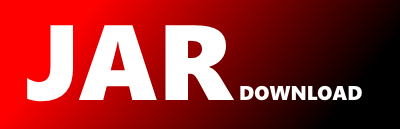
org.nuiton.wikitty.services.WikittySecurityUtil Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2011 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.services;
import java.util.Collections;
import java.util.Date;
import java.util.Set;
import org.nuiton.config.ApplicationConfig;
import org.nuiton.wikitty.WikittyClient;
import org.nuiton.wikitty.WikittyConfigOption;
import org.nuiton.wikitty.WikittyService;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.entities.WikittyGroup;
import org.nuiton.wikitty.entities.WikittyGroupHelper;
import org.nuiton.wikitty.entities.WikittyGroupImpl;
import org.nuiton.wikitty.entities.WikittyTokenHelper;
import org.nuiton.wikitty.entities.WikittyUser;
import org.nuiton.wikitty.query.WikittyQuery;
import org.nuiton.wikitty.query.WikittyQueryMaker;
import org.nuiton.wikitty.query.WikittyQueryResult;
/**
*
* Security utility methods. This class should replace #WikittySecurityHelper
*
* User: couteau
* Date: 22/12/10
*/
public class WikittySecurityUtil {
/**
* Name of the application administrators group
*/
static final public String WIKITTY_APPADMIN_GROUP_NAME = "WikittyAppAdmin";
/**
* Verifie si la date du token depasse le temps authorise pour un token
* Si l'option n'est pas presente dans la config retourne toujours false
* (donc le token a une validite permanente)
*
* @param date la date a verifier
* @return vrai si la date est depassee
*/
public static boolean isOutdated(
ApplicationConfig config, WikittyService ws, String tokenId) {
boolean result = false;
if (config != null) {
// TODO poussin 20120123 prevoir un timeout dans la config
// qui invaliderait le vieux token et en recreerait un nouveau
long timeout = 1000 * config.getOptionAsLong(
WikittyConfigOption.WIKITTY_SERVICE_AUTHENTICATION_TIMEOUT.getKey());
if (timeout > 0) {
Wikitty token = ws.restore(
null, Collections.singletonList(tokenId)).get(0);
Date date = WikittyTokenHelper.getDate(token);
Date now = new Date();
long nowMillis = now.getTime();
long dateMillis = date.getTime();
long delta = nowMillis - dateMillis;
result = delta > timeout;
}
}
return result;
}
/**
* Check on a WikittyService if a user is member of a group. A
* SecurityException might be thrown at runtime if the securityToken has
* expired.
*
* @param ws the wikitty service to do the check on
* @param securityToken the security token
* @param userId the user to check's wikitty id
* @param groupId the group to check the user is in's wikitty id
* @return true if the user is in the group, false otherwise
*/
public static boolean isMember(WikittyService ws, String securityToken,
String userId, String groupId) {
if (groupId != null) {
Wikitty group = WikittyServiceEnhanced.restore(ws, securityToken,
groupId);
if (WikittyGroupHelper.hasExtension(group)) {
Set members = WikittyGroupHelper.getMembers(group);
if (members != null) {
return members.contains(userId);
}
}
}
return false;
}
/**
* Get a user corresponding to a securityToken
* @param ws the WikittyService to do the check on
* @param securityToken the token of the user to search for.
* @return the wikitty Id of the user corresponding to the securityToken
*/
public static String getUserForToken(WikittyService ws,
String securityToken) {
// recuperation de l'utilisateur associe au securityToken
// le securityToken est aussi l'id de l'objet
String userId = null;
if (securityToken != null) {
//Get the token
Wikitty securityTokenWikitty = WikittyServiceEnhanced.restore(ws,
securityToken, securityToken);
if (securityTokenWikitty == null) {
throw new SecurityException("bad (obsolete ?) token");
} else {
//Get the user
userId = WikittyTokenHelper.getUser(securityTokenWikitty);
}
}
return userId;
}
/**
* Create appAdminGroup and add current user as first member. The group has
* to be stored after as it is not persisted in this method.
*
* @param user the user that will be the first admin group member
* @return the admin group
*/
static public WikittyGroup createAppAdminGroup(WikittyUser user) {
WikittyGroup result = new WikittyGroupImpl();
result.setName(WIKITTY_APPADMIN_GROUP_NAME);
String firstUserId = user.getWikittyId();
result.addMembers(firstUserId);
return result;
}
static public WikittyGroup getAppAdminGroup(WikittyClient client) {
WikittyQuery criteria = new WikittyQueryMaker().and()
.exteq(WikittyGroup.EXT_WIKITTYGROUP)
.eq(WikittyGroup.ELEMENT_FIELD_WIKITTYGROUP_NAME, WIKITTY_APPADMIN_GROUP_NAME).end();
WikittyGroup result = client.findByQuery(WikittyGroup.class, criteria);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy