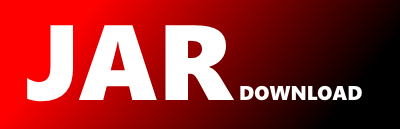
org.nuiton.wikitty.services.WikittyServiceAuthenticationAbstract Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2012 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.services;
import java.util.Arrays;
import java.util.Date;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.config.ApplicationConfig;
import org.nuiton.util.TimeLog;
import org.nuiton.wikitty.WikittyConfigOption;
import org.nuiton.wikitty.WikittyService;
import org.nuiton.wikitty.WikittyUtil;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.entities.WikittyImpl;
import org.nuiton.wikitty.entities.WikittyToken;
import org.nuiton.wikitty.entities.WikittyTokenHelper;
import org.nuiton.wikitty.entities.WikittyUserHelper;
import org.nuiton.wikitty.query.WikittyQuery;
import org.nuiton.wikitty.query.WikittyQueryMaker;
/**
* Classe abstraite pour simplifier l'implantation d'autre methode
* d'authentification. Ce serivce surcharge logout qui supprime le token
* et la methode getToken qui recupere ou genere un token.
*
* Pour implanter une nouveau service, il faut faire l'authentification de la
* personne si elle reussi, on retourne l'id du token retourne par getToken
* si l'authentification echoue il faut lever une exception.
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public abstract class WikittyServiceAuthenticationAbstract extends WikittyServiceDelegator {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(WikittyServiceAuthenticationAbstract.class);
/** use to trace time of security code, timelog must not include delegator
* time in this class */
final static private TimeLog timeLog = new TimeLog(WikittyServiceAuthenticationAbstract.class);
protected ApplicationConfig config;
public WikittyServiceAuthenticationAbstract(WikittyService delegate) {
this(null, delegate);
}
public WikittyServiceAuthenticationAbstract(
ApplicationConfig config, WikittyService delegate) {
super(delegate);
this.config = config;
if (config != null) {
long timeToLogInfo = config.getOptionAsInt(WikittyConfigOption.
WIKITTY_SERVICE_TIME_TO_LOG_INFO.getKey());
long timeToLogWarn = config.getOptionAsInt(WikittyConfigOption.
WIKITTY_SERVICE_TIME_TO_LOG_WARN.getKey());
timeLog.setTimeToLogInfo(timeToLogInfo);
timeLog.setTimeToLogWarn(timeToLogWarn);
}
}
@Override
abstract public String login(String login, String password);
/**
* Recherche si l'utilisateur n'a pas deja un token, et dans ce cas on
* retourne le meme token. Sinon on en cree un nouveau
*
* @param userId l'utilisateur pour l'equel il faut recherche/creer le token
* @return le token de l'utilisateur
*/
@Override
public String getToken(String userId) {
// on recherche si l'utilisateur n'est pas deja authentifier.
// s'il l'est on lui retourne le meme token.
WikittyQuery query = new WikittyQueryMaker()
.eq(WikittyToken.ELEMENT_FIELD_WIKITTYTOKEN_USER, userId)
.end()
.setLimit(1);
String tokenId = getAnonymousClient().findByQuery(query);
// on a retrouve un ancien token, on le reutilise peut-etre
if (tokenId != null) {
if (WikittySecurityUtil.isOutdated(config, getDelegate(), tokenId)) {
getDelegate().delete(tokenId, Arrays.asList(tokenId));
tokenId = null;
}
}
if (tokenId == null) {
// generation d'un nouveau token
tokenId = WikittyUtil.genSecurityTokenId();
Wikitty wikittyToken = new WikittyImpl(tokenId);
// force add extension to wikitty
WikittyTokenHelper.addExtension(wikittyToken);
WikittyTokenHelper.setUser(wikittyToken, userId);
WikittyTokenHelper.setDate(wikittyToken, new Date());
getDelegate().store(null, Arrays.asList(wikittyToken), false);
if (log.isDebugEnabled()) {
log.debug(String.format("token '%s' is for login '%s'",
tokenId, userId));
}
}
return tokenId;
}
@Override
public void logout(String securityToken) {
long start = TimeLog.getTime();
if (securityToken != null) {
getDelegate().delete(securityToken, Arrays.asList(securityToken));
}
timeLog.log(start, "logout");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy