream.NO_REFERENCES);
xstream.alias("event", WikittyEvent.class);
String result = xstream.toXML(this);
return result;
}
/**
* Inverse de la methode toXML
* @param xml
* @return
*/
static public WikittyEvent fromXML(String xml) {
XStream xstream = new XStream();
xstream.alias("event", WikittyEvent.class);
WikittyEvent result = (WikittyEvent)xstream.fromXML(xml);
return result;
}
}