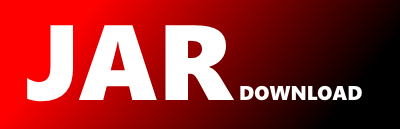
org.nuiton.wikitty.storage.WikittyStorage Maven / Gradle / Ivy
The newest version!
/*
* #%L
* Wikitty :: api
* %%
* Copyright (C) 2009 - 2010 CodeLutin, Benjamin Poussin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.wikitty.storage;
import java.util.Collection;
import org.nuiton.wikitty.entities.Wikitty;
import org.nuiton.wikitty.WikittyException;
import org.nuiton.wikitty.services.WikittyEvent;
import org.nuiton.wikitty.services.WikittyTransaction;
/**
* WikittyStorage is used to abstract data and extension storage in WikittyService.
*
* @author poussin
* @version $Revision$
*
* Last update: $Date$
* by : $Author$
*/
public interface WikittyStorage {
/**
* Store wikitty in storage.
*
* if force is true, wikitty in argument is all time stored.
* if wikitty already stored and deleted, wikitty is born again
*
* new version is computed as follow:
*
* if version in wikitty > version in database
* newversion = version in wikitty
* else
* newversion = version in database + 1
*
*
* @param transaction transaction
* @param wikitties wikitty collection
* @param force boolean force non version version increment on saved wikitty
* or force version on wikitty creation (version 0.0)
*
* @return information usefull for client side update data
*/
public WikittyEvent store(WikittyTransaction transaction, Collection wikitties, boolean force);
/**
* Return true if id exists in storage.
*
* @param transaction transaction
* @param id id to check
* @return {@code true} if wikitty id exists
*/
public boolean exists(WikittyTransaction transaction, String id);
/**
* Return true if wikitty object with this id are marked deleted.
*
* @param transaction transaction
* @param id id to check
* @return {@code true} if wikitty id is deleted
*/
public boolean isDeleted(WikittyTransaction transaction, String id);
/**
* Restore list of wikitty object.
*
* @param transaction transaction
* @param id list of wikitty id
* @param fqFieldName list of field that must be loaded, other fields
* can be not loaded
* @return found wikitty
* @throws WikittyException if wikitty not found
*/
public Wikitty restore(WikittyTransaction transaction, String id,
String ... fqFieldName) throws WikittyException;
/**
* Delete all object with id argument. Delete don't fail if wikitty
* don't existe or is already deleted.
*
* @param transaction transaction
* @param idList
* @return delete response (can be empty if no wikitty are realy deleted
* @throws WikittyException If id is not valid or don't exist.
*/
public WikittyEvent delete(WikittyTransaction transaction, Collection idList) throws WikittyException;
/**
* Scan all wikitties with specific scanner, even if the wikitty is deleted.
*
* @param transaction transaction
* @param scanner scanner
*/
public void scanWikitties(WikittyTransaction transaction, Scanner scanner);
/**
* Call each time wikitty is scan.
*/
public static interface Scanner {
void scan(String wikittyId);
}
/**
* Remove all wikitty.
*
* @param transaction transaction
*/
public WikittyEvent clear(WikittyTransaction transaction);
/**
* Return some statistique about the data
* @param transaction
* @return
* @since 3.0.5
*/
public DataStatistic getDataStatistic(WikittyTransaction transaction);
/**
* @see #getDataStatistic(org.nuiton.wikitty.services.WikittyTransaction)
* @since 3.0.5
*/
static public class DataStatistic {
protected long activeWikitties = -1;
protected long deletedWikitties = -1;
/**
* Use this constructor when your implementation don't support statistic
*/
public DataStatistic() {
}
public DataStatistic(long activeWikitties, long deletedWikitties) {
this.activeWikitties = activeWikitties;
this.deletedWikitties = deletedWikitties;
}
/**
* Return number of non deleted wikitties, or negative value if this
* information can't be retrieved
* @return
*/
public long getActiveWikitties() {
return activeWikitties;
}
/**
* Return number of deleted wikitties, or negative value if this
* information can't be retrieved
* @return
*/
public long getDeletedWikitties() {
return deletedWikitties;
}
/**
* Return number of non deleted and deleted wikitties, or negative value
* if this information can't be retrieved
* @return
*/
public long getTotalWikitties() {
long result = getActiveWikitties() + getDeletedWikitties();
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy