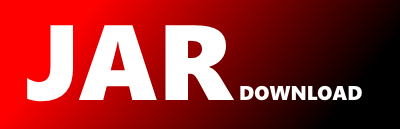
org.nuiton.wikitty.entities.WikittyTreeNodeDTO Maven / Gradle / Ivy
package org.nuiton.wikitty.entities;
/*
* #%L
* Wikitty :: dto
* %%
* Copyright (C) 2010 - 2015 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.beans.PropertyChangeListener;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.Set;
import javax.annotation.Generated;
import org.nuiton.wikitty.WikittyException;
@Generated(value = "org.nuiton.wikitty.generator.WikittyDTOGenerator", date = "Thu Aug 06 22:58:52 CEST 2015")
public class WikittyTreeNodeDTO implements WikittyTreeNode {
@WikittyField(fqn = "WikittyTreeNode.name")
protected String name;
@WikittyField(fqn = "WikittyTreeNode.attachment")
protected Set attachment = new LinkedHashSet();
@WikittyField(fqn = "WikittyTreeNode.parent")
protected String parent;
protected String wikittyId;
protected int modificationCount = 0;
protected String wikittyVersion;
@Override
public String getName() {
return name;
}
@Override
public void setName(String name) {
this.name=name;
modificationCount++;
}
@Override
public Set getAttachment() {
return attachment;
}
@Override
public void setAttachment(Set attachment) {
if (attachment == null){
this.attachment = new LinkedHashSet();
} else {
// make copy to prevent modification of source collection
this.attachment=new LinkedHashSet(attachment);
}
modificationCount++;
}
@Override
public void addAllAttachment(Collection attachment) {
if (this.attachment == null){
this.attachment = new LinkedHashSet();
}
this.attachment.addAll(attachment);
modificationCount++;
}
@Override
public void addAttachment(String... element) {
if (this.attachment == null){
this.attachment = new LinkedHashSet();
}
for (String v : element) {
this.attachment.add(v);
}
modificationCount++;
}
@Override
public void removeAttachment(String... element) {
if (this.attachment != null) {
for (String v : element) {
attachment.remove(element);
}
modificationCount++;
}
}
@Override
public void clearAttachment() {
if (this.attachment != null) {
attachment.clear();
modificationCount++;
}
}
@Override
public Set getAttachment(boolean exceptionIfNotLoaded) {
if (exceptionIfNotLoaded) {
throw new WikittyException("Preload is not implemented in DTO");
} else {
return null;
}
}
@Override
public void setAttachmentEntity(Collection attachment) {
LinkedHashSet tmp = new LinkedHashSet();
for (Wikitty e : attachment) {
tmp.add(e.getWikittyId());
}
setAttachment(tmp);
}
@Override
public void addAllAttachmentEntity(Collection attachment) {
LinkedHashSet tmp = new LinkedHashSet();
for (Wikitty e : attachment) {
tmp.add(e.getWikittyId());
}
addAllAttachment(tmp);
}
@Override
public void addAttachment(Wikitty... element) {
String[] tmp = new String[element.length];
for (int i=0; i 0) {
result += "." + modificationCount;
}
return result;
}
public void setWikittyVersion(String wikittyVersion) {
this.wikittyVersion=wikittyVersion;
modificationCount=0;
}
@Override
public void addPropertyChangeListener(PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void removePropertyChangeListener(PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void addPropertyChangeListener(String property, PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void removePropertyChangeListener(String property, PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection getExtensionFields(String ext) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection getExtensionNames() {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection getStaticExtensionNames() {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Object getField(String ext, String fieldName) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Object getFieldAsObject(String ext, String fieldName) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void setField(String ext, String fieldName, Object value) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void copyFrom(BusinessEntity source) {
if (!(source instanceof WikittyTreeNode)){
throw new WikittyException("Can't copy source object " + source +
". They are not of the same type");
}
WikittyTreeNode sourceCopy = (WikittyTreeNode)source;
setName(sourceCopy.getName());
Set attachment = sourceCopy.getAttachment();
if (attachment != null){
setAttachment(new LinkedHashSet(attachment));
}
setParent(sourceCopy.getParent());
setWikittyVersion(sourceCopy.getWikittyVersion());
}
} //WikittyTreeNodeDTO
© 2015 - 2025 Weber Informatics LLC | Privacy Policy