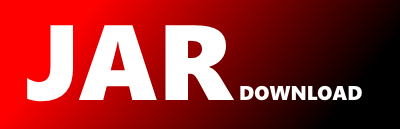
org.nuiton.wikitty.storage.WikittySearchEngine Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.storage;
import java.io.Serializable;
import java.util.Collection;
import java.util.Map;
import org.nuiton.wikitty.search.Criteria;
import org.nuiton.wikitty.search.PagedResult;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.WikittyException;
import org.nuiton.wikitty.query.WikittyQuery;
import org.nuiton.wikitty.query.WikittyQueryResult;
import org.nuiton.wikitty.query.WikittyQueryResultTreeNode;
import org.nuiton.wikitty.search.TreeNodeResult;
import org.nuiton.wikitty.services.WikittyTransaction;
/**
* WikittySearchEngine is used to abstract search engine used in WikittyService.
*
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public interface WikittySearchEngine {
/**
* Remove all data in index
*/
public void clear(WikittyTransaction transaction);
/**
* Store wikitty in storage
* Tree are reindexed if necessary.
* @param force if true, force indexation of wikitty, otherwize only dirty
* or new wikitties are indexed
*/
public void store(WikittyTransaction transaction,
Collection wikitties, boolean force);
/**
* Delete all object with idList argument. If id is not valid or don't exist.
* Tree are reindexed if necessary.
*
* @param idList list of ids to delete
* @throws WikittyException
*/
public void delete(WikittyTransaction transaction,
Collection idList) throws WikittyException;
/**
* @deprecated since 3.3 use {@link #findAllByCriteria(org.nuiton.wikitty.services.WikittyTransaction, org.nuiton.wikitty.search.Criteria)}
*/
@Deprecated
public PagedResult findAllByCriteria(WikittyTransaction transaction, Criteria criteria);
/**
* Find all values that satisfy queries constraint. Values is Wikitty's id
* if there is no Select condition, otherwize is String that represent
* field value and can be String representation of
* Wikitty, Date, Boolean, Numeric, Binary, String.
* Or TODO (map? array?)
*
* @param transaction
* @param queries
* @return id of wikitties
* @since 3.3
*/
public WikittyQueryResult
© 2015 - 2025 Weber Informatics LLC | Privacy Policy