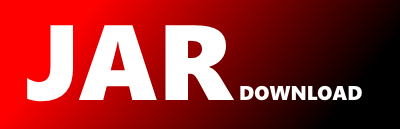
org.nuiton.io.MirroredFileUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helper-maven-plugin-api Show documentation
Show all versions of helper-maven-plugin-api Show documentation
Simple maven mojo api for our projects
package org.nuiton.io;
/*
* #%L
* Helper Maven Plugin :: API
* $Id: MirroredFileUpdater.java 876 2012-11-11 08:14:19Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-2.1/helper-maven-plugin-api/src/main/java/org/nuiton/io/MirroredFileUpdater.java $
* %%
* Copyright (C) 2009 - 2012 Codelutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.io.File;
/**
* Simple base implementation of a {@link FileUpdater} for an updater with
* a sourcedir and a destinationdir.
*
* @author tchemit
*/
public class MirroredFileUpdater implements FileUpdater {
/** to use log facility, just put in your code: log.info(\"...\"); */
static private final Log log = LogFactory.getLog(MirroredFileUpdater.class);
/** source basedir */
protected File sourceDirectory;
/** length of source basedir absolute path */
protected int prefixSourceDirecotory;
/** destination basedir */
protected File destinationDirectory;
protected String fileInPattern = "";
protected String fileOutPattern = "";
public MirroredFileUpdater(String fileInPattern,
String fileOutPattern,
File sourceDirectory,
File destinationDirectory) {
this.fileInPattern = fileInPattern;
this.fileOutPattern = fileOutPattern;
this.sourceDirectory = sourceDirectory;
this.destinationDirectory = destinationDirectory;
if (sourceDirectory != null) {
prefixSourceDirecotory = sourceDirectory.getAbsolutePath().length();
}
if (log.isDebugEnabled()) {
log.debug(this);
}
}
public File getSourceDirectory() {
return sourceDirectory;
}
public File getDestinationDirectory() {
return destinationDirectory;
}
public String getFileInPattern() {
return fileInPattern;
}
public String getFileOutPattern() {
return fileOutPattern;
}
public File getMirrorFile(File f) {
String filename = f.getName();
String file = f.getAbsolutePath().substring(prefixSourceDirecotory);
file = file.substring(0, file.length() - filename.length());
String destFilename = applyTransformationFilename(filename);
String mirrorRelativePath = file + destFilename;
return new File(destinationDirectory + File.separator +
mirrorRelativePath);
}
@Override
public boolean isFileUpToDate(File f) {
File mirror = getMirrorFile(f);
return mirror.exists() && f.lastModified() < mirror.lastModified();
}
public void setDestinationDirectory(File destinationDirectory) {
this.destinationDirectory = destinationDirectory;
}
public void setSourceDirectory(File sourceDirectory) {
this.sourceDirectory = sourceDirectory;
prefixSourceDirecotory = sourceDirectory.getAbsolutePath().length();
}
public void setFileInPattern(String fileInPattern) {
this.fileInPattern = fileInPattern;
}
public void setFileOutPattern(String fileOutPattern) {
this.fileOutPattern = fileOutPattern;
}
public String applyTransformationFilename(String filename) {
return filename.replaceAll(fileInPattern, fileOutPattern);
}
@Override
public String toString() {
return super.toString() + "";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy