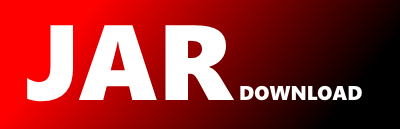
org.nuiton.wikitty.services.WikittyServiceInMemory Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.services;
import org.nuiton.wikitty.storage.WikittySearchEngineInMemory;
import org.nuiton.wikitty.storage.WikittyExtensionStorageInMemory;
import org.nuiton.wikitty.storage.WikittyStorageInMemory;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.config.ApplicationConfig;
import org.nuiton.wikitty.WikittyConfig;
import org.nuiton.wikitty.WikittyConfigOption;
/**
* WARNING In memory implementation of WikittyService, currently used for test only.
*
* If you want in memory wikitty, you must use h2 and solr int inmemory mode
* (show configuration sample)
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public class WikittyServiceInMemory extends WikittyServiceStorage {
//TODO InMemory implementation is not usable for production. Must be reviewed.
//TODO The version increment must be done in 'prepare' method
/** to use log facility, just put in your code: log.info(\"...\"); */
static private Log log = LogFactory.getLog(WikittyServiceInMemory.class);
/**
* if persistenceFile is not null, serialize all data to disk during store
* operation and the file is reloaded during init
*/
protected File persistenceFile = null;
public WikittyServiceInMemory(ApplicationConfig config) {
super(config,
new WikittyExtensionStorageInMemory(),
new WikittyStorageInMemory(),
null);
searchEngine = new WikittySearchEngineInMemory(
(WikittyStorageInMemory) wikittyStorage);
if (config != null) {
boolean persist = config.getOptionAsBoolean(
WikittyConfigOption.WIKITTY_WIKITTYSERVICEINMEMORY_PERSISTENCE.getKey());
if (persist) {
persistenceFile = config.getOptionAsFile(
WikittyConfigOption.WIKITTY_WIKITTYSERVICEINMEMORY_PERSISTENCE_FILE.getKey());
restoreFromPersistenceFile(persistenceFile);
}
}
}
@Override
protected void finalize() throws Throwable {
saveToPersistenceFile(persistenceFile);
super.finalize();
}
protected void restoreFromPersistenceFile(File persistenceFile) {
if (persistenceFile != null && persistenceFile.exists()) {
try {
ObjectInputStream in = new ObjectInputStream(new FileInputStream(
persistenceFile));
((WikittyExtensionStorageInMemory)extensionStorage).setExtensions((Map) in.readObject());
((WikittyStorageInMemory)wikittyStorage).setWikitties((Map) in.readObject());
in.close();
} catch (Exception eee) {
log.error("Can't read data file " + persistenceFile, eee);
}
}
}
public void saveToPersistenceFile(File persistenceFile) {
if (persistenceFile != null) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(
persistenceFile));
out.writeObject(((WikittyExtensionStorageInMemory)extensionStorage).getExtensions());
out.writeObject(((WikittyStorageInMemory)wikittyStorage).getWikitties());
out.close();
} catch (IOException eee) {
log.error("Can't write data file " + persistenceFile, eee);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy