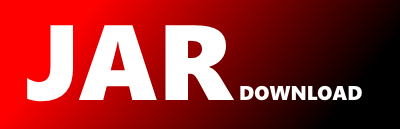
org.nuiton.wikitty.entities.WikittyLabelDTO Maven / Gradle / Ivy
package org.nuiton.wikitty.entities;
/*
* #%L
* Wikitty :: dto
* %%
* Copyright (C) 2010 - 2015 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.beans.PropertyChangeListener;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.Set;
import javax.annotation.Generated;
import org.nuiton.wikitty.WikittyException;
@Generated(value = "org.nuiton.wikitty.generator.WikittyDTOGenerator", date = "Thu Aug 06 22:58:52 CEST 2015")
public class WikittyLabelDTO implements WikittyLabel {
@WikittyField(fqn = "WikittyLabel.labels")
protected Set labels = new LinkedHashSet();
protected String wikittyId;
protected int modificationCount = 0;
protected String wikittyVersion;
@Override
public Set getLabels() {
return labels;
}
@Override
public void setLabels(Set labels) {
if (labels == null){
this.labels = new LinkedHashSet();
} else {
// make copy to prevent modification of source collection
this.labels=new LinkedHashSet(labels);
}
modificationCount++;
}
@Override
public void addAllLabels(Collection labels) {
if (this.labels == null){
this.labels = new LinkedHashSet();
}
this.labels.addAll(labels);
modificationCount++;
}
@Override
public void addLabels(String... element) {
if (this.labels == null){
this.labels = new LinkedHashSet();
}
for (String v : element) {
this.labels.add(v);
}
modificationCount++;
}
@Override
public void removeLabels(String... element) {
if (this.labels != null) {
for (String v : element) {
labels.remove(element);
}
modificationCount++;
}
}
@Override
public void clearLabels() {
if (this.labels != null) {
labels.clear();
modificationCount++;
}
}
public WikittyLabelDTO() {
}
public WikittyLabelDTO(String wikittyId) {
this.wikittyId=wikittyId;
}
@Override
public String toString() {
return "dto:"+getWikittyId()+":"+getWikittyVersion();
}
@Override
public String getWikittyId() {
return wikittyId;
}
public void setWikittyId(String wikittyId) {
this.wikittyId=wikittyId;
}
@Override
public String getWikittyVersion() {
String result = wikittyVersion;
if (modificationCount > 0) {
result += "." + modificationCount;
}
return result;
}
public void setWikittyVersion(String wikittyVersion) {
this.wikittyVersion=wikittyVersion;
modificationCount=0;
}
@Override
public void addPropertyChangeListener(PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void removePropertyChangeListener(PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void addPropertyChangeListener(String property, PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void removePropertyChangeListener(String property, PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection getExtensionFields(String ext) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection getExtensionNames() {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection getStaticExtensionNames() {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Object getField(String ext, String fieldName) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Object getFieldAsObject(String ext, String fieldName) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void setField(String ext, String fieldName, Object value) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void copyFrom(BusinessEntity source) {
if (!(source instanceof WikittyLabel)){
throw new WikittyException("Can't copy source object " + source +
". They are not of the same type");
}
WikittyLabel sourceCopy = (WikittyLabel)source;
Set labels = sourceCopy.getLabels();
if (labels != null){
setLabels(new LinkedHashSet(labels));
}
setWikittyVersion(sourceCopy.getWikittyVersion());
}
} //WikittyLabelDTO
© 2015 - 2025 Weber Informatics LLC | Privacy Policy